C++에서 문자열이 회문인지 확인
-
rbegin
/rend
메서드와 함께string
복사 생성자를 사용하여 C++에서 문자열 회문을 확인합니다 -
std::equal
메서드를 사용하여 C++에서 문자열 회문을 확인합니다 - 사용자 지정 함수를 사용하여 C++에서 문자열 회문 확인
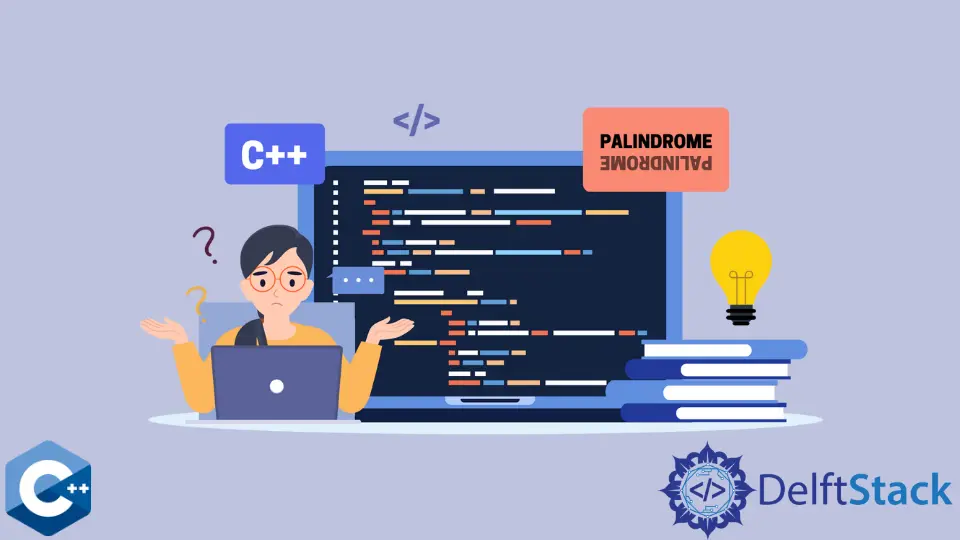
이 기사에서는 C++에서 문자열이 회문인지 확인하는 방법에 대해 설명합니다.
rbegin
/rend
메서드와 함께string
복사 생성자를 사용하여 C++에서 문자열 회문을 확인합니다
string
클래스 객체는==
연산자를 사용한 비교를 지원하며 회문 패턴을 따르는 문자열을 찾는 데 활용할 수 있습니다. 회문은 문자를 역순으로 일치시키는 것을 의미하므로rbegin
및rend
반복자를 사용하여 새 문자열 객체를 생성해야합니다. 나머지는 필요에 따라if
문에서 생성되도록 남겨집니다.
다음 예에서는 한 단어 회문과 여러 단어 회문이라는 두 개의 문자열을 선언합니다. 이 메서드는 정의 패턴에 맞더라도 회문으로 공백이있는 문자열을 감지 할 수 없습니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::remove;
using std::string;
int main() {
string s1 = "radar";
string s2 = "Was it a cat I saw";
if (s1 == string(s1.rbegin(), s1.rend())) {
cout << "s1 is a palindrome" << endl;
} else {
cout << "s1 is not a palindrome" << endl;
}
if (s2 == string(s2.rbegin(), s2.rend())) {
cout << "s2 is a palindrome" << endl;
} else {
cout << "s2 is not a palindrome" << endl;
}
return EXIT_SUCCESS;
}
출력:
s1 is a palindrome
s2 is not a palindrome
std::equal
메서드를 사용하여 C++에서 문자열 회문을 확인합니다
마지막 구현이 한 단어 문자열에 대해 작업을 수행하더라도 개체 복사본을 만들고 전체 범위를 비교하는 오버 헤드가 있습니다. std::equal
알고리즘을 사용하여 동일한 ‘문자열’개체 범위의 전반부와 후반부를 비교할 수 있습니다. std::equal
은 지정된 두 범위의 요소가 같으면 부울 값 true
를 반환합니다. 범위의 끝이 first2 + (last1 - first1)
로 계산되기 때문에 함수는 두 번째 범위에 대해 하나의 반복자 인s1.rbegin()
만 사용합니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::remove;
using std::string;
int main() {
string s1 = "radar";
string s2 = "Was it a cat I saw";
equal(s1.begin(), s1.begin() + s1.size() / 2, s1.rbegin())
? cout << "s1 is a palindrome" << endl
: cout << "s1 is not a palindrome" << endl;
equal(s2.begin(), s2.begin() + s2.size() / 2, s2.rbegin())
? cout << "s2 is a palindrome" << endl
: cout << "s2 is not a palindrome" << endl;
return EXIT_SUCCESS;
}
출력:
s1 is a palindrome
s2 is not a palindrome
사용자 지정 함수를 사용하여 C++에서 문자열 회문 확인
이전 메서드는 여러 단어가있는 문자열에서 부족하므로 사용자 지정 함수를 구현하여 해결할 수 있습니다. 이 예는string&
인수를 취하고 값을 로컬string
변수에 저장하는 부울 함수checkPalindrome
을 보여줍니다. 그런 다음 로컬 객체는transform
알고리즘으로 처리되어이를 소문자로 변환하고 결과적으로erase-remove
관용구에 의해 모든 공백 문자를 삭제합니다. 마지막으로 if
문 조건에서 equal
알고리즘을 호출하고 해당하는 부울 값을 반환합니다. 하지만 문자열이 멀티 바이트 문자로 구성된 경우이 메서드는 실패합니다. 따라서 모든 공통 문자 인코딩 체계를 지원하는 소문자 변환 방법을 구현해야합니다.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::remove;
using std::string;
bool checkPalindrome(string& s) {
string tmp = s;
transform(tmp.begin(), tmp.end(), tmp.begin(),
[](unsigned char c) { return tolower(c); });
tmp.erase(remove(tmp.begin(), tmp.end(), ' '), tmp.end());
if (equal(tmp.begin(), tmp.begin() + tmp.size() / 2, tmp.rbegin())) {
return true;
} else {
return false;
}
}
int main() {
string s1 = "radar";
string s2 = "Was it a cat I saw";
checkPalindrome(s1) ? cout << "s1 is a palindrome" << endl
: cout << "s1 is not a palindrome" << endl;
checkPalindrome(s2) ? cout << "s2 is a palindrome" << endl
: cout << "s2 is not a palindrome" << endl;
return EXIT_SUCCESS;
}
출력:
s1 is a palindrome
s2 is a palindrome
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook