C에서 구조체와 Typedef 구조체의 포워드 선언과 차이점
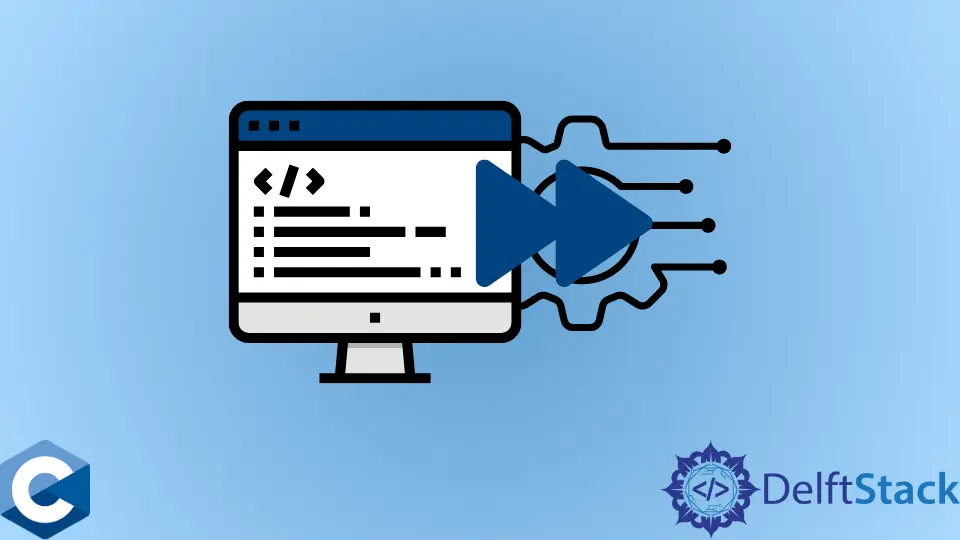
이 자습서에서는 struct
의 개념과 typedef
키워드의 사용에 대해 설명합니다. 우리는 또한 C에서 전방 선언
의 개념을 볼 것입니다.
C 프로젝트를 생성하여 시작하겠습니다.
C 프로젝트 생성
-
첫 번째 단계는 컴파일러를 설치하는 것입니다. C 컴파일러 다운로드 및 설치 단계.
-
다음 단계에서 메뉴 표시줄에서
파일
을 클릭하여 C 언어로 빈 프로젝트를 만듭니다. -
컴파일하기 전에 파일을 저장하십시오.
-
코드를 실행합니다.
컴파일 및 실행
을 클릭합니다. -
실행 화면이 나타납니다.
C 언어의 구조체
Struct는 관련 데이터 구성원
그룹을 만들기 위해 C 언어에서 사용됩니다. 구조체의 멤버는 동일한 데이터 유형을 가지고 있지 않으며 그룹에는 한 곳에 보관된 다양한 변수가 포함되어 있습니다.
아시다시피 해당 배열에는 동일한 유형의 멤버가 있지만 구조체에서 데이터 멤버는 int
, float
및 char
와 같은 다른 유형일 수 있습니다. 다음 코드 스니펫은 C 언어로 구조체를 만드는 방법을 설명합니다.
이 코드에서는 struct name
과 variable
을 작성하여 main function
의 local scope
에서 변수를 선언했습니다.
코드 예:
#include <stdio.h>
struct Student { // Structure declaration
int rollno; // Member (int variable)
char gender; // Member (char variable)
}; // End the structure with a semicolon
int main() {
struct Student s1; // Making a variable of a struct
s1.rollno = 3; // assigning value to every member using dot operator
s1.gender = 'M';
printf("The name and gender of a student is %d and %c", s1.rollno, s1.gender);
}
출력:
The name and gender of a student is 3 and M
다음 코드에서 전역 범위의 변수를 선언합니다.
코드 예:
#include <stdio.h>
struct Student { // Structure declaration
int rollno; // Member (int variable)
char gender; // Member (char variable)
} s1; // Declared a variable s1 before ending the struct with a semicolon
int main() {
s1.rollno = 3; // calling a member using a variable
s1.gender = 'M';
printf("The name and gender of a student is %d and %c", s1.rollno, s1.gender);
}
출력:
The name and gender of a student is 3 and M
이번에도 동일한 출력을 제공하지만 위의 코드 스니펫에서는 변수 s1
을 전역적으로 선언했습니다. main()
함수에서 구조체를 정의할 필요가 없습니다.
C의 typedef
typedef
는 C 언어에서 기존 데이터 유형의 이름을 새 이름으로 바꾸는 데 사용됩니다.
통사론:
typedef<existing_name><alias_name>
먼저 typedef
의 예약어
를 작성하고 C 언어로 된 기존 이름과 지정하려는 이름을 작성해야 합니다. typedef
를 사용한 후 전체 프로그램에서 alias_name
을 사용합니다.
암호:
#include <stdio.h>
void main() {
typedef int integer; // assigning int a new name of the integer
integer a = 3; // variable is declared by integer
integer b = 4;
printf("The values of a and b are %d and %d", a,
b); // print value of a and b on the screen
}
출력:
The values of a and b are 3 and 4
C의 typedef
구조체
우리는 main()
함수에 전체 구조체 정의를 작성해야 한다는 것을 보았습니다. 매번 struct student
를 작성하는 대신 typedef를 사용하여 이전 유형을 새 유형으로 바꿀 수 있습니다.
typedef
는 C 언어로 유형을 만드는 데 도움이 됩니다.
코드 예:
#include <stdio.h> // including header file of input/output
#include <string.h> // including header file of string
typedef struct Books { // old type
char title[30]; // data members of the struct book
char author[30];
char subject[50];
int id;
} Book; // new type
void main() {
Book b1; // variable of a new type
strcpy(b1.title, "C Programming"); // copy string in title
strcpy(b1.author, "Robert Lafore"); // copy string in author
strcpy(b1.subject, "Typedef Struct in C"); // copy string in subject
b1.id = 564555; // assigning id to variable
printf("Book title is : %s\n", b1.title); // printing book title on screen
printf("Book author is : %s\n", b1.author);
printf("Book subject is : %s\n", b1.subject);
printf("Book id is : %d\n", b1.id);
}
출력:
Book title is : C Programming
Book author is : Robert Lafore
Book subject is : Typedef Struct in C
Book id is : 564555
위의 코드에서 세미콜론 앞의 구조체 끝에 새 구조체 유형을 정의했습니다. 그런 다음 main()
함수에서 새 유형을 사용했습니다.
이 typedef
는 변수를 만들기 위해 main()
함수에서 매번 구조체를 정의하는 수고를 줄였습니다.
C의 전방 선언
전방 선언은 Struct의 실제 정의 앞에 오는 선언입니다. 정의는 사용할 수 없지만 사전 선언인 전방 선언으로 인해 선언된 유형을 참조할 수 있습니다.
이 메서드는 함수를 정의하고 선언하는 데 사용됩니다. main()
함수 위에 함수를 정의하는 대신 맨 위에 선언하고 맨 아래에 정의할 수 있습니다. 이를 전방 선언이라고 합니다.
코드 예:
#include <stdio.h>
int add(int x, int y); // (prototype)function declaration
void main() {
int n1, n2, sum;
scanf("%d %d", &n1, &n2); // taking numbers from user
sum = add(n1, n2); // call to the add function
printf("The sum of n1 and n2 is %d ", sum); // display sum on the screen
}
int add(int x, int y) // function definition
{
int result;
result = x + y;
return result; // returning the result to the main function
}
위의 코드 스니펫은 main()
함수 맨 위에 함수 프로토타입을 선언했음을 보여줍니다. 정의하기 전에 main에서 add()
함수를 호출하지만 프로토타입이 이미 정의되어 있으므로 제대로 작동합니다.
이는 전방 선언 때문입니다.
출력:
4 5
The sum of n1 and n2 is 9