문자열에 C의 하위 문자열이 포함되어 있는지 확인
-
strstr
함수를 사용하여 문자열에 C의 하위 문자열이 포함되어 있는지 확인 -
strcasestr
함수를 사용하여 문자열에 하위 문자열이 포함되어 있는지 확인 -
strncpy
함수를 사용하여 부분 문자열 복사
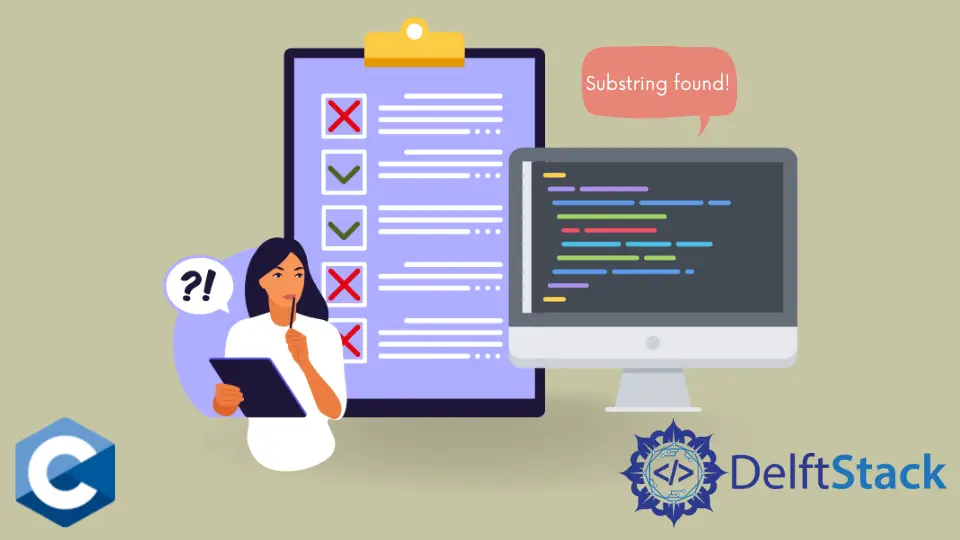
이 기사에서는 문자열에 C로 지정된 하위 문자열이 포함되어 있는지 확인하는 여러 방법에 대해 설명합니다.
strstr
함수를 사용하여 문자열에 C의 하위 문자열이 포함되어 있는지 확인
strstr
함수는 C 표준 라이브러리 문자열 기능의 일부이며<string.h>
헤더에 정의되어 있습니다. 이 함수는 두 개의char
포인터 인수를받습니다. 첫 번째는 검색 할 문자열을 나타내고 다른 하나는 검색 할 문자열을 나타냅니다. 주어진 부분 문자열의 첫 번째 시작 주소를 찾고 char
에 해당하는 포인터를 반환합니다. 첫 번째 인수 문자열에 하위 문자열이 없으면 NULL 포인터가 반환됩니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *tmp = "This string literal is arbitrary";
int main(int argc, char *argv[]) {
char *ret;
ret = strstr(tmp, "literal");
if (ret)
printf("found substring at address %p\n", ret);
else
printf("no substring found!\n");
exit(EXIT_SUCCESS);
}
출력:
found substring at address 0x55edd2ecc014
strcasestr
함수를 사용하여 문자열에 하위 문자열이 포함되어 있는지 확인
strcasestr
은 표준 라이브러리 기능의 일부가 아니지만_GNU_SOURCE
매크로 정의로 표시 할 수있는 GNU C 라이브러리의 확장으로 구현됩니다. 일단 정의되면strcasestr
함수를 호출하여 주어진 하위 문자열의 첫 번째 발생을 찾을 수 있습니다. 하지만이 함수는 두 문자열의 대소 문자를 모두 무시합니다.
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *tmp = "This string literal is arbitrary";
int main(int argc, char *argv[]) {
char *ret;
ret = strcasestr(tmp, "LITERAL");
if (ret)
printf("found substring at address %p\n", ret);
else
printf("no substring found!\n");
exit(EXIT_SUCCESS);
}
출력:
found substring at address 0x55edd2ecc014
strncpy
함수를 사용하여 부분 문자열 복사
또는strncpy
함수를 사용하여 주어진 하위 문자열을 새 버퍼에 복사 할 수 있습니다. 세 개의 인수가 필요하며 첫 번째는 복사 된 하위 문자열이 저장 될 대상 char
포인터입니다. 두 번째 인수는 소스 문자열이고 마지막 인수는 최대 복사 할 첫 번째 바이트 수를 나타냅니다. 소스 문자열의 첫 번째 바이트에서 null 바이트를 찾을 수없는 경우 대상 문자열은 null로 끝나지 않습니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char *tmp = "This string literal is arbitrary";
int main(int argc, char *argv[]) {
char *str = malloc(strlen(tmp));
printf("%s\n", strncpy(str, tmp, 4));
printf("%s\n", strncpy(str, tmp + 5, 10));
free(str);
exit(EXIT_SUCCESS);
}
출력:
This
string lit
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook