TypeScript를 사용하여 Angular에서 getElementById 교체
-
TypeScript의
document.getElementById()
메서드 -
TypeScript를 사용하여 Angular에서
ElementRef
를getElementById
대체로 사용
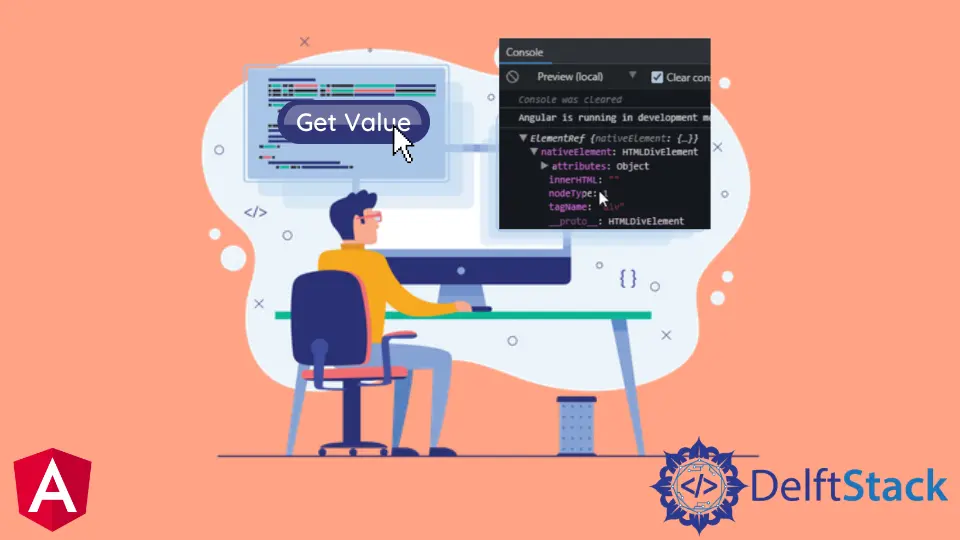
이 튜토리얼 가이드라인은 TypeScript를 사용하여 Angular에서 document.getElementById
대체에 대한 간략한 설명을 제공합니다.
이것은 또한 코드 예제와 함께 Angular에서 getElementById
에 사용되는 최상의 방법을 제공합니다. 또한 TypeScript에서 DOM querySelector의 목적을 배웁니다.
먼저 TypeScript의 document.getElementById
메소드와 이것이 개발자 커뮤니티에서 인기 있는 이유에 대해 알아보겠습니다.
TypeScript의 document.getElementById()
메서드
document.getElementById()
는 document
개체의 조작에 사용할 수 있는 미리 정의된 JavaScript(TypeScript와 마찬가지로) 메서드입니다. 지정된 값을 가진 요소를 반환하고 요소가 존재하지 않으면 null
을 반환합니다.
document.getElementById()
는 HTML DOM에 대한 개발자 커뮤니티에서 가장 일반적이고 인기 있는 방법 중 하나입니다.
명심해야 할 한 가지 중요한 점은 둘 이상의 요소가 동일한 ID를 갖는 경우 document.getElementById()
가 첫 번째 요소를 반환한다는 것입니다.
이제 목적과 사용법을 더 잘 이해하기 위해 몇 가지 코딩 예제를 살펴보겠습니다.
고유한 demo
ID를 가진 일부 텍스트가 있는 h1
태그를 고려하십시오. 이제 scripts
섹션에서 특정 태그를 대상으로 지정하려면 document
개체에서 사용할 수 있는 getElementById()
메서드를 사용합니다.
<!DOCTYPE html>
<html>
<body>
<h1 id="demo">The Document Object</h1>
<h2>The getElementById() Method</h2>
<script>
document.getElementById("demo").style.color = "red";
</script>
</body>
</html>
출력:
이제 동적 예를 하나 더 생각해 보겠습니다. 입력 필드는 숫자를 받아 큐브 값을 반환합니다.
<!DOCTYPE html>
<html>
<body>
<form>
Enter No:<input type="text" id="number" name="number"/><br/>
<input type="button" value="cube" onclick="getcube()"/>
</form>
<script>
function getcube(){
var number=document.getElementById("number").value;
alert(number*number*number);
}
</script>
</body>
</html>
출력:
이제 TypeScript를 사용하여 Angular에서 getElementById
를 대체하는 방법을 살펴보겠습니다.
TypeScript를 사용하여 Angular에서 ElementRef
를 getElementById
대체로 사용
AngularJs의 ElementRef
는 nativeElement
속성을 포함하고 기본 DOM 개체에 대한 참조를 보유하는 HTML 요소를 둘러싼 래퍼입니다. ElementRef
를 사용하면 TypeScript를 사용하여 AngularJ에서 DOM을 조작할 수 있습니다.
ViewChild
를 사용하여 컴포넌트 클래스 내 HTML 요소의 ElementRef
를 얻습니다. Angular는 구성 요소가 생성자에서 요청될 때 구성 요소의 지시문 요소의 ElementRef
를 주입할 수 있습니다.
@ViewChild
데코레이터를 사용하여 클래스 내부의 ElementRef
인터페이스를 사용하여 요소 참조를 가져옵니다.
main.component.html
파일의 AngularJs
에 있는 다음 코드 예제를 고려하십시오. 이벤트가 있는 버튼과 고유 ID가 myName
인 div가 있습니다.
# angular
<div #myName></div>
<button (click)="getData()">Get Value</button>
출력:
예 1(app.component.ts
):
#angular
import { Component, VERSION, ViewChild, ElementRef } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./main.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
name = "Angular " + VERSION.major;
@ViewChild("myName") myName: ElementRef;
getData() {
console.log(this.myName);
this.myName.nativeElement.innerHTML = "I am changed by ElementRef & ViewChild";
}
}
출력:
@ViewChild
데코레이터를 사용하여 클래스 내부의 ElementRef
인터페이스를 통해 입력 참조를 얻습니다. 그런 다음 이 참조와 함께 getData()
함수를 사용하여 값을 변경합니다.
예 2(app.component.ts
):
import { Component, ViewChild, ElementRef, AfterViewInit } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent implements AfterViewInit {
name = "Angular";
@ViewChild("ipt", { static: true }) input: ElementRef;
ngAfterViewInit() {
console.log(this.input.nativeElement.value);
}
onClick() {
this.input.nativeElement.value = "test!";
}
}
출력:
이것은 입력 필드의 참조를 가져오고 버튼 클릭 시 내부 텍스트를 변경합니다.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn