파일이 C++에 있는지 확인하는 방법
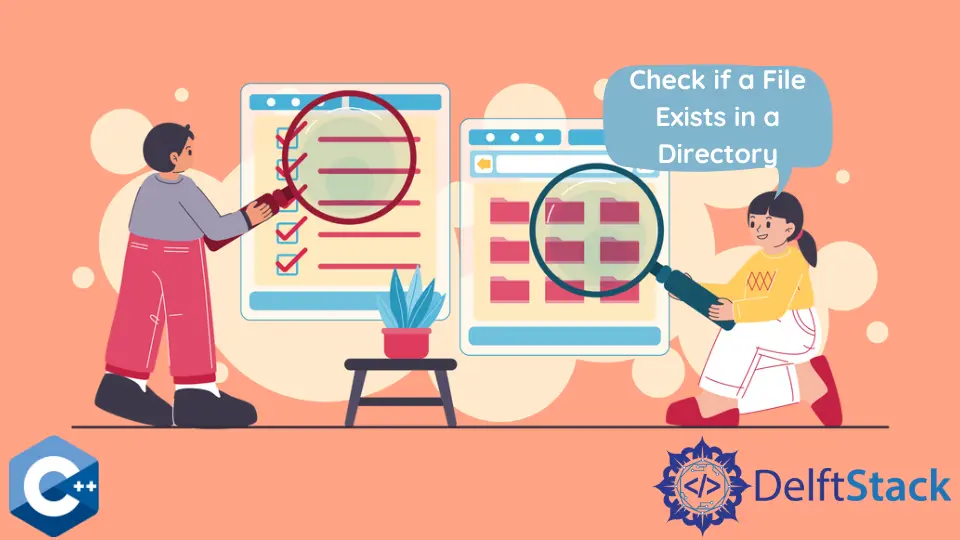
이 기사에서는 특정 파일이 디렉토리에 있는지 확인하는 C++ 메서드를 소개합니다. 하지만 다음 튜토리얼은 새로운 컴파일러에서만 지원되는 C++ 17 filesystem
라이브러리를 기반으로합니다.
std::filesystem::exists
를 사용하여 파일이 디렉토리에 있는지 확인
exists
메소드는 경로를 인수로 취하고 기존 파일 또는 디렉토리에 해당하는 경우 부울 값true
를 리턴합니다. 다음 예제에서는 exists
함수를 사용하여 파일 시스템에서 확인하기 위해 임의의 파일 이름으로 벡터를 초기화합니다. exists
메소드는 실행 파일이있는 현재 디렉토리 만 확인합니다.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::exists;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
for (const auto &file : files_to_check) {
exists(file) ? cout << "Exists\n" : cout << "Doesn't exist\n";
}
return EXIT_SUCCESS;
}
위의 코드는 더 나은 코드 재사용 성을 제공하는for_Each
STL 알고리즘으로 다시 구현할 수 있습니다.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::exists;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
auto check = [](const auto &file) {
exists(file) ? cout << "Exists\n" : cout << "Doesn't exist\n";
};
for_each(files_to_check.begin(), files_to_check.end(), check);
return EXIT_SUCCESS;
}
exists
메소드는 is_directory
및 is_regular_file
과 같은 다른<filesystem>
라이브러리 루틴과 결합하면 더 많은 정보를 얻을 수 있습니다. 일반적으로 일부 파일 시스템 메서드는 파일과 디렉터리를 구분하지 않지만 다음 샘플 코드와 같이 특정 파일 형식 검사 기능을 사용하여 경로 이름을 확인할 수 있습니다.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::exists;
using std::filesystem::is_directory;
using std::filesystem::is_regular_file;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
for (const auto &file : files_to_check) {
if (exists(file)) {
if (is_directory(file)) cout << "Directory exists\n";
if (is_regular_file(file))
cout << "File exists\n";
else
cout << "Exists\n";
} else {
cout << "Doesn't exist\n";
};
};
return EXIT_SUCCESS;
}
이제 특정 디렉터리로 이동하여 특정 파일 이름이 있는지 확인하려는 경우를 고려해 보겠습니다. 이를 위해서는 인자가 전달되지 않으면 현재 디렉토리를 반환하는current_path
메소드를 사용하거나 인자가 주어지면 현재 작업 디렉토리를 지정된 경로로 변경할 수 있습니다. 프로그램 출력을 더 잘 확인하려면 시스템에 따라 디렉토리 경로와 파일 이름을 변경하는 것을 잊지 마십시오.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::current_path;
using std::filesystem::exists;
using std::filesystem::is_directory;
using std::filesystem::is_regular_file;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
current_path("../");
for (const auto &file : files_to_check) {
exists(file) ? cout << "Exists\n" : cout << "Doesn't exist\n";
}
return EXIT_SUCCESS;
}
또한directory_iterator
를 반복하는 동안 현재 사용자가 디렉토리의 파일에 대해 어떤 권한을 가지고 있는지 확인할 수 있습니다. status
메소드는 권한을 가져와perms
라는 특수 클래스에 저장하는 데 사용됩니다. 검색된 권한은perms
구조에 대한 비트 연산으로 표시 될 수 있습니다. 다음 예제는 소유자 권한 만 추출한 것입니다 (여기에서 전체 목록 참조).
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
using std::filesystem::directory_iterator;
using std::filesystem::exists;
using std::filesystem::perms;
using std::string;
int main() {
vector<std::filesystem::perms> f_perm;
string path = "../";
for (const auto& file : directory_iterator(path)) {
cout << file << " - ";
cout << ((file.status().permissions() & perms::owner_read) != perms::none
? "r"
: "-")
<< ((file.status().permissions() & perms::owner_write) != perms::none
? "w"
: "-")
<< ((file.status().permissions() & perms::owner_exec) != perms::none
? "x"
: "-")
<< endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook