Angular에서 개체 속성으로 필터링
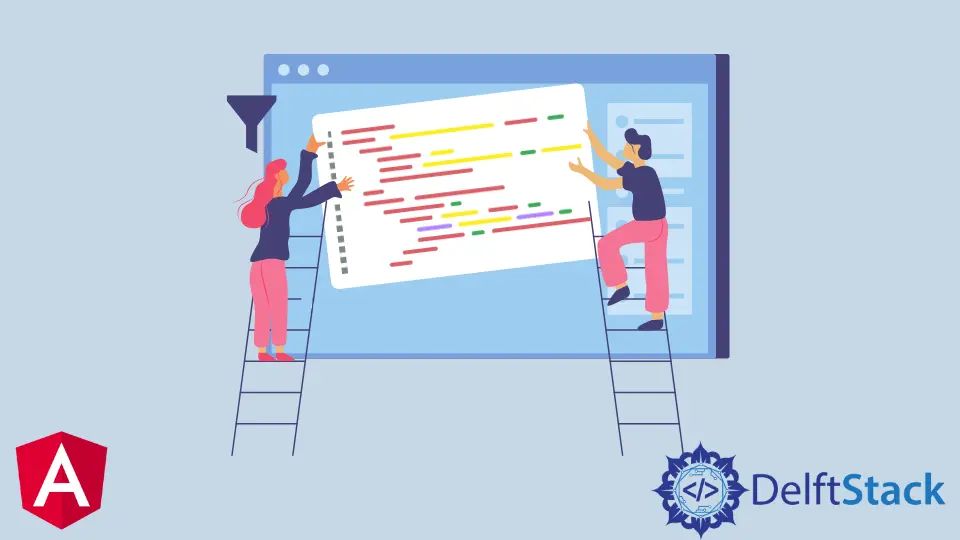
웹 페이지의 필터 기능을 사용하면 항목이 긴 항목 목록에 있는지 여부를 평가하려는 경우와 같이 사용자가 검색 범위를 빠르게 좁힐 수 있습니다. 또한 자동차 목록과 같은 목록을 필터링하려는 경우 필터 기능을 사용할 수 있습니다.
속성으로 객체를 필터링하기 위해 문자열, 숫자 등으로 목록을 필터링할 수 있습니다. 이 기사에서는 이제 웹 페이지 내에서 필터를 수행하는 다양한 방법을 살펴보겠습니다.
Angular의 모든 객체 속성으로 필터링
이 예제에서는 문자열과 숫자 등 모든 기준으로 필터링할 수 있는 웹 앱을 만들고자 합니다. 따라서 새 프로젝트 폴더를 만든 다음 index.html
파일로 이동하여 코드를 작성합니다.
코드 스니펫 - index.html
:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Example Filter</title>
<script src="//code.angularjs.org/snapshot/angular.min.js"></script>
</head>
<body ng-app="">
<div ng-init="friends = [{name:'John', phone:'555-1276'},
{name:'Mary', phone:'800-BIG-MARY'},
{name:'Mike', phone:'555-4321'},
{name:'Adam', phone:'555-5678'},
{name:'Julie', phone:'555-8765'},
{name:'Juliette', phone:'555-5678'}]"></div>
<label>Search: <input ng-model="searchText"></label>
<table id="searchTextResults">
<tr><th>Name</th><th>Phone</th></tr>
<tr ng-repeat="friend in friends | filter:searchText">
<td>{{friend.name}}</td>
<td>{{friend.phone}}</td>
</tr>
</table>
<hr>
</table>
</body>
</html>
개체 배열을 테이블로 구성한 다음 개체를 필터링하는 데 사용할 입력 표시줄도 제공됩니다. 그런 다음 filter.js
라는 새 파일을 만들어야 합니다. 여기에서 앱의 기능을 추가합니다.
코드 스니펫 - filter.js
:
var expectFriendNames = function(expectedNames, key) {
element.all(by.repeater(key + ' in friends').column(key + '.name')).then(function(arr) {
arr.forEach(function(wd, i) {
expect(wd.getText()).toMatch(expectedNames[i]);
});
});
};
it('should search across all fields when filtering with a string', function() {
var searchText = element(by.model('searchText'));
searchText.clear();
searchText.sendKeys('m');
expectFriendNames(['Mary', 'Mike', 'Adam'], 'friend');
searchText.clear();
searchText.sendKeys('76');
expectFriendNames(['John', 'Julie'], 'friend');
});
그래서 검색 입력을 위한 함수를 만든 다음 searchText 변수 내에서 모델을 호출했습니다. 아래 출력을 참조하십시오.
Angular에서 특정 개체 속성으로 필터링
이번에는 특정 속성으로 필터링할 수 있는 앱을 만들고자 합니다. 여기서 문자열로 필터링하고 문자열만 작동하고 숫자로 필터링하고 숫자만 작동한 다음 검색 필터도 사용할 수 있습니다. 어디에서나 문자열과 숫자로 검색할 수 있습니다.
새로운 Angular 프로젝트를 수행하고 index.html
파일을 탐색하여 페이지 구조를 생성할 코드를 추가합니다. 여기에는 숫자로만, 문자열로만 검색할 수 있는 검색 표시줄이 포함됩니다.
코드 스니펫 - index.html
:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Example - example-filter-filter-production</title>
<script src="//code.angularjs.org/snapshot/angular.min.js"></script>
</head>
<body ng-app="">
<div ng-init="friends = [{name:'John', phone:'555-1276'},
{name:'Mary', phone:'555-2578'},
{name:'Mike', phone:'555-4321'},
{name:'Adam', phone:'555-5678'},
{name:'Julie', phone:'555-8765'},
{name:'Juliette', phone:'555-5678'}]">
</div>
<hr>
<label>Any: <input ng-model="search.$"></label> <br>
<label>Name only <input ng-model="search.name"></label><br>
<label>Phone only <input ng-model="search.phone"></label><br>
<table id="searchObjResults">
<tr><th>Name</th><th>Phone</th></tr>
<tr ng-repeat="friendObj in friends | filter:search:strict">
<td>{{friendObj.name}}</td>
<td>{{friendObj.phone}}</td>
</tr>
</table>
</body>
</html>
이 시점에서 위에서 정의한 구조의 웹 페이지가 표시되어야 합니다. 이제 앱의 기능을 디자인하는 것입니다.
새 파일을 만들고 filter.js
라는 이름을 지정한 다음 다음 코드를 입력합니다.
코드 스니펫 - filter.js
:
var expectFriendNames = function(expectedNames, key) {
it('should search in specific fields when filtering with a predicate object', function() {
var searchAny = element(by.model('search.$'));
searchAny.clear();
searchAny.sendKeys('i');
expectFriendNames(['Mary', 'Mike', 'Julie', 'Juliette'], 'friendObj');
});
it('should use a equal comparison when comparator is true', function() {
var searchName = element(by.model('search.name'));
var strict = element(by.model('strict'));
searchName.clear();
searchName.sendKeys('Julie');
strict.click();
expectFriendNames(['Julie'], 'friendObj');
});
searchAny
변수를 사용하여 사용자가 문자열 또는 숫자를 사용하여 필터링할 수 있음을 나타냅니다.
searchName
변수를 선언한 후 strict
변수도 선언하여 숫자에 대한 입력 필드에서 문자열로 필터링하는 위치에 데이터가 표시되지 않도록 합니다. 문자열에 대한 입력 필드의 숫자로 필터링을 시도할 때도 마찬가지입니다.
아래 출력을 참조하십시오.
Angular에서 파이프를 사용하여 객체 속성으로 필터링
파이프는 두 개의 명령 또는 프로세스를 실행하는 데 이상적이며 여기서 수행하는 작업에 이상적입니다. 예를 들어, 하나의 프로세스인 필터링을 원하고 두 번째 프로세스인 필터의 결과를 반환하도록 합니다.
따라서 우리는 새로운 Angular 프로젝트를 생성하고 싶고 필터링하려는 항목의 세부 정보를 저장할 새 파일을 만들고 싶습니다. 파일 이름을 cars.ts
로 지정하고 다음 코드를 입력합니다.
코드 스니펫 - cars.ts
:
export const CARS = [
{
brand: 'Audi',
model: 'A4',
year: 2018
}, {
brand: 'Audi',
model: 'A5 Sportback',
year: 2021
}, {
brand: 'BMW',
model: '3 Series',
year: 2015
}, {
brand: 'BMW',
model: '4 Series',
year: 2017
}, {
brand: 'Mercedes-Benz',
model: 'C Klasse',
year: 2016
}
];
다음으로 필터에 대한 입력 필드를 포함하여 cars.ts
의 데이터를 테이블로 구성할 파일 구조를 생성하려고 합니다. app.component.html
파일 내부에 해당 코드를 입력합니다.
코드 스니펫 - app.component.html
<div class="container">
<h2 class="py-4">Cars</h2>
<div class="form-group mb-4">
<input class="form-control" type="text" [(ngModel)]="searchText" placeholder="Search">
</div>
<table class="table" *ngIf="(cars | filter: searchText).length > 0; else noResults">
<colgroup>
<col width="5%">
<col width="*">
<col width="35%">
<col width="15%">
</colgroup>
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">Brand</th>
<th scope="col">Model</th>
<th scope="col">Year</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let car of cars | filter: searchText; let i = index">
<th scope="row">{{i + 1}}</th>
<td>{{car.brand}}</td>
<td>{{car.model}}</td>
<td>{{car.year}}</td>
</tr>
</tbody>
</table>
<ng-template #noResults>
<p>No results found for "{{searchText}}".</p>
</ng-template>
</div>
이제 우리는 앱의 기능에 대해 작업하려고 합니다. 새 파일을 만들고 filter.pipe.ts
라는 이름을 지정하고 다음 코드를 포함합니다.
코드 스니펫 - filter.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'filter'
})
export class FilterPipe implements PipeTransform {
transform(items: any[], searchText: string): any[] {
if (!items) return [];
if (!searchText) return items;
return items.filter(item => {
return Object.keys(item).some(key => {
return String(item[key]).toLowerCase().includes(searchText.toLowerCase());
});
});
}
}
Angular 코어에서 Pipe
및 PipeTransform
을 가져왔으므로 항목을 필터링할 때 데이터를 변환하고 필터링된 결과를 반환합니다. 다음으로 app.component.ts
파일에 액세스하여 추가 기능을 위한 코드를 추가해야 합니다.
코드 스니펫 - app.component.ts
:
import { Component } from '@angular/core';
import { CARS } from './cars';
interface Car {
brand: string;
model: string;
year: number;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'filthree';
cars: Car[] = CARS;
searchText: string;
}
cars.ts
배열의 항목에 대한 데이터 유형을 선언했습니다. 그런 다음 마지막으로 필요한 모듈을 app.module.ts
파일로 가져와야 합니다.
코드 스니펫 - app.module.ts
:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { FilterPipe } from './filter.pipe';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, FilterPipe ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
모든 단계를 완료하면 다음 출력이 표시됩니다.
따라서 Angular 필터로 항목을 정렬하는 것은 원하는 항목을 빠르게 검색하는 데 도움이 되므로 웹 사이트 사용자가 편리하게 사용할 수 있는 기능 중 하나입니다.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn