Scala の可変リスト
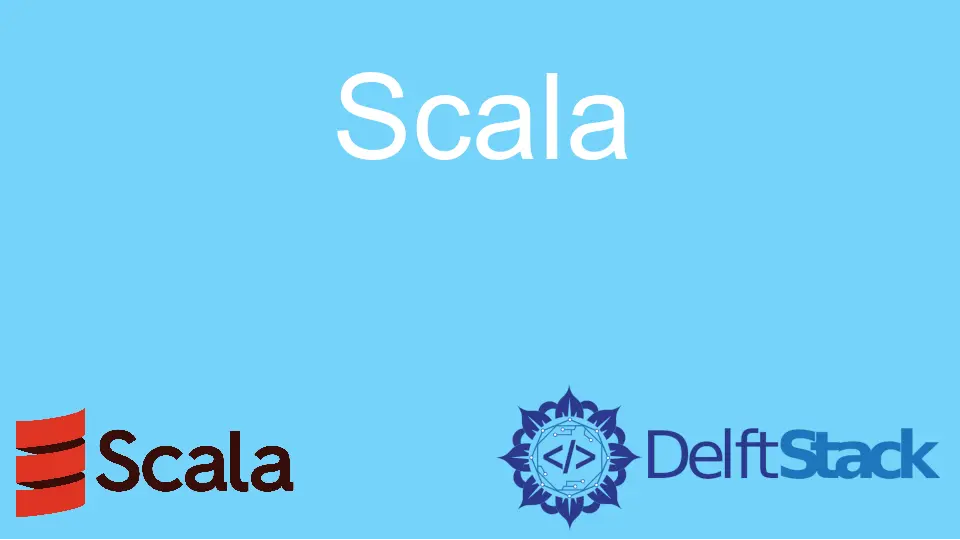
この記事では、Scala の可変リストについて学びます。 デフォルトでは、Scala のリストは不変であるため、一度初期化すると更新できませんが、Scala は多くの可変リストを提供します。
それらを1つずつ見てみましょう。
Scala の DoubleLinkedList
Scala の DoubleLinkedList
は、前のノードまたは次のノードに移動するための prev
と next
の 2つの参照を持つリンクされたリストです。 2つの参照があると、リストを行き来することができます。
例を見てみましょう。
object MyClass {
class Node(var data: Int,var prev: Node, var next: Node)
{
def this(data: Int)
{
this(data, null, null)
}
}
class DoublyLinkedList(var head:Node,var tail: Node)
{
def this()
{
this(null, null)
}
def insert(x: Int): Unit = {
var node:Node = new Node(x);
if (this.head == null)
{
this.head = node;
this.tail = node;
return;
}
this.tail.next = node;
node.prev = this.tail;
this.tail = node;
}
def show(): Unit = {
if (this.head == null)
{
println("List is empty");
}
else
{
print("\nPrinting list from front to back :\n");
var first: Node = this.head;
while (first!= null)
{
print(" " + first.data);
first = first.next;
}
print("\nPrinting list from back to front:\n");
var last: Node = this.tail;
while (last!= null)
{
print(" " + last.data);
last = last.prev;
}
}
}
}
def main(args: Array[String])
{
var dll: DoublyLinkedList = new DoublyLinkedList();
dll.insert(100);
dll.insert(200);
dll.insert(300);
dll.insert(400);
dll.show(); //to print the list
}
}
出力:
Printing list from front to back :
100 200 300 400
Printing list from back to front:
400 300 200 100
Scala のLinkedList
Scala の LinkedList
は単一のリンク リストを作成するために使用されるため、ここには prev
ポインタはありません。 一方向のみの次のノードにトラバースしたい場合に使用します。
ここで、リンクを作成し、必要に応じて変更できます。
コード例:
case class LinkedList[obj]()
{
var head: node[obj] = null;
def insert(myData: obj) = {
head match {
case null => head = new node(myData,null)
case _ =>
{
var end:node[obj] = head;
while (end.next!= null) {
end = end.next;
}
end.next = new node[obj](myData, null);
}
}
}
def display()
{
if (head != null) {
head.displayLinkedList();
}
println();
}
}
sealed case class node[obj](var data: obj, var next: node[obj])
{
def myData: obj = this.data
def nextPtr: node[obj] = this.next;
def displayLinkedList(): Unit = {
print(data)
if (next!= null) {
print(" -> ")
next.displayLinkedList();
}
}
}
object Main extends App {
var list: LinkedList[Int] = new LinkedList();
list.insert(100);
list.insert(200);
list.insert(300);
list.display();
}
出力:
100 -> 200 -> 300
DoubleLinkedList
と LinkedList
は、mutable.Queues
や mutable.lists
などのより複雑なリスト構造を作成するために使用されます。
ScalaのListBuffer
ListBuffer は、名前が示すように、リストに基づく Buffer 実装です。 これを使用する主な利点は、ほとんどの線形時間操作を実行できることです。
append や prepend などの操作。 したがって、変更可能にしたい場合にリストを実装するために広く使用されています。
リストは不変であるため、頻繁に変化し続けるリストが必要な場合は、ListBuffer
を使用します。 ListBuffer
を使用するには、scala.collection.mutable.ListBuffer
をプログラムにインポートする必要があります。
構文:
var one = new ListBuffer[data_type]() //this creates empty buffer
OR
var two = new ListBuffer("apple","orange","watermelon")
ListBuffer
で実行される一般的な操作の一部は次のとおりです。
LB+=element
を使用して一定時間内に要素を追加できます。LB+=:Buffer
を使用して、要素を一定時間前に追加できます。- リストの処理が完了したら、
LB.toList
を使用してListBuffer
(可変) をList
(不変) に一定時間で変換できます。
コード例:
import scala.collection.mutable.ListBuffer
object MyClass {
def main(args: Array[String]) {
var lb = ListBuffer[String]()
lb+= "Apple";
lb+= "Orange";
"dragonFruit"+=:lb //prepending
println(lb);
var result: List[String] = lb.toList
println(result)
}
}
出力:
ListBuffer(dragonFruit, Apple, Orange)
List(dragonFruit, Apple, Orange)