TypeScript でクラス定数を実装する
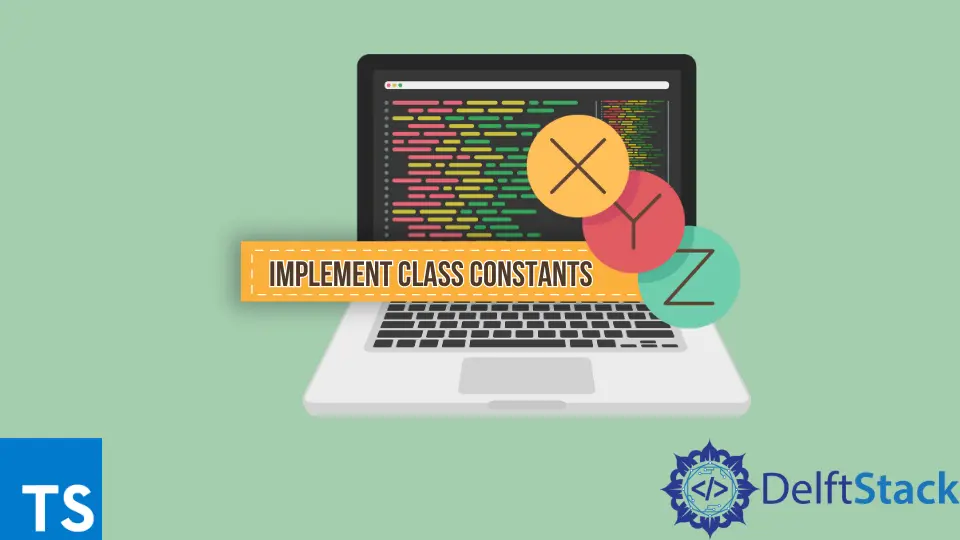
この記事では、readonly
キーワードと readonly
キーワードを組み合わせた static
キーワードを使用してクラス定数を実装する方法を説明します。
TypeScript の定数
プログラミング言語の定数は、一度割り当てられると値を変更できない固定値です。 クラス定数は、一度割り当てられると変更できないクラス内の定数フィールドです。
TypeScript では、クラスの作成は単純なタスクです。 以下は、TypeScript でクラスを作成する方法の構文です。
class className {
// Block of code
}
上記は、TypeScript でクラスを作成する簡単な方法です。 いくつかの方法に従って、変更または変更できない値を持つ定数フィールドまたはプロパティを持つクラスを作成できます。
TypeScript では、クラス メンバーが const
キーワードを持つことができないことを覚えておくことが不可欠です。 クラス メンバーの定数のインライン宣言は、TypeScript では不可能です。
そうしようとすると、コンパイラはコンパイル エラーをスローします。 クラスを定数にするために、次のセクションで説明する TypeScript で使用可能ないくつかのオプションを実装できます。
TypeScript で readonly
を使用してクラス定数を実装する
クラス プロパティまたはフィールドを変更不可にするクラスを作成した後、TypeScript 2.0 で導入された readonly
キーワードを使用して、クラス フィールドまたはプロパティを変更不可として定義できます。 プロパティを変更しようとすると、エラー メッセージが表示されます。
例:
class DisplayOutput{
readonly name : string = "Only using readonly";
displayOutput() : void{
console.log(this.name);
}
}
let instance = new DisplayOutput();
instance.displayOutput();
出力:
Only using readonly
readonly
のみを使用すると、定数として完全には機能しません。 これは、コンストラクターで代入が許可されているためです。
TypeScript で readonly
と static
を使用してクラス定数を実装する
クラス フィールドまたはプロパティを変更不可にするために使用できる別の方法は、static
キーワードを使用した readonly
キーワードです。 readonly
を使用する場合と比較した場合の利点は、コンストラクターで割り当てが許可されないため、1つの場所にしか割り当てられないことです。
例:
class DisplayOutput{
static readonly username : string = "Using both static and readonly keywords";
static displayOutput() : void {
console.log(DisplayOutput.username);
}
}
DisplayOutput.displayOutput();
出力:
Using both static and readonly keywords
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.