React-Table 固定カラム
-
react-table
バージョン > 7 にはreact-table-sticky
を使用する -
react-table
バージョン < 7 にはreact-table-hoc-fixed-columns
を使用する
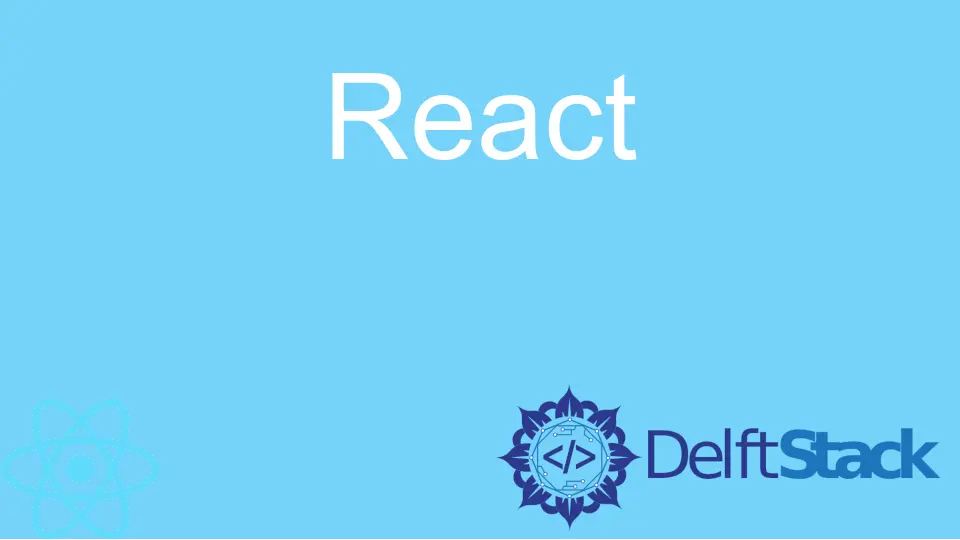
この記事では、react-table
コンポーネントの固定列を作成する方法を説明します。 場合によっては、左または右の位置に固定列を作成し、他の列をスクロール可能にする必要があります。
この記事では、固定列を作成する 2つの異なる方法について説明します。
react-table
バージョン > 7 には react-table-sticky
を使用する
react-table
ライブラリ バージョン > 7 を使用する場合は、react-table-sticky
ライブラリを使用してスティッキー カラムを作成する必要があります。 react-table-sticky
依存関係をインストールするには、プロジェクト ディレクトリで以下のコマンドを実行します。
npm i react-table-sticky
スティッキー列を作成するには、以下に示すように、テーブルの作成中に useSticky
を使用し、列データの作成中に sticky
キーを渡す必要があります。
// creating a table
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } =
useTable({columns, data,},useBlockLayout,
// use useSticky here
useSticky
);
// column data
const columns = [
{
Header: "City Info",
sticky: "left",
columns: [
{
Header: "Name",
accessor: "Name",
width: 150,
},
],
{
//Other groups of columns
},
},
]
以下の例では、さまざまな都市のデータを作成し、列とデータを Table
コンポーネントの prop として渡しています。 また、sticky: left
キーと値のペアを City Info
グループの列に追加しました。
table
コンポーネントでは、データを繰り返し処理してデータを作成し、表示しました。
コード例:
import React from "react";
import styled from "styled-components";
import { useTable, useBlockLayout } from "react-table";
import { useSticky } from "react-table-sticky";
// style for the table
const Styles = styled.div`
padding: 1rem;
.table {
border: 2px dotted #ddd;
.th,
.td {
border-bottom: 1px dotted #ddd;
padding: 5px;
background-color: #fff;
border-right: 1px dotted #ddd;
}
&.sticky {
overflow: scroll;
}
}
`;
function Table({ columns, data }) {
const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } =
useTable(
{
columns,
data,
},
useBlockLayout,
// use useSticky here
useSticky
);
return (
<Styles>
{/* setup table header */}
<div {...getTableProps()} className="table sticky">
<div className="header">
{headerGroups.map((hdrGroup) => (
<div {...hdrGroup.getHeaderGroupProps()} className="tr">
{hdrGroup.headers.map((clmn) => (
<div {...clmn.getHeaderProps()} className="th">
{clmn.render("Header")}
</div>
))}
</div>
))}
</div>
{/* setup table body */}
<div {...getTableBodyProps()}>
{rows.map((singleRow) => {
prepareRow(singleRow);
return (
<div {...singleRow.getRowProps()} className="tr">
{singleRow.cells.map((singleCell) => {
return (
<div {...singleCell.getCellProps()} className="td">
{singleCell.render("Cell")}
</div>
);
})}
</div>
);
})}
</div>
</div>
</Styles>
);
}
function App() {
// data of the different cities to show in the table
const data = [
{
Name: "Rajkot",
population: 3000000,
isClean: "Yes",
totalCars: 40000,
Bikes: 60000,
},
{
Name: "Gondal",
population: 3400000,
isClean: "Yes",
totalCars: 45000,
Bikes: 40000,
},
{
Name: "Surat",
population: 45000000,
isClean: "Yes",
totalCars: 23000,
Bikes: 32000,
},
{
Name: "Vadodara",
population: 560000,
isClean: "No",
totalCars: 870000,
Bikes: 100000,
},
{
Name: "Jetpur",
population: 20000,
isClean: "Yes",
totalCars: 10000,
Bikes: 30000,
},
{
Name: "Valsad",
population: 700000,
isClean: "No",
totalCars: 8000,
Bikes: 45000,
},
];
// table columns
const columns = [
{
Header: "City Info",
sticky: "left",
columns: [
{
Header: "Name",
accessor: "Name",
width: 150,
},
],
},
{
Header: "Details",
columns: [
{
Header: "Population",
accessor: "population",
width: 200,
},
{
Header: "Is Clean?",
accessor: "isClean",
width: 200,
},
{
Header: "Total Cars",
accessor: "totalCars",
width: 200,
},
{
Header: "Total Bikes",
accessor: "Bikes",
width: 200,
},
],
},
];
return <Table columns={columns} data={data} />;
}
export default App;
出力:
上記の出力では、ブラウザーのサイズが小さくなり、テーブルの列が水平方向にスクロール可能になると、Name
列は左側に固定されたままになり、他の列はスクロール可能になることがわかります。
react-table
バージョン < 7 には react-table-hoc-fixed-columns
を使用する
開発者が react-table
バージョン < 7 を使用している場合、react-table-hoc-fixed-columns
ライブラリも使用できます。 react-sticky-table
ライブラリよりも使いやすいです。
現在の React プロジェクトに react-table-hoc-fixed-columns
依存関係をインストールするには、ターミナルで以下のコマンドを実行する必要があります。
npm i react-table-hoc-fixed-columns
その後、react-table-hoc-fixed-columns
からインポートされた withFixedColumns
でテーブルを作成する必要があります。 また、ユーザーは fixed: position
キーと値のペアを列グループに追加する必要があります。
const ReactFixedColumnsTable = withFixedColumns(ReactTable);
const columns = [
{
Header: "Name Info",
fixed: "Right",
columns: [
{
Header: "First Name",
accessor: "firstName",
width: 150,
},
],
{
//Other groups of columns
},
},
]
以下の例で何人かの通常のデータを作成し、それを使用して react-table
でテーブルを作成しました。 また、fixed: "left"
キーと値のペアを列配列の最初のグループに渡し、最初の 2 列を左側に固定しました。
コード例:
// import required libraries
import React from "react";
import { render } from "react-dom";
import ReactTable from "react-table";
import "react-table/react-table.css";
// Import React Table HOC Fixed columns
import withFixedColumns from "react-table-hoc-fixed-columns";
import "react-table-hoc-fixed-columns/lib/styles.css";
const ReactFixedColumnsTable = withFixedColumns(ReactTable);
function App() {
// Creating the data for the table
const tableData = [
{
firstName: "Shubham",
lastName: "Vora",
Age: 21,
Above18: "Yes",
Gender: "Male",
City: "Rajkot",
},
{
firstName: "Akshay",
lastName: "Kumar",
Age: 32,
Above18: "Yes",
Gender: "Male",
City: "Ahmedabad",
},
{
firstName: "Jems",
lastName: "trum",
Age: 13,
Above18: "No",
Gender: "Male",
City: "Delft",
},
{
firstName: "Nora",
lastName: "Fatehi",
Age: 45,
Above18: "Yes",
Gender: "Female",
City: "Mumbai",
},
{
firstName: "Malaika",
lastName: "Arora",
Age: 47,
Above18: "Yes",
Gender: "female",
City: "Delhi",
},
];
const tableColumns = [
{
Header: "Name Info",
// adding fixed property in the object
fixed: "left",
columns: [
{
Header: "First Name",
accessor: "firstName",
width: 150,
},
{
Header: "Last Name",
accessor: "lastName",
width: 150,
},
],
},
{
Header: "Details",
columns: [
{
Header: "Age",
accessor: "Age",
width: 200,
},
{
Header: "Above 18?",
accessor: "Above18",
width: 200,
},
{
Header: "Gender",
accessor: "Gender",
width: 200,
},
{
Header: "City",
accessor: "City",
width: 200,
},
],
},
];
return (
<div>
{/* calling the table component */}
<ReactFixedColumnsTable
data={tableData}
columns={tableColumns}
Defa
className="-striped"
/>
<br />
</div>
);
}
export default App;
出力:
そのため、react-table
ライブラリを使用している間、列を固定またはスティッキーにするデフォルトの方法はありません。 ユーザーは常に他のサードパーティ ライブラリを使用する必要があります。
上記で説明したように、ユーザーは、使用している react-table
のバージョンに応じて、任意の React ライブラリを使用できます。
ユーザーがサードパーティ ライブラリを使用してテーブルの列をスティッキーにしたくない場合は、CSS をカスタマイズして同じことを実現できます。