Python でプログラミングの基本概念を用いて乗算表を表示する
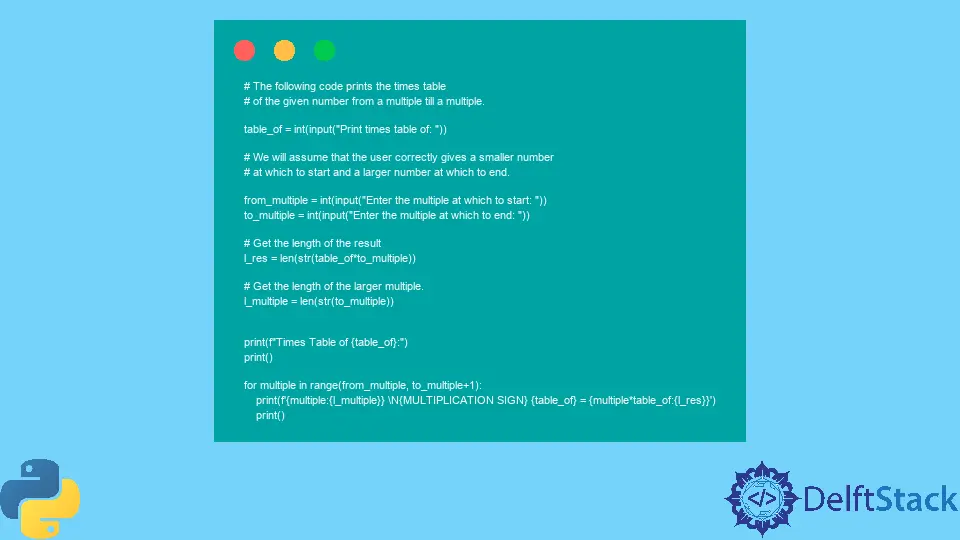
Python でタイムテーブルを印刷する方法を学ぶことで、いくつかの基本的なプログラミングの概念を実践できます。これらには以下が含まれます:
- 変数の使用
- ユーザー入力の取得
- 組み込み関数の使用
- キャスト変数を入力します
- 反復(ループ)
- 文字列のフォーマット
- Unicode シンボルの使用
Python 3.6 以降で使用できる Python の f
文字列フォーマット機能を使用します。
基本的なプログラミングの概念
次のように、変数を宣言して値を割り当てることができます。
table_of = 5
以下に示すように、input()
関数を使用してユーザー入力を取得します。
table_of = input("Print times table of: ")
プログラムは文字列 Print times table of:
を表示し、ユーザー入力を待ちます。ユーザーは何でも入力できます。Python は入力を文字列として解釈します。
これを整数に変換するには、input()
関数の周りに int()
関数を使用します。
table_of = int(input("Print times table of: "))
print()
Times)
はディスプレイに Times
という単語を印刷します。空の print()
関数は、空の行を出力します。
range()
関数は、start_int
から end_int
までのシーケンスを作成します。デフォルトでは、1 ずつ増加します。
range(start_int, end_int, step_int)
コードでは for
ループを使用します。変数が指定された範囲内にある回数だけ、ループ内のコードを繰り返します。
for variable in range(start, end):
code to repeat
Python の f
文字列フォーマット機能を使用すると、プレースホルダー{}
を使用して文字列に変数を含めることができます。変数 table_of
の値を使用するには、次を使用します。
print(f"Times table of {table_of}")
プレースホルダーの長さは整数で指定できます。コードでは、別の変数を使用してこれを指定します。結果の長さ table_of * 9
です。
str()
を使用して整数を文字列に変換し、長さを取得します。
乗算記号は、Unicode 名を使用して指定されます。
\N{MULTIPLICATION SIGN}
与えられた数のタイムズテーブルを Python で印刷する
上記のすべての概念を次のコードに入れます。ユーザー指定の数値の乗算表を 2つの方法で出力します。
サンプルコード:
# The following code prints the times table
# of the given number till 'number x 9'.
# It prints the times table in two different ways.
table_of = int(input("Print times table of: "))
# Get the length of the result
l_res = len(str(table_of * 9))
print(f"Times Table of {table_of}:")
print()
for multiple in range(1, 10):
print(
f"{multiple} \N{MULTIPLICATION SIGN} {table_of} = {table_of*multiple:{l_res}}"
)
print()
print("-------------")
print()
for multiple in range(1, 10):
print(
f"{table_of} \N{MULTIPLICATION SIGN} {multiple} = {table_of*multiple:{l_res}}"
)
print()
サンプル出力:
Print times table of: 1717
Times Table of 1717:
1 × 1717 = 1717
2 × 1717 = 3434
3 × 1717 = 5151
4 × 1717 = 6868
5 × 1717 = 8585
6 × 1717 = 10302
7 × 1717 = 12019
8 × 1717 = 13736
9 × 1717 = 15453
-------------
1717 × 1 = 1717
1717 × 2 = 3434
1717 × 3 = 5151
1717 × 4 = 6868
1717 × 5 = 8585
1717 × 6 = 10302
1717 × 7 = 12019
1717 × 8 = 13736
1717 × 9 = 15453
バリエーションとして、与えられた数の希望の倍数との間で乗算テーブルを印刷できます。
サンプルコード:
# The following code prints the times table
# of the given number from a multiple till a multiple.
table_of = int(input("Print times table of: "))
# We will assume that the user correctly gives a smaller number
# at which to start and a larger number at which to end.
from_multiple = int(input("Enter the multiple at which to start: "))
to_multiple = int(input("Enter the multiple at which to end: "))
# Get the length of the result
l_res = len(str(table_of * to_multiple))
# Get the length of the larger multiple.
l_multiple = len(str(to_multiple))
print(f"Times Table of {table_of}:")
print()
for multiple in range(from_multiple, to_multiple + 1):
print(
f"{multiple:{l_multiple}} \N{MULTIPLICATION SIGN} {table_of} = {multiple*table_of:{l_res}}"
)
print()
サンプル出力:
Print times table of: 16
Enter the multiple at which to start: 5
Enter the multiple at which to end: 15
Times Table of 16:
5 × 16 = 80
6 × 16 = 96
7 × 16 = 112
8 × 16 = 128
9 × 16 = 144
10 × 16 = 160
11 × 16 = 176
12 × 16 = 192
13 × 16 = 208
14 × 16 = 224
15 × 16 = 240