C의 연결 목록에서 노드에 할당된 메모리 해제
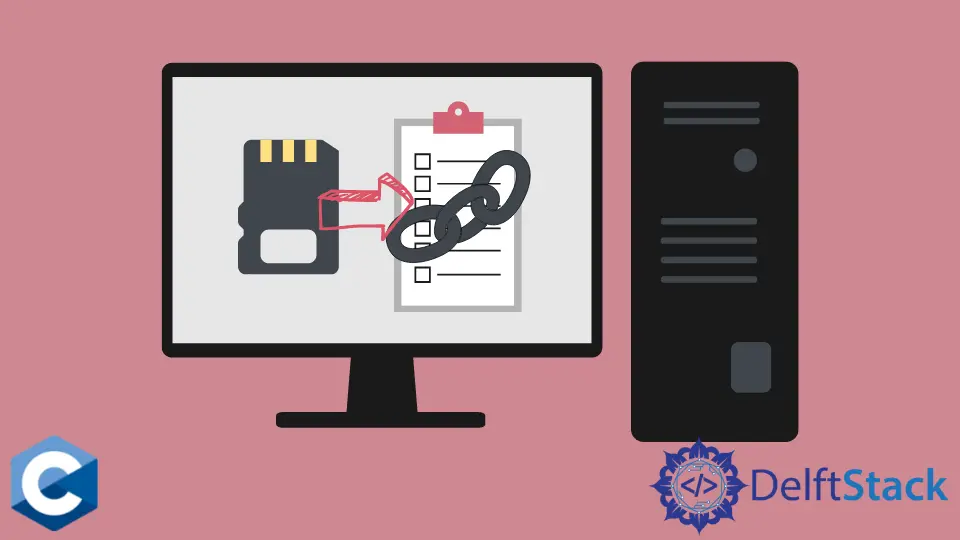
오늘은 C에서 링크드 리스트의 노드에 할당된 메모리를 해제하거나 해제하는 방법을 알아보겠습니다.
C의 연결 목록에서 노드에 할당된 메모리 해제
이 기사에서는 free()
함수를 사용하여 malloc()
함수에 의해 예약되거나 할당된 메모리를 해제합니다. 이는 우리가 malloc()
함수를 호출할 때마다 해당 free()
함수를 호출하여 메모리를 해제해야 함을 의미합니다.
C 프로그래밍에서 링크드 리스트를 사용하는 동안 링크드 리스트의 모든 노드는 malloc()
함수가 호출되는 즉시 메모리가 할당됩니다. 따라서 노드 또는 전체 연결 목록이 더 이상 필요하지 않을 때마다 free()
메서드를 호출하여 할당된 메모리를 해제합니다.
3개의 노드를 포함하는 연결 목록이 있고 이 연결 목록이 지금 필요하지 않다고 결정했다고 가정합니다. 루프를 사용하여 연결된 목록을 반복하고 각 노드에 할당된 메모리를 해제할 수 있습니다.
루트 노드에서 free()
함수를 호출하여 연결 목록의 전체 구조를 해제할 수는 없습니다. 실질적으로 이해하기 위해 두 가지 코드 예제를 사용하고 있습니다.
- 완전한 연결 목록을 인쇄하고 모든 노드에 할당된 메모리를 해제합니다.
- 현재 노드를 인쇄하고 다음 노드의 포인터를 저장하고 현재 노드의 메모리를 비웁니다. 이것은 우리가 하나의 루프에서 할당된 메모리를 인쇄하고 해제한다는 것을 의미합니다.
전체 연결 목록 인쇄 및 할당된 메모리 해제
이 시나리오를 실제로 배우기 위해 연결 목록 노드를 다음과 같이 정의합니다. C 프로그래밍이 허용하는 재귀 방식으로 구조를 정의합니다.
또한 struct
대신 typedef struct
를 사용하여 보다 체계적이고 깔끔한 코드를 작성하고 있습니다. typedef struct
와 struct
의 차이점은 여기에서 자세히 설명합니다.
cCopytypedef struct node {
int data;
struct node* next;
} nodes;
makeList()
기능을 사용하여 모든 노드를 채웁니다. 여기에서는 연결된 목록에 세 개의 노드만 있습니다.
cCopystruct node* makeList() {
nodes* headNode = NULL;
nodes* secondNode = NULL;
nodes* thirdNode = NULL;
headNode = malloc(sizeof(nodes));
secondNode = malloc(sizeof(nodes));
thirdNode = malloc(sizeof(nodes));
headNode->data = 1;
headNode->next = secondNode;
secondNode->data = 2;
secondNode->next = thirdNode;
thirdNode->data = 3;
thirdNode->next = NULL;
return headNode;
}
printList()
메서드(아래)는 연결된 목록을 반복하고 모든 목록 노드를 인쇄합니다. 여기에서 headNode
를 참조하는 currentNode
를 생성했습니다.
그런 다음 while
루프를 사용하여 currentNode
가 NULL
인지 확인합니다. 그렇지 않은 경우 currentNode
데이터를 인쇄하고 currentNode
에 다음 노드의 주소를 저장합니다.
이 프로세스는 currentNode
가 NULL
과 같을 때까지 계속됩니다.
cCopyvoid printList(nodes* headNode) {
nodes* currentNode = headNode;
while (currentNode != NULL) {
printf("The current element is %d\n", currentNode->data);
currentNode = currentNode->next;
}
}
전체 연결 목록을 인쇄한 후 freeList()
함수(아래)는 각 노드에 할당된 메모리를 해제합니다.
freeList()
함수는 headNode
가 NULL
인지 확인합니다. NULL
이면 목록이 비어 있으므로 이 함수에서 즉시 반환합니다.
둘째, currentNode
변수에 headNode
를 저장하고 headNode
가 목록의 바로 다음 노드를 가리키도록 합니다. headNode = headNode->next;
에서 수행하고 있습니다.
셋째, free(currentNode)
를 사용하여 할당된 메모리를 안전하게 해제합니다. 이제 headNode
는 나머지 목록을 가리키고 첫 번째 단계로 돌아갑니다.
cCopyvoid freeList(nodes* headNode) {
nodes* currentNode;
while (headNode != NULL) {
currentNode = headNode;
headNode = headNode->next;
free(currentNode);
}
}
마지막으로 main()
메서드를 사용하여 다음과 같이 함수를 호출하고 목표를 달성합니다.
cCopyint main() {
nodes* headNode = makeList();
printList(headNode);
freeList(headNode);
return 0;
}
완전한 예제 코드
모든 코드 청크를 조립하면 전체 프로그램은 아래와 같이 보입니다.
cCopy#include <stdio.h>
typedef struct node {
int data;
struct node* next;
} nodes;
struct node* makeList() {
nodes* headNode = NULL;
nodes* secondNode = NULL;
nodes* thirdNode = NULL;
headNode = malloc(sizeof(nodes));
secondNode = malloc(sizeof(nodes));
thirdNode = malloc(sizeof(nodes));
headNode->data = 1;
headNode->next = secondNode;
secondNode->data = 2;
secondNode->next = thirdNode;
thirdNode->data = 3;
thirdNode->next = NULL;
return headNode;
}
void printList(nodes* headNode) {
nodes* currentNode = headNode;
while (currentNode != NULL) {
printf("The current element is %d\n", currentNode->data);
currentNode = currentNode->next;
}
}
void freeList(nodes* headNode) {
nodes* currentNode;
while (headNode != NULL) {
currentNode = headNode;
headNode = headNode->next;
free(currentNode);
}
}
int main() {
nodes* headNode = makeList();
printList(headNode);
freeList(headNode);
return 0;
}
출력:
textCopyThe current element is 1
The current element is 2
The current element is 3
현재 노드 인쇄, 다음 노드에 대한 포인터 저장, 현재 노드에 할당된 메모리 해제
이 코드 예제에서는 현재 노드를 인쇄하고 다음 노드의 주소를 저장하고 현재 노드에 할당된 메모리를 해제합니다.
두 가지를 제외하고 모든 것이 이전 예제와 동일합니다. 첫 번째로 freeList()
함수가 없고 두 번째로 printList()
대신 printAndFreeList()
가 있습니다.
printAndFreeList()
함수에서 목록을 반복하고 현재 노드를 인쇄하고 나머지 연결된 목록에 대한 포인터를 저장하고 현재 노드에 대한 메모리를 해제합니다.
이 프로세스는 NULL
이 발견될 때까지 계속됩니다.
cCopyvoid printAndFreeList(nodes* currentNode) {
nodes* tempNode;
while (currentNode != NULL) {
tempNode = currentNode;
printf("The current element is %d\n", currentNode->data);
currentNode = currentNode->next;
free(tempNode);
}
}
완전한 예제 코드
전체 프로그램은 다음과 같습니다.
cCopy#include <stdio.h>
typedef struct node {
int data;
struct node* next;
} nodes;
struct node* makeList() {
nodes* headNode = NULL;
nodes* secondNode = NULL;
nodes* thirdNode = NULL;
headNode = malloc(sizeof(nodes));
secondNode = malloc(sizeof(nodes));
thirdNode = malloc(sizeof(nodes));
headNode->data = 1;
headNode->next = secondNode;
secondNode->data = 2;
secondNode->next = thirdNode;
thirdNode->data = 3;
thirdNode->next = NULL;
return headNode;
}
void printAndFreeList(nodes* currentNode) {
nodes* tempNode;
while (currentNode != NULL) {
tempNode = currentNode;
printf("The current element is %d\n", currentNode->data);
currentNode = currentNode->next;
free(tempNode);
}
}
int main() {
nodes* headNode = makeList();
printAndFreeList(headNode);
return 0;
}
출력:
textCopyThe current element is 1
The current element is 2
The current element is 3