React 要素の幅を取得する
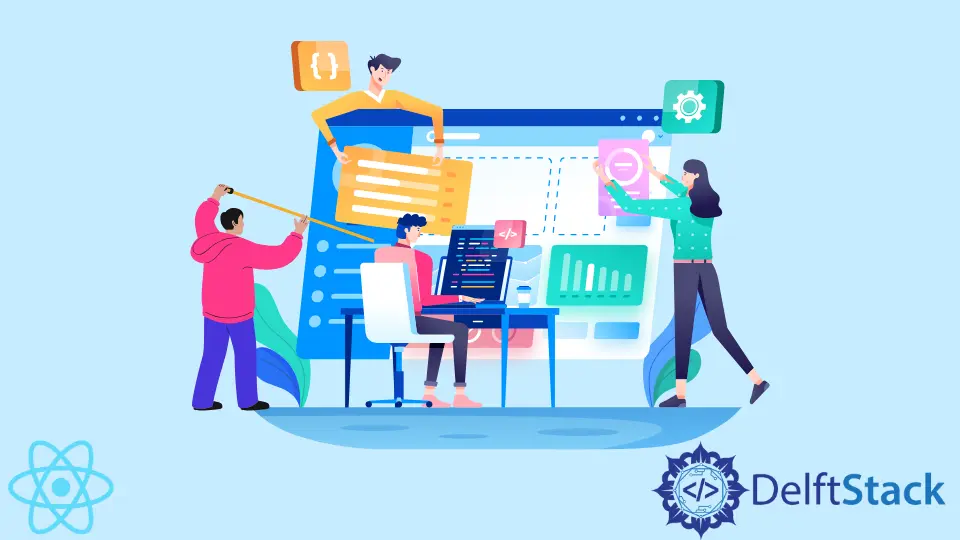
この記事では、React で要素の幅を取得する方法を学習します。 多くの場合、要素の幅を取得して、幅またはその他の寸法に従って操作を実行する必要があります。 したがって、ここでは useRef()
React フックを使用して、特定の要素のサイズを取得します。
useRef()
React フックを使用して要素の幅を取得する
React で useRef()
の働きをゼロから理解してみましょう。
まず、標準の JavaScript で要素の幅を取得する必要があるかどうかを検討してください。 職業はなんですか? 答えは、まず DOM 要素にアクセスし、その DOM 要素の width
プロパティを取得することです。
ここでは、useRef()
を使用して、React DOM 要素への参照を作成します。 ユーザーは useRef()
フックを使用して、以下のような DOM 要素の参照を取得できます。
const ref = useRef(Initial DOM element);
return (
<div ref={ref} >
</div>
);
useRef()
フックには current
プロパティが含まれており、それを使用して参照先の要素にアクセスできます。 参照要素を取得したら、offsetWidth
を使用して特定の参照要素の幅を取得できます。
let width = ref.current.offsetWidth;
以下の例では、HTML の <div>
要素を作成し、ref
変数を使用して参照しています。 最初に、div の height
と width
を 200px に設定します。
さらに、Change Div Size
ボタンを追加しました。ユーザーがそれをクリックすると、changeDivSize()
関数が実行されます。
changeDivSize()
関数は、100 から 300 の間のランダムな値を生成し、それに応じて div
要素のサイズを変更します。 その後、ref.current.offsetWidth
を使用して、div
要素の新しい幅を取得しました。
ここでは、setTimeout()
関数を使用して、div
要素の新しい幅を取得するための遅延を追加しました。
コード例:
import { useState, useRef } from "react";
function App() {
// creating the variables for the div width and height
let [divWidth, setDivWidth] = useState("200px");
let [divheight, setDivheight] = useState("200px");
// variable to store the element's width from `ref`.
let [widthByRef, setWidthByRef] = useState(200);
// It is used to get the reference of any component or element
const ref = useRef(null);
// whenever user clicks on Change Div size button, ChangeDivSize() function will be called.
function changeDivSize() {
// get a random number between 100 and 300;
let random = 100 + Math.random() * (300 - 100);
// convert the random number to a string with pixels
random = random + "px";
// change the height and width of the div element.
setDivWidth(random);
setDivheight(random);
// get the width of the div element using ref.
// reason to add the setTimeout() function is that we want to get the width of the Div once it is updated in the DOM;
// Otherwise, it returns the old width.
setTimeout(() => {
setWidthByRef(ref.current ? ref.current.offsetWidth : 0);
}, 100);
}
return (
<div>
{/* showing width which we got using ref */}
<h3>Width of Element is : {widthByRef}</h3>
{/* resizable div element */}
<div
ref={ref}
style={{ backgroundColor: "red", height: divheight, width: divWidth }}
>
Click on the below buttons to resize the div.
</div>
{/* button to resize the div element */}
<button style={{ marginTop: "20px" }} onClick={changeDivSize}>
Change Div Size
</button>
</div>
);
}
export default App;
出力:
上記の出力では、ボタンをクリックすると、div
のサイズが変更され、Web ページに新しい幅が表示されることがわかります。