Python の無限ループのテキストメニュー
- Python の無限ループのテキストメニュー
-
Python で
break
ステートメントを使用して無限ループでテキストメニューを終了する -
Python の
Flag
変数を使用して無限ループでテキストメニューを終了する - まとめ
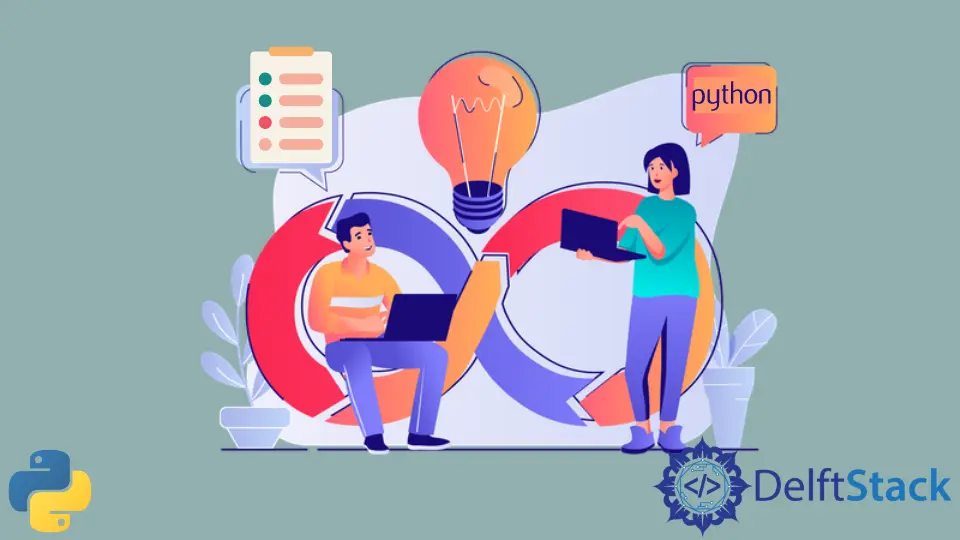
while
ループと if-else
ステートメントを使用して、Python プログラムにさまざまなツールを実装できます。この記事では、Python で無限ループのテキストメニューを作成します。
Python の無限ループのテキストメニュー
条件付きステートメントで while
ループを使用して、無限ループでテキストメニューを作成します。while
ループ内では、最初にユーザーにいくつかのオプションを表示し、オプションを表示した後、ユーザーからの入力を受け取ります。
入力を受け取った後、プログラムは目的の出力を出力します。最後に、プログラムはオプションを出力します。
これは、プログラムがユーザーによって手動で終了されるまで続きます。
これを理解するために、次のプログラムを検討してください。
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
while True:
options()
option = int(input())
if option == 1:
print("Hi")
elif option == 2:
print("Hello")
elif option == 3:
print("Namaste")
elif option == 4:
print("Bonjour")
elif option == 5:
print("Hola")
出力:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
1
Hi
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
2
Hello
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
^D
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/pythonProject/webscraping.py", line 11, in <module>
option = int(input())
EOFError: EOF when reading a line
上記のコードでは、最初に関数 options()
を定義して、ユーザーが利用できるさまざまなオプションを出力しています。その後、while
ループを作成しました。
while
ループ内で、最初に options()
関数を実行しました。その後、ユーザーに番号の入力を求めました。
続いて、input()
関数が文字列を返したため、int()
関数を使用して入力を整数に変換しました。
プログラムは、入力に従ってメッセージを出力しました。その後、プログラムは再びオプションを表示しました。
これは、ユーザーがプログラムを手動で終了するまで続きました。
次のセクションで説明するように、いくつかの方法を使用して while
ループを終了し、プログラムに進むことができます。
Python で break
ステートメントを使用して無限ループでテキストメニューを終了する
while
ループを終了するには、指定されたオプション以外の任意の番号を押すようにユーザーに求めます。その後、条件文に else
ブロックを含めます。
ユーザーが指定されたオプション以外の番号を入力すると、次の例に示すように、Bye
が出力され、break
ステートメントを使用して while
ループから抜け出します。
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
print("Enter any other number to terminate.")
while True:
options()
option = int(input())
if option == 1:
print("Hi")
elif option == 2:
print("Hello")
elif option == 3:
print("Namaste")
elif option == 4:
print("Bonjour")
elif option == 5:
print("Hola")
else:
print("Bye")
break
出力:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
1
Hi
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
3
Namaste
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
12
Bye
上記の例では、ユーザーが 1 から 5 以外の数値を入力すると、プログラムの実行は条件ステートメントの else
ブロックに入ります。したがって、プログラムは Bye
を出力し、break
ステートメントが実行されます。
このため、プログラムの実行は while
ループから外れます。
Python の Flag
変数を使用して無限ループでテキストメニューを終了する
break
ステートメントを使用する代わりに、flag
変数を使用して無限ループの実行を制御できます。まず、while
ループを実行する前に、flag
変数を True
に初期化します。
次に、flag
変数が True
の場合、while
ループを実行します。while
ループ内で、ユーザーが指定されたオプション以外の数値を入力すると、Bye
が出力され、値 False
が flag
変数に割り当てられます。
flag
変数が False
になると、while
ループの実行は自動的に終了します。これは、次のコードで確認できます。
def options():
print("Enter 1 to print 'Hi'.")
print("Enter 2 to print 'Hello'.")
print("Enter 3 to print 'Namaste'.")
print("Enter 4 to print 'Bonjour'.")
print("Enter 5 to print 'Hola'.")
print("Enter any other number to terminate.")
flag = True
while flag:
options()
option = int(input())
if option == 1:
print("Hi")
elif option == 2:
print("Hello")
elif option == 3:
print("Namaste")
elif option == 4:
print("Bonjour")
elif option == 5:
print("Hola")
else:
print("Bye")
flag = False
出力:
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
1
Hi
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
3
Namaste
Enter 1 to print 'Hi'.
Enter 2 to print 'Hello'.
Enter 3 to print 'Namaste'.
Enter 4 to print 'Bonjour'.
Enter 5 to print 'Hola'.
Enter any other number to terminate.
12
Bye
上記の例では、flag
変数が False
になると、while
ループの実行が終了します。これは、Python インタープリターが最初に flag
変数に値 True
が含まれているかどうかをチェックするためです。はいの場合、while
ループのみが実行されます。
値 False
を flag
変数に割り当てると、インタープリターは、while
ループを次に実行する前に、flag
変数の値をチェックします。flag
変数の値が False
であることを確認すると、while
ループを終了します。
まとめ
この記事では、Python の while
ループと条件ステートメントを使用して、無限ループのテキストメニューを作成しました。また、break
ステートメントと flag
変数を使用して無限ループの実行を終了する方法についても説明しました。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub