C++의 맵에 키가 있는지 확인
-
std::map::find
함수를 사용하여 C++ 맵에 키가 있는지 확인 -
std::map::count
함수를 사용하여 C++ 맵에 키가 있는지 확인 -
std::map::contains
함수를 사용하여 C++ 맵에 키가 있는지 확인
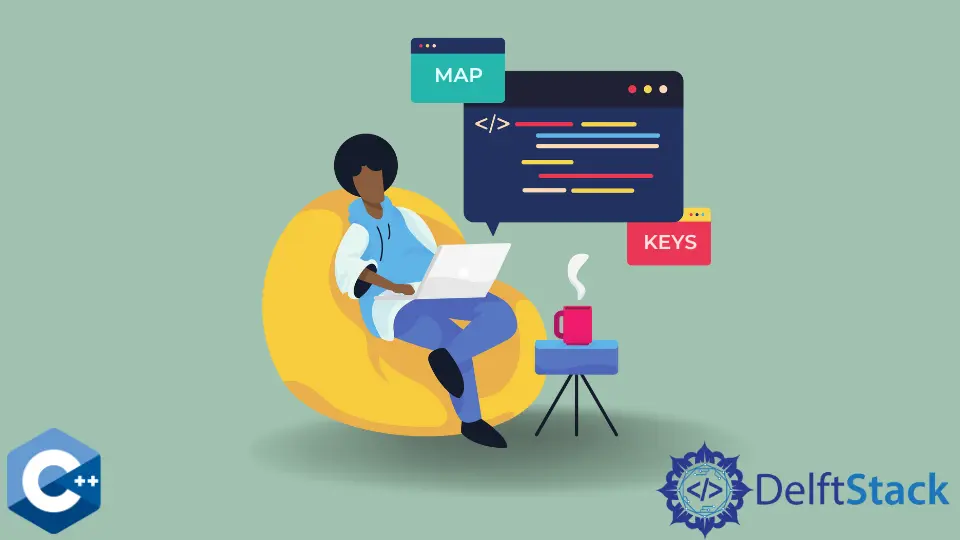
이 기사에서는 C++의 맵에 키가 있는지 확인하는 방법을 소개합니다.
std::map::find
함수를 사용하여 C++ 맵에 키가 있는지 확인
std::map
컨테이너는 정렬 된 키-값 쌍의 연관 데이터 구조이며 각 요소에는 고유 한 키가 있습니다. 반면에 STL은std::unordered_map
이라는 동일한 컨테이너의 정렬되지 않은 버전도 제공합니다. 두 컨테이너 모두 아래에 설명 된 키 검색 방법을 지원합니다.
find
는 검색 할 해당 키 값의 단일 인수를 취하는std::map
컨테이너의 내장 함수 중 하나입니다. 이 함수는 주어진 키-값을 가진 요소에 대한 반복자를 반환합니다. 그렇지 않으면 마지막 반복자를 반환합니다. 다음 예제에서는std::pair<string, string>
유형의map
을 초기화 한 다음find
함수에 전달 된 사용자 입력에서 키 값을 가져옵니다. 예제 프로그램은cout
스트림에 긍정 문자열을 출력합니다.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
string key_to_find;
std::map<string, string> lang_map = {{
"j",
"Julia",
},
{
"p",
"Python",
},
{
"m",
"MATLAB",
},
{
"o",
"Octave",
},
{
"s",
"Scala",
},
{
"l",
"Lua",
}};
for (const auto& [key, value] : lang_map) {
cout << key << " : " << value << endl;
}
cout << "Enter the key to search for: ";
cin >> key_to_find;
if (lang_map.find(key_to_find) != lang_map.end()) {
cout << "Key Exists!" << endl;
}
return EXIT_SUCCESS;
}
출력:
j : Julia
l : Lua
m : MATLAB
o : Octave
p : Python
s : Scala
Enter the key to search for: l
Key Exists!
std::map::count
함수를 사용하여 C++ 맵에 키가 있는지 확인
또는std::map
컨테이너의count
내장 함수를 사용하여 지정된 키가 맵 객체에 존재하는지 확인할 수 있습니다. count
함수는 주어진 키 값을 가진 요소의 수를 검색합니다. 키0
값을 가진 요소가 없으면 값이 리턴됩니다. 따라서count
함수 호출을if
조건으로 사용하여 지정된 키가 맵 객체에 존재할 때 확인 문자열을 출력 할 수 있습니다.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
string key_to_find;
std::map<string, string> lang_map = {{
"j",
"Julia",
},
{
"p",
"Python",
},
{
"m",
"MATLAB",
},
{
"o",
"Octave",
},
{
"s",
"Scala",
},
{
"l",
"Lua",
}};
cout << "Enter the key to search for: ";
cin >> key_to_find;
if (lang_map.count(key_to_find)) {
cout << "Key Exists!" << endl;
}
return EXIT_SUCCESS;
}
출력:
Enter the key to search for: l
Key Exists!
std::map::contains
함수를 사용하여 C++ 맵에 키가 있는지 확인
contains
는 키가map
에 있는지 확인하는 데 사용할 수있는 또 다른 내장 함수입니다. 이 함수는 주어진 키를 가진 요소가 객체에 존재하는 경우 부울 값을 반환합니다. 이 기사에 나열된 세 가지 함수는 모두 로그 복잡성을가집니다.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
string key_to_find;
std::map<string, string> lang_map = {{
"j",
"Julia",
},
{
"p",
"Python",
},
{
"m",
"MATLAB",
},
{
"o",
"Octave",
},
{
"s",
"Scala",
},
{
"l",
"Lua",
}};
cout << "Enter the key to search for: ";
cin >> key_to_find;
if (lang_map.contains(key_to_find)) {
cout << "Key Exists!" << endl;
}
return EXIT_SUCCESS;
}
출력:
Enter the key to search for: l
Key Exists!
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook