AngularJS에서 간단한 테이블 만들기
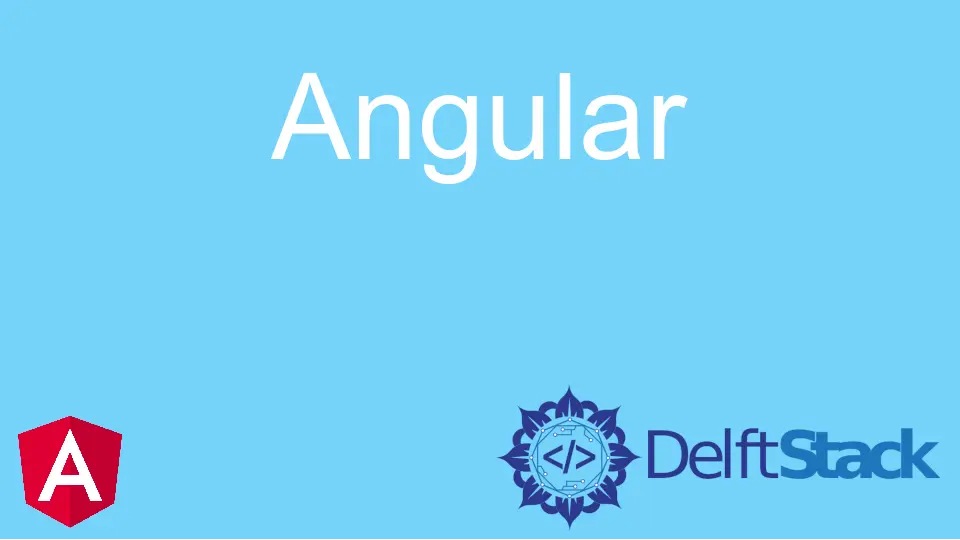
전자 상거래 웹 사이트에서 웹 페이지를 만들 때 제품 목록 및 가격, 직원 목록, 급여, 고용 날짜 등과 같은 크고 복잡한 정보를 표시하기 위해 테이블을 사용해야 합니다.
표는 이러한 데이터를 잘 구조화되고 소화하기 쉬운 방식으로 제시하는 데 도움이 됩니다. 또한 웹 사이트의 화면 자산을 더 잘 관리할 수 있습니다.
따라서 *ngFor
함수를 사용하여 간단한 테이블을 만든 다음 데이터베이스를 통해 전달합니다. JSON Server Rest API
를 사용할 것입니다.
먼저 VS Code 편집기를 사용하여 내부에서 터미널을 사용하여 종속성을 설치할 수 있습니다. 그런 다음 터미널을 열고 npm install -g json-server
를 입력하고 json server
를 시스템에 설치합니다.
다음 단계는 새 프로젝트를 만드는 것입니다.
프로젝트가 생성되면 프로젝트 폴더를 열고 db.json
이라는 새 파일을 추가합니다. 여기에 REST API
가 액세스할 데이터를 저장합니다.
이제 db.json
폴더로 이동하여 테이블에 갖고 싶은 데이터를 입력합니다.
{
"Users": [
{
"id": 1,
"firstName": "Nitin",
"lastName": "Rana",
"email": "nitin.rana@gmail.com",
"mobile": "2345678901",
"salary": "25000"
},
{
"id": 2,
"firstName": "Rajat",
"lastName": "Singh",
"email": "rajat.singh1@gmail.com",
"mobile": "5637189302",
"salary": "30000"
},
{
"id": 3,
"firstName": "Rahul",
"lastName": "Singh",
"email": "rahul.singh1@gmail.com",
"mobile": "5557189302",
"salary": "40000"
},
{
"id": 4,
"firstName": "Akhil",
"lastName": "Verma",
"email": "akhil.verma2@gmail.com",
"mobile": "5690889302",
"salary": "20000"
},
{
"id": 5,
"firstName": "Mohan",
"lastName": "Ram",
"email": "mohan.ram1@gmail.com",
"mobile": "7637189302",
"salary": "60000"
},
{
"id": 6,
"firstName": "Sohan",
"lastName": "Rana",
"email": "sohan.rana@gmail.com",
"mobile": "3425167890",
"salary": "25000"
},
{
"id": 7,
"firstName": "Rajjev",
"lastName": "Singh",
"email": "rajeev.singh1@gmail.com",
"mobile": "5637189302",
"salary": "30000"
},
{
"id": 8,
"firstName": "Mukul",
"lastName": "Singh",
"email": "mukul.singh1@gmail.com",
"mobile": "5557189302",
"salary": "40000"
},
{
"id": 9,
"firstName": "Vivek",
"lastName": "Verma",
"email": "vivek.verma2@gmail.com",
"mobile": "5690889302",
"salary": "20000"
},
{
"id": 10,
"firstName": "Shubham",
"lastName": "Singh",
"email": "shubham.singh@gmail.com",
"mobile": "7637189502",
"salary": "60000"
}
]
}
다음으로 할 일은 app.component.html
을 탐색하여 *ngFor
기능을 사용하여 테이블을 생성하는 것입니다. 그러나 그 전에 db.json
의 데이터에 액세스할 Rest 서비스
를 만들어야 합니다.
그런 다음 터미널로 이동합니다. 프로젝트 폴더에 ng generate service Rest
를 입력합니다.
설치 후 scr/app
폴더에 rest.service.spec.ts
및 rest.service.ts
라는 두 개의 파일이 생성됩니다. 이제 코딩을 하기 위해 rest.service.ts
로 이동해야 합니다.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Users } from './Users';
@Injectable({
providedIn: 'root'
})
export class RestService {
constructor(private http : HttpClient) { }
url : string = "http://localhost:3000/Users";
getUsers()
{
return this.http.get<Users[]>(this.url);
}
}
이 서비스는 데이터베이스가 통과할 원하는 URL의 활동을 모니터링하는 데 도움이 됩니다.
그런 다음 app.component.html
로 이동하여 *ngFor
함수를 사용하여 테이블을 생성합니다.
<h1>Table using JSON Server API</h1>
<hr>
<table id="users">
<tr>
<th *ngFor="let col of columns">
{{col}}
</th>
</tr>
<tr *ngFor="let user of users">
<td *ngFor="let col of index">
{{user[col]}}
</td>
</tr>
</table>
다음으로 해야 할 일은 app.module.ts
로 이동하여 더 많은 코딩을 수행하는 것입니다. 여기에서 가져오기 배열에 HttpClientModule
을 추가합니다.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
db.json
의 테이블 날짜를 HttpClient
에서 읽을 수 있도록 하려면 문자열 속성으로 만들어야 합니다. 따라서 Users.ts
파일을 만들고 다음 코드를 입력합니다.
export class Users
{
id : string;
firstName : string;
lastName : string;
email : string;
mobile : string;
salary : string;
constructor(id, firstName, lastName, email, mobile, salary)
{
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.mobile = mobile;
this.salary = salary;
}
}
이 시점에서 해야 할 유일한 일은 CSS 스타일을 사용하여 테이블을 아름답게 하는 것입니다.
h1
{
text-align: center;
color: #4CAF50;
}
#users {
font-family: "Trebuchet MS", Arial, Helvetica, sans-serif;
border-collapse: collapse;
width: 100%;
}
#users td, #users th {
border: 1px solid #ddd;
padding: 8px;
}
#users tr:nth-child(even){background-color: #f2f2f2;}
#users tr:hover {background-color: #ddd;}
#users th {
padding-top: 12px;
padding-bottom: 12px;
text-align: left;
background-color: #4CAF50;
color: white;
}
이제 모든 것이 잘 작동하지만 Parameter mobile
에 암시적으로 any
유형이 있는 것과 같은 오류가 발생할 수 있습니다.
ts.config.json
파일로 이동하여 noImplicitAny: false
를 목록에 추가해야 합니다. 그런 다음 noImplicitOverride: true,
에 \\
를 추가하여 설정을 비활성화합니다.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn