C에서 변수가 할당되는 방법
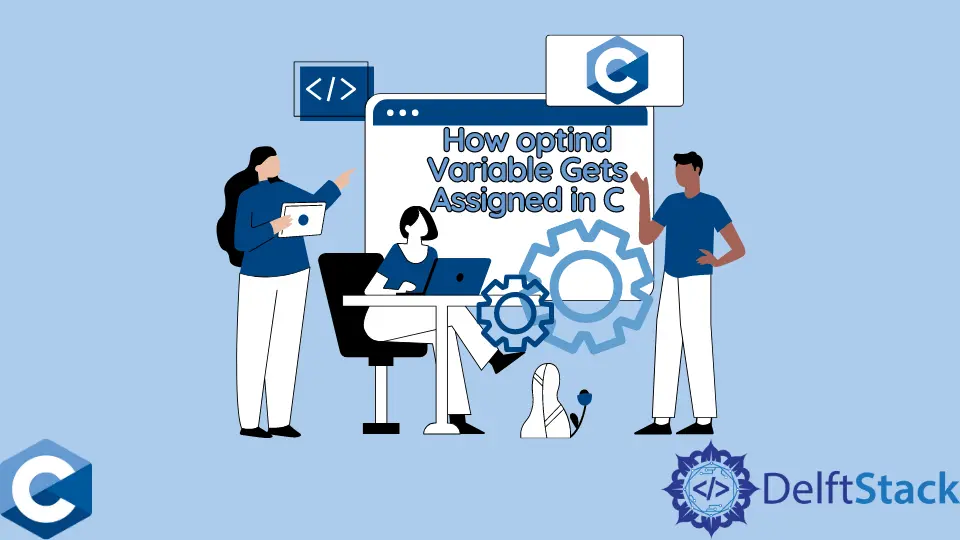
이 자료에서는 C에서 optind
변수가 어떻게 할당되는지에 대한 여러 가지 방법을 시연한다.
getopt
함수를 사용하여 C에서 명령 줄 옵션 구문 분석
UNIX 기반 시스템의 일반적인 명령 줄 프로그램은 인수와 옵션을 사용합니다. 옵션은 일반적으로 하이픈 다음에 오는 문자로 지정되며 각 고유 문자는 주어진 인수에 대한 정보를 나타내거나 실행할 프로그램의 특정 동작을 생성합니다. 옵션은 필수 또는 선택적 인수를 가질 수 있습니다. 후자의 경우 프로그램에서 단일 하이픈 뒤에 옵션 그룹화를 허용하는 것이 일반적이며 명령 끝에 유일한 인수가 전달 될 수 있습니다.
getopt
함수는 옵션을 구문 분석하고 프로그램에서 주어진 인수를 검색하고 입력에 따라 해당 코드 경로를 실행하는 데 사용됩니다. getopt
는 세 개의 인수를 취하며, 그 중 처음 두 개는main
함수에 전달 된argc
및argv
입니다. 세 번째 인수는optstring
-합법적 인 옵션 문자를 나타내는 문자열에 대한 포인터입니다. 모든 옵션이 검색 될 때까지getopt
를 연속적으로 호출해야합니다.
다음 예에서는p
및x
문자로 옵션을 사용하는 프로그램을 보여줍니다. optstring
은 누락 된 인수가 발견 될 때getopt
호출이 리턴해야하는 값을 나타내는 콜론으로 시작합니다. 또한 문자 뒤에 지정된 콜론 (예 :p
))은 옵션에 인수가 필요함을 나타냅니다. 두 개의 콜론은 인수가 선택 사항임을 의미합니다. 명령 줄에서 동일한 옵션 문자를 여러 번 전달할 수 있습니다. getopt
함수는 성공하면 옵션 문자를 반환하고, 발견 된 옵션 문자가optstring
에 없으면?
문자를 반환합니다.
#include <getopt.h>
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
int opt, xnum = 0;
char *pstr = NULL;
while ((opt = getopt(argc, argv, ":p:x")) != -1) {
printf("opt = %3d (%c); optind = %d\n", opt, opt, optind);
switch (opt) {
case 'p':
pstr = optarg;
break;
case 'x':
xnum++;
break;
case ':':
fprintf(stderr,
"Missing argument!\n"
"Usage: %s [-p arg] [-x]\n",
argv[0]);
exit(EXIT_FAILURE);
case '?':
fprintf(stderr,
"Unrecognized option!\n"
"Usage: %s [-p arg] [-x]\n",
argv[0]);
exit(EXIT_FAILURE);
default:
fprintf(stderr, "Unexpected case in switch()");
exit(EXIT_FAILURE);
}
}
exit(EXIT_SUCCESS);
}
샘플 명령 :
./program_name -p hello -p there
출력:
opt = 112 (p); optind = 3
opt = 112 (p); optind = 5
optind
및optarg
변수를 사용하여 C의argv
요소 처리
이전 코드 샘플은 일반적인getopt
사용법을 보여줍니다. 여기서 함수는 오류 코드-1
을 리턴 할 때까지while
루프 표현식에서 호출됩니다. 한편switch
문은 가능한getopt
반환 값을 확인하여 해당 코드 블록을 실행합니다. optind
변수는argv
에서 다음 요소의 색인을 나타내며getopt
를 처음 호출하기 전에1
로 초기화됩니다. 반면optarg
는 현재 옵션 문자 다음에 오는 인수를 가리키는 외부 변수입니다. 옵션에 인수가 포함되지 않은 경우optarg
가 0으로 설정됩니다. 다음 코드 예제는optarg
변수가 가리키는 인수를 저장하고 필요에 따라 작동하는 방법을 보여줍니다. 인수는 공백 구분 기호없이 옵션 뒤에 올 수 있습니다.
#include <getopt.h>
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[]) {
int opt, xnum = 0;
char *pstr = NULL;
while ((opt = getopt(argc, argv, ":p:x")) != -1) {
printf("opt = %3d (%c); optind = %d\n", opt, opt, optind);
switch (opt) {
case 'p':
pstr = optarg;
break;
case 'x':
xnum++;
break;
case ':':
fprintf(stderr,
"Missing argument!\n"
"Usage: %s [-p arg] [-x]\n",
argv[0]);
exit(EXIT_FAILURE);
case '?':
fprintf(stderr,
"Unrecognized option!\n"
"Usage: %s [-p arg] [-x]\n",
argv[0]);
exit(EXIT_FAILURE);
default:
fprintf(stderr, "Unexpected case in switch()");
exit(EXIT_FAILURE);
}
}
if (xnum != 0) printf("-x was specified (count=%d)\n", xnum);
if (pstr != NULL) printf("-p was specified with the value \"%s\"\n", pstr);
if (optind < argc)
printf("First non-option argument is \"%s\" at argv[%d]\n", argv[optind],
optind);
exit(EXIT_SUCCESS);
}
샘플 명령 :
./program_name -p hello
출력:
opt = 112 (p); optind = 3
-p was specified with the value "hello"
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook