Pandas DataFrame DataFrame.set_index() 함수
Suraj Joshi
2023년1월30일
-
pandas.DataFrame.set_index()
메서드 구문: -
예제 코드: Pandas
DataFrame.set_index()
메서드로 Pandas DataFrame 인덱스 설정 -
예제 코드: Pandas
DataFrame.set_index()
메서드에서drop = False
설정 -
예제 코드: Pandas
DataFrame.set_index
메서드에서inplace = True
설정 -
예제 코드: Pandas
DataFrame.set_index()
메서드를 사용하여 여러 인덱스 열 설정 -
예제 코드:
verify_integrity
가True
일 때 PandasDataFrame.set_index()
동작
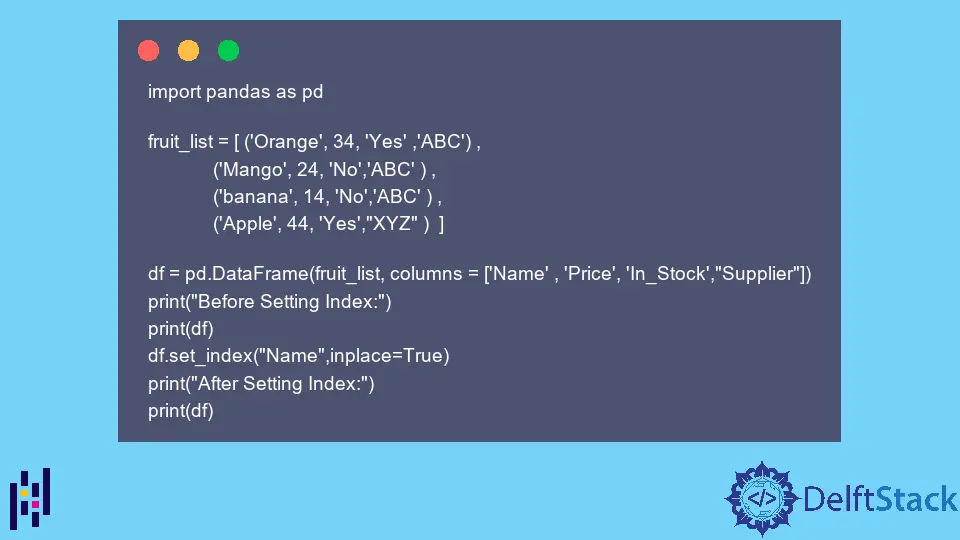
pandas.DataFrame.set_index()
메서드를 사용하여 배열 또는 열을 설정할 수 있습니다. DataFrame 생성 후에도 DataFrame의 인덱스로 적절한 길이의 새로 설정된 인덱스는 기존 인덱스를 대체하거나 기존 인덱스에서 확장 할 수도 있습니다.
pandas.DataFrame.set_index()
메서드 구문:
DataFrame.set_index(
keys, drop=True, append=False, inplace=False, verify_integrity=False
)
매개 변수
keys |
인덱스로 설정할 열 또는 열 목록 |
drop |
부울. 기본값은 True 로 인덱스로 설정할 컬럼을 삭제합니다. |
append |
부울. 기본값은 False 이며 기존 색인에 열을 추가할지 여부를 지정합니다. |
inplace |
부울. True 이면 호출자 DataFrame을 제자리에서 수정합니다. |
verify_integrity |
부울. True 인 경우 중복 색인을 만들 때 ValueError 를 발생시킵니다. 기본값은 False 입니다. |
반환
inplace
가True
이면 인덱스 열이 수정 된DataFrame
객체를 반환합니다. 그렇지 않으면 None
.
예제 코드: Pandas DataFrame.set_index()
메서드로 Pandas DataFrame 인덱스 설정
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print(df)
df_modified=df.set_index("Name")
print(df_modified)
출력:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
4 Pineapple 64 No XYZ
5 Kiwi 84 Yes XYZ
Price In_Stock Supplier
Name
Orange 34 Yes ABC
Mango 24 No ABC
banana 14 No ABC
Apple 44 Yes XYZ
Pineapple 64 No XYZ
Kiwi 84 Yes XYZ
원본Dataframe
은 기본 인덱스 열로 숫자 범위를 가지며modified_df
에서는set_index()
메서드를 사용하여Name
열을 인덱스로 설정합니다.
예제 코드: Pandas DataFrame.set_index()
메서드에서drop = False
설정
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list,
columns = ['Name',
'Price',
'In_Stock',
'Supplier'])
print(df)
df_modified=df.set_index("Name",drop=False)
print(df_modified)
출력:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
Name Price In_Stock Supplier
Name
Orange Orange 34 Yes ABC
Mango Mango 24 No ABC
banana banana 14 No ABC
Apple Apple 44 Yes XYZ
DataFrame set_index
메소드에서drop = False
를 설정하면name
열은index
열로 설정 한 후에도Dataframe
에서 열로 남아 있습니다.
예제 코드: Pandas DataFrame.set_index
메서드에서inplace = True
설정
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list, columns = ['Name' , 'Price', 'In_Stock',"Supplier"])
print("Before Setting Index:")
print(df)
df.set_index("Name",inplace=True)
print("After Setting Index:")
print(df)
출력:
Before Setting Index:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
After Setting Index:
Price In_Stock Supplier
Name
Orange 34 Yes ABC
Mango 24 No ABC
banana 14 No ABC
Apple 44 Yes XYZ
set_index()
메서드에서inplace = True
를 설정하면 호출자dataFrame
이 제자리에서 수정됩니다.
예제 코드: Pandas DataFrame.set_index()
메서드를 사용하여 여러 인덱스 열 설정
import pandas as pd
fruit_list = [ ('Orange', 34, 'Yes' ,'ABC') ,
('Mango', 24, 'No','ABC' ) ,
('banana', 14, 'No','ABC' ) ,
('Apple', 44, 'Yes',"XYZ" ) ]
df = pd.DataFrame(fruit_list, columns = ['Name' , 'Price', 'In_Stock',"Supplier"])
print("Before Setting Index:")
print(df)
df.set_index("Name",append=True,inplace=True,drop=False)
print("After Setting Index:")
print(df)
출력:
Before Setting Index:
Name Price In_Stock Supplier
0 Orange 34 Yes ABC
1 Mango 24 No ABC
2 banana 14 No ABC
3 Apple 44 Yes XYZ
After Setting Index:
Name Price In_Stock Supplier
Name
0 Orange Orange 34 Yes ABC
1 Mango Mango 24 No ABC
2 banana banana 14 No ABC
3 Apple Apple 44 Yes XYZ
set_index
메소드에서 append = True
를 설정하면 새로 설정된 색인 열을 기존 색인에 추가하고 단일 DataFrame
에 대해 여러 색인 열을 갖습니다.
예제 코드: verify_integrity
가True
일 때 Pandas DataFrame.set_index()
동작
import pandas as pd
fruit_list = [
("Orange", 34, "Yes", "ABC"),
("Mango", 24, "No", "ABC"),
("Apple", 14, "No", "ABC"),
("Apple", 44, "Yes", "XYZ"),
]
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "In_Stock", "Supplier"])
df_modified = df.set_index("Name", verify_integrity=True)
print(df_modified)
출력:
Traceback (most recent call last):
.....line 3920, in set_index
dup=duplicates))
ValueError: Index has duplicate keys: Index(['Apple'], dtype='object', name='Name')
인덱스에 Apple
이라는 중복 키가 있으므로 ValueError
가 발생합니다. 인덱스로 설정된 열에 두 개의 Apple
이 있습니다. 따라서set_index()
메소드에서verify_integrity
가True
로 설정되면 오류가 발생합니다.
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn