C에서 난수 생성
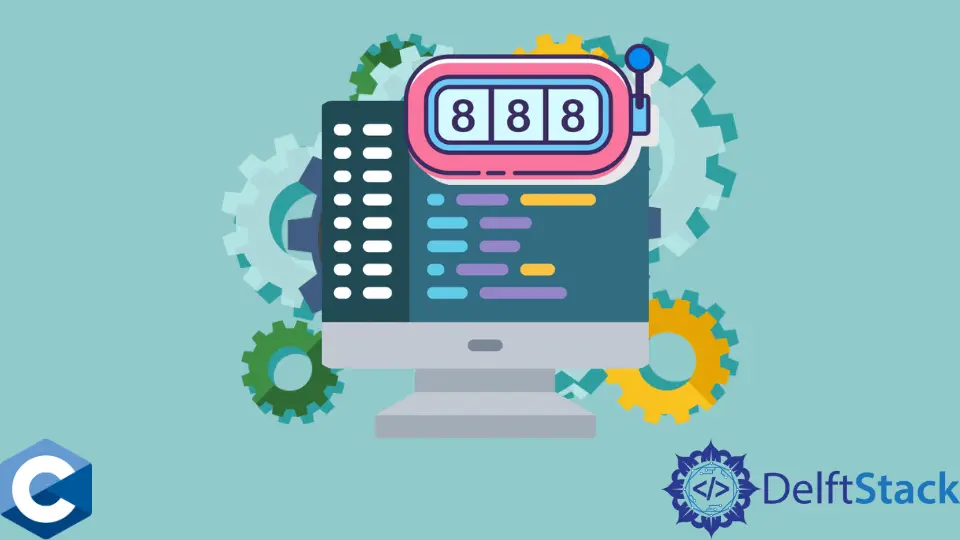
이 기사에서는 C에서 난수를 생성하는 방법에 대한 몇 가지 방법을 소개합니다.
rand
및srand
함수를 사용하여 C에서 난수 생성
rand
함수는[0, RAND_MAX]
범위의 정수를 제공 할 수있는 의사 난수 생성기를 구현합니다. 여기서RAND_MAX
는 최신 시스템에서 231 -1입니다. rand
함수 뒤에있는 생성기 알고리즘은 결정적입니다. 따라서 무작위 비트로 시드해야합니다.
srand
함수는 의사 난수 생성기를 시드하는 데 사용되며 이후rand
를 호출하면 무작위 정수 시퀀스가 생성됩니다. 단점은 rand
구현이 균일하게 임의의 비트를 생성 할 것으로 예상되지 않는다는 것입니다. 따라서 rand
함수는 암호화가 매우 민감한 애플리케이션에서 사용하지 않는 것이 좋습니다. 다음 예제는 임의성의 좋은 소스가 아닌 현재 시간 값으로 생성기를 시드합니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX 100000
#define SIZE 100
#define NUMS_TO_GENERATE 10
int main() {
srand(time(NULL));
for (int i = 0; i < NUMS_TO_GENERATE; i++) {
printf("%d\n", rand() % MAX);
}
exit(EXIT_SUCCESS);
}
출력:
85084
91989
85251
85016
43001
54883
8122
84491
6195
54793
random
및srandom
함수를 사용하여 C에서 난수 생성
C 표준 라이브러리에서 사용할 수있는 또 다른 의사 난수 생성기는 random
함수로 구현됩니다. 이 방법은 rand
에 비해 선호되는 방법이지만 암호화 응용 프로그램은 민감한 코드에서 random
기능을 사용해서는 안됩니다. random
은 인수를 취하지 않으며[0, RAND_MAX]
범위의long int
유형 정수를 반환합니다. 비교적 좋은 품질의 난수를 생성하려면 함수에 srandom
함수를 추가하는 것이 좋습니다.
이전 예와 마찬가지로 time
함수를 사용하여 현재 시간 값을 시드로 전달하므로 보안에 민감한 애플리케이션에서는 권장되지 않습니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/random.h>
#define MAX 100000
#define SIZE 100
#define NUMS_TO_GENERATE 10
int main() {
srandom(time(NULL));
for (int i = 0; i < NUMS_TO_GENERATE; i++) {
printf("%ld\n", random() / MAX);
}
printf("\n");
exit(EXIT_SUCCESS);
}
출력:
91
2019
2410
11784
9139
5858
5293
17558
16625
3069
getrandom
함수를 사용하여 C에서 난수 생성
getrandom
은 이전에 제공된 두 가지 방법보다 훨씬 우수한 품질의 임의 비트를 얻는 Linux 전용 함수입니다. getrandom
은 임의의 비트를 저장해야하는 버퍼를 가리키는void
포인터, 바이트 단위의 버퍼 크기, 특수 기능에 대한 플래그의 세 가지 인수를받습니다.
다음 예에서는 단일 unsigned
정수를 생성합니다.이 주소의 주소는 &tmp
가 임의의 비트를 저장하기위한 버퍼로 전달되고 크기는 sizeof
연산자로 계산됩니다. 드문 시나리오에서getrandom
이 비트를 검색하는 임의의 소스는 초기화되지 않을 수 있습니다. getrandom
함수를 호출하면 프로그램 실행이 차단됩니다. 따라서GRND_NONBLOCK
매크로 정의는 이러한 경우 즉시 오류 값 -1을 반환하는 함수의 세 번째 인수로 전달됩니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/random.h>
#include <time.h>
#define MAX 100000
#define SIZE 100
#define NUMS_TO_GENERATE 10
int main() {
unsigned int tmp;
getrandom(&tmp, sizeof(unsigned int), GRND_NONBLOCK) == -1
? perror("getrandom")
: "";
printf("%u\n", tmp);
exit(EXIT_SUCCESS);
}
934103271
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook