C에서 바이너리 파일 읽기
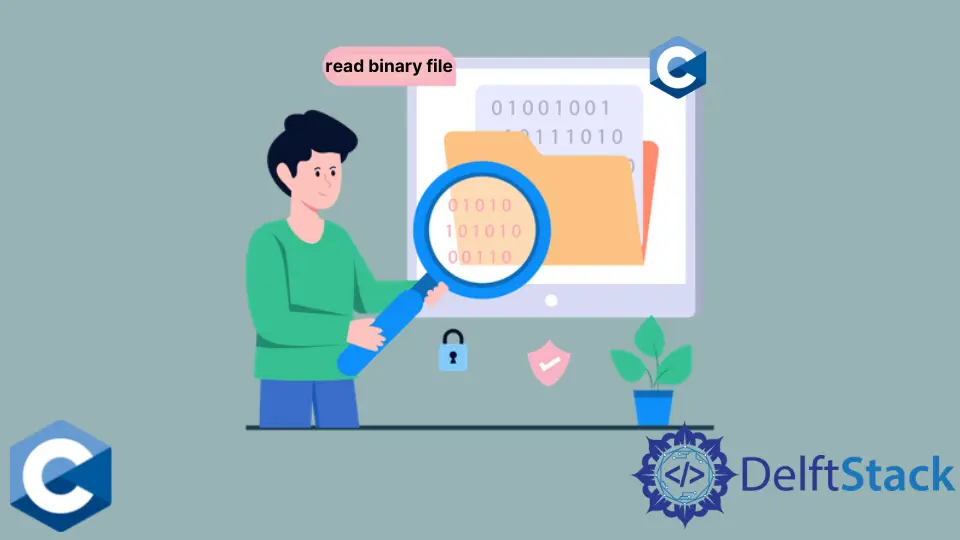
이 기사에서는 C로 바이너리 파일을 읽는 방법에 대한 여러 가지 방법을 보여줍니다.
fread
함수를 사용하여 C에서 이진 파일 읽기
fread
는 C 표준 라이브러리 입출력 기능의 일부이며 일반 파일에서 바이너리 데이터를 읽는 데 사용할 수 있습니다. C 표준 라이브러리는 이진 파일 데이터 읽기/쓰기를 처리하기 위해 플랫폼 독립적 인 솔루션과 함께 사용자 버퍼 I/O를 구현합니다. 표준 I/O 함수는 파일 설명자 대신 파일 포인터에서 작동합니다. FILE *
스트림은fopen
함수에 의해 검색됩니다.이 함수는 파일 경로를 문자열 상수로 사용하고이를 여는 모드를 사용합니다. 파일 모드는 읽기, 쓰기 또는 추가를 위해 파일을 열지 여부를 지정합니다. 하지만 각 모드 문자열에는 2 진 파일 모드를 명시 적으로 지정하기 위해 문자 b
가 포함될 수 있습니다. 이는 텍스트와 바이너리 파일을 다르게 처리하는 일부 비 UNIX 시스템에서 해석 할 수 있습니다.
fopen
이 파일 포인터를 반환 한 후fread
함수를 호출하여 바이너리 스트림을 읽을 수 있습니다. fread
는 4 개의 인수를 취하며, 그 중 첫 번째는 읽기 바이트가 저장되어야하는 위치에 대한void
포인터입니다. 다음 두 인수는 주어진 파일에서 읽어야하는 데이터 항목의 크기와 수를 지정합니다. 마지막으로 함수의 네 번째 인수는 데이터를 읽어야하는FILE
포인터입니다. 다음 예에서는input.txt
라는 파일을 열고 임의의 바이트를 씁니다. 그런 다음 파일을 닫고 읽기 위해 다시 엽니 다.
#include <fcntl.h>
#include <inttypes.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <unistd.h>
const uint8_t data[] = {0x54, 0x65, 0x6d, 0x70, 0x6f, 0x72, 0x61, 0x72,
0x79, 0x20, 0x73, 0x74, 0x72, 0x69, 0x6e, 0x67,
0x20, 0x74, 0x6f, 0x20, 0x62, 0x65, 0x20, 0x77,
0x72, 0x69, 0x74, 0x74, 0x65, 0x6e, 0x20, 0x74,
0x6f, 0x20, 0x66, 0x69, 0x6c, 0x65};
const char* filename = "input.txt";
int main(void) {
FILE* output_file = fopen(filename, "wb+");
if (!output_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
fwrite(data, 1, sizeof data, output_file);
printf("Done Writing!\n");
fclose(output_file);
FILE* in_file = fopen(filename, "rb");
if (!in_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
char* file_contents = malloc(sb.st_size);
fread(file_contents, sb.st_size, 1, in_file);
printf("read data: %s\n", file_contents);
fclose(in_file);
free(file_contents);
exit(EXIT_SUCCESS);
}
출력:
Done Writing!
read data: Temporary string to be written to file
read
기능을 사용하여 C에서 이진 파일 읽기
또는 본질적으로 후드 아래의 시스템 호출 인read
함수를 사용할 수 있습니다. read
는 파일 설명자에서 작동합니다. 따라서 파일은open
시스템 호출로 열어야합니다. 읽은 데이터가 저장 될void
포인터와 파일에서 읽을 바이트 수를 나타내는 추가 두 개의 인수가 필요합니다. 파일의 전체 내용을 읽고malloc
함수를 사용하여 동적으로 메모리를 할당합니다. stat
시스템 호출을 사용하여 파일 크기를 찾습니다.
#include <fcntl.h>
#include <inttypes.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <unistd.h>
const uint8_t data[] = {0x54, 0x65, 0x6d, 0x70, 0x6f, 0x72, 0x61, 0x72,
0x79, 0x20, 0x73, 0x74, 0x72, 0x69, 0x6e, 0x67,
0x20, 0x74, 0x6f, 0x20, 0x62, 0x65, 0x20, 0x77,
0x72, 0x69, 0x74, 0x74, 0x65, 0x6e, 0x20, 0x74,
0x6f, 0x20, 0x66, 0x69, 0x6c, 0x65};
const char* filename = "input.txt";
int main(void) {
FILE* output_file = fopen(filename, "wb+");
if (!output_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
fwrite(data, 1, sizeof data, output_file);
printf("Done Writing!\n");
fclose(output_file);
int fd = open(filename, O_RDONLY);
if (fd == -1) {
perror("open\n");
exit(EXIT_FAILURE);
}
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
char* file_contents = malloc(sb.st_size);
read(fd, file_contents, sb.st_size);
printf("read data: %s\n", file_contents);
close(fd);
free(file_contents);
exit(EXIT_SUCCESS);
}
출력:
Done Writing!
read data: Temporary string to be written to file
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook