C++ 벡터에 요소가 있는지 확인하는 방법
-
벡터에 요소가 있는지 확인하는 C++
std::find()
알고리즘 -
벡터에 요소가 있는지 확인하는 C++ 범위 기반
for
루프 -
벡터에 요소가 있는지 확인하는 C++
any_of()
알고리즘
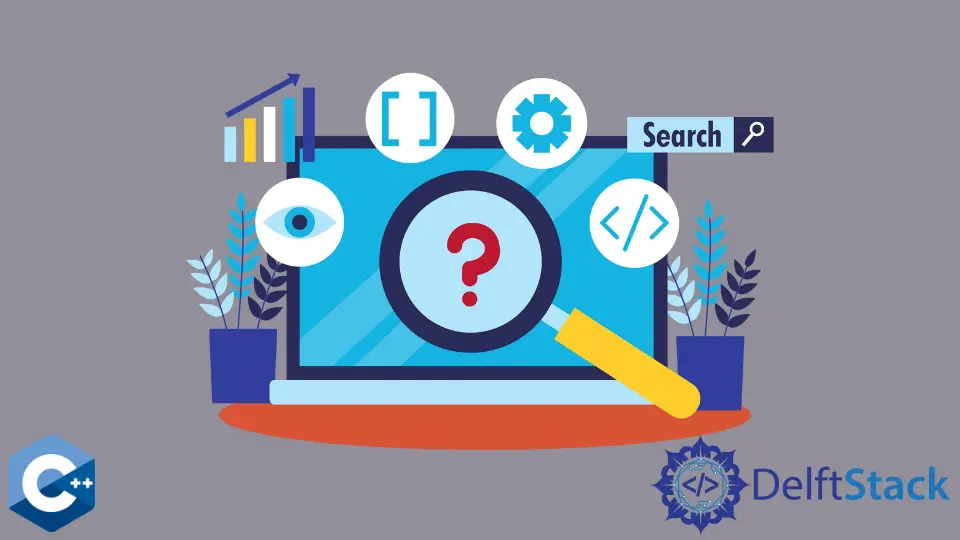
이 기사에서는 요소가 C++ 벡터에 존재하는지 확인하는 방법에 대한 여러 방법을 보여줍니다.
벡터에 요소가 있는지 확인하는 C++ std::find()
알고리즘
find
메소드는 STL 알고리즘 라이브러리의 일부입니다. 주어진 요소가 특정 범위에 존재하는지 확인할 수 있습니다. 이 함수는 사용자가 전달한 세 번째 매개 변수와 동일한 요소를 검색합니다. 해당하는 반환 값은 발견 된 첫 번째 요소에 대한 반복자이거나, 발견되지 않은 경우 범위의 끝이 반환됩니다.
다음 예제와 같이*
연산자를 사용하여 반환 된 문자열 값에 액세스하고if
문에서 비교 조건을 만듭니다.
++ cCopy#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::find;
using std::string;
using std::vector;
int main() {
string element_to_check1 = "nibble";
string element_to_check2 = "nimble";
vector<string> data_types = {"bit", "nibble", "byte", "char",
"int", "long", "long long", "float",
"double", "long double"};
if (*find(data_types.begin(), data_types.end(), element_to_check1) ==
element_to_check1) {
printf("%s is present in the vector\n", element_to_check1.c_str());
} else {
printf("%s is not present in the vector\n", element_to_check1.c_str());
}
if (*find(data_types.begin(), data_types.end(), element_to_check2) ==
element_to_check2) {
printf("%s is present in the vector\n", element_to_check2.c_str());
} else {
printf("%s is not present in the vector\n", element_to_check2.c_str());
}
return EXIT_SUCCESS;
}
출력:
textCopynibble is present in the vector
nimble is not present in the vector
벡터에 요소가 있는지 확인하는 C++ 범위 기반for
루프
범위 기반 for
루프는 벡터에 주어진 요소가 있는지 확인하기위한 또 다른 솔루션으로 사용할 수 있습니다. 이 방법은 벡터를 반복하기 때문에 비교적 간단합니다. 각 반복은 주어진 문자열과 같은지 확인합니다. 요소가 일치하면 확인 문자열이 인쇄되고break
문을 사용하여 루프가 중지됩니다.
++ cCopy#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string element_to_check = "nibble";
vector<string> data_types = {"bit", "nibble", "byte", "char",
"int", "long", "long long", "float",
"double", "long double"};
for (const auto &item : data_types) {
if (item == element_to_check) {
printf("%s is present in the vector\n", element_to_check.c_str());
break;
}
}
return EXIT_SUCCESS;
}
출력:
textCopynibble is present in the vector
벡터에 요소가 있는지 확인하는 C++ any_of()
알고리즘
find
메서드와 유사한<algorithm>
헤더의 또 다른 유용한 메서드는any_of
알고리즘입니다. any_of
메소드는 세 번째 인수로 지정된 단항 술어가 주어진 범위에서 하나 이상의 요소에 대해true
를 리턴하는지 확인합니다. 이 예에서는 람다 식을 사용하여 벡터 요소를 비교하기위한 단항 조건자를 생성합니다.
++ cCopy#include <algorithm>
#include <iostream>
#include <vector>
using std::any_of;
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string element_to_check = "nibble";
vector<string> data_types = {"bit", "nibble", "byte", "char",
"int", "long", "long long", "float",
"double", "long double"};
if (any_of(data_types.begin(), data_types.end(),
[&](const string& elem) { return elem == element_to_check; })) {
printf("%s is present in the vector\n", element_to_check.c_str());
}
return EXIT_SUCCESS;
}
출력:
textCopynibble is present in the vector
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook