Python の Smith-Waterman アルゴリズム
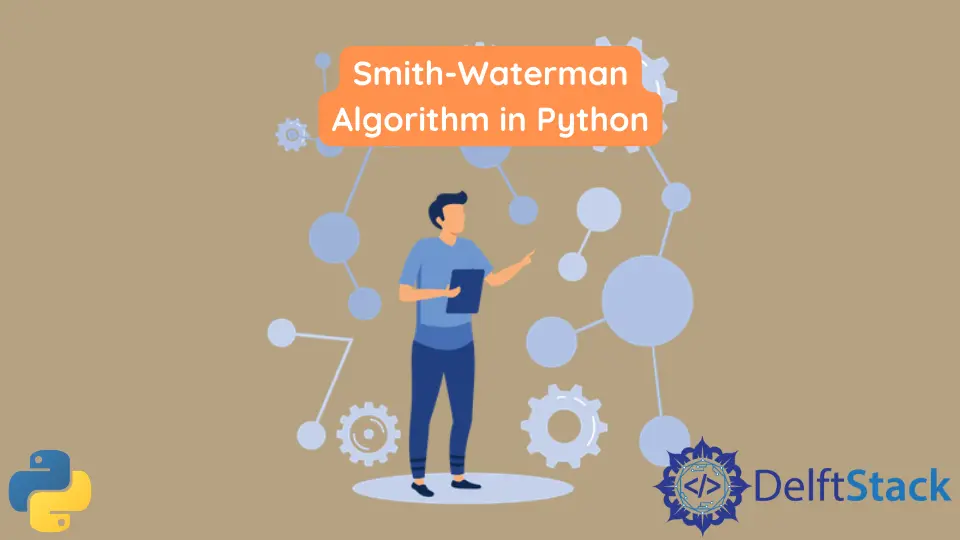
Smith-Waterman アルゴリズムは、文字列のローカルシーケンスアラインメントを実行するために使用されます。文字列は主に DNA 鎖またはタンパク質配列を表します。
この記事では、Python での Smith-Waterman アルゴリズムの実装について説明します。
Python の Smith-Waterman アルゴリズム
swalign
モジュールには、Python で Smith-Waterman アルゴリズムを実装するためのいくつかの関数が含まれています。コマンドラインで次のステートメントを実行することにより、PIP
を使用して swalign
モジュールをインストールできます。
pip3 install swalign
上記のステートメントは、Python バージョン 3 のモジュールをインストールします。Python バージョン 2 にモジュールをインストールするには、次のコマンドを使用できます。
pip install swalign
swalign
モジュールをインストールした後、次の手順を使用して、Python プログラムに Smith-Waterman アルゴリズムを実装します。
-
まず、
import
ステートメントを使用してswalign
モジュールをインポートします。 -
アラインメントを実行するには、ヌクレオチドスコアリングマトリックスを作成する必要があります。マトリックスでは、一致と不一致ごとにスコアを提供します。
通常、一致スコアには 2 を使用し、不一致には-1 を使用します。
-
ヌクレオチドスコアリングマトリックスを作成するには、
NucleotideScoringMatrix()
メソッドを使用します。NucleotideScoringMatrix()
は、最初の入力引数として一致スコアを取り、2 番目の入力引数として不一致スコアを取ります。実行後、
IdentityScoringMatrix
オブジェクトを返します。 -
ヌクレオチドマトリックスを取得したら、
LocalAlignment()
メソッドを使用してLocalAlignment
オブジェクトを作成します。LocalAlignment()
メソッドは、入力としてヌクレオチドスコアリングマトリックスを受け取り、LocalAlignment
オブジェクトを返します。 -
LocalAlignment
オブジェクトを取得したら、align()
メソッドを使用して Smith-Waterman アルゴリズムを実行できます。 -
align()
メソッドは、LocalAlignment
オブジェクトで呼び出されると、最初の入力引数として DNA 鎖を表す文字列を取ります。参照 DNA 鎖を表す別の文字列を取ります。 -
実行後、
align()
メソッドはAlignment
オブジェクトを返します。Alignment
オブジェクトには、入力文字列の一致の詳細と不一致、およびその他のいくつかの詳細が含まれています。
次の例では、プロセス全体を観察できます。
import swalign
dna_string = "ATCCACAGC"
reference_string = "ATGCAGCGC"
match_score = 2
mismatch_score = -1
matrix = swalign.NucleotideScoringMatrix(match_score, mismatch_score)
lalignment_object = swalign.LocalAlignment(matrix)
alignment_object = lalignment_object.align(dna_string, reference_string)
alignment_object.dump()
出力:
Query: 1 ATGCAGC-GC 9
||.|| | ||
Ref : 1 ATCCA-CAGC 9
Score: 11
Matches: 7 (70.0%)
Mismatches: 3
CIGAR: 5M1I1M1D2M
まとめ
この記事では、Python の swalign
モジュールを使用して Smith-Waterman アルゴリズムを実装する方法について説明します。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub