Python でファイル内の文字列を検索する
-
ファイル
readlines()
メソッドを使って Python でファイル内の文字列を探す -
ファイルの中の文字列を検索するためにファイル
read()
メソッドを使用する -
Python でファイル内の文字列を検索するには
find
メソッドを使用する -
Python でファイル内の文字列を検索するには
mmap
モジュールを用いる
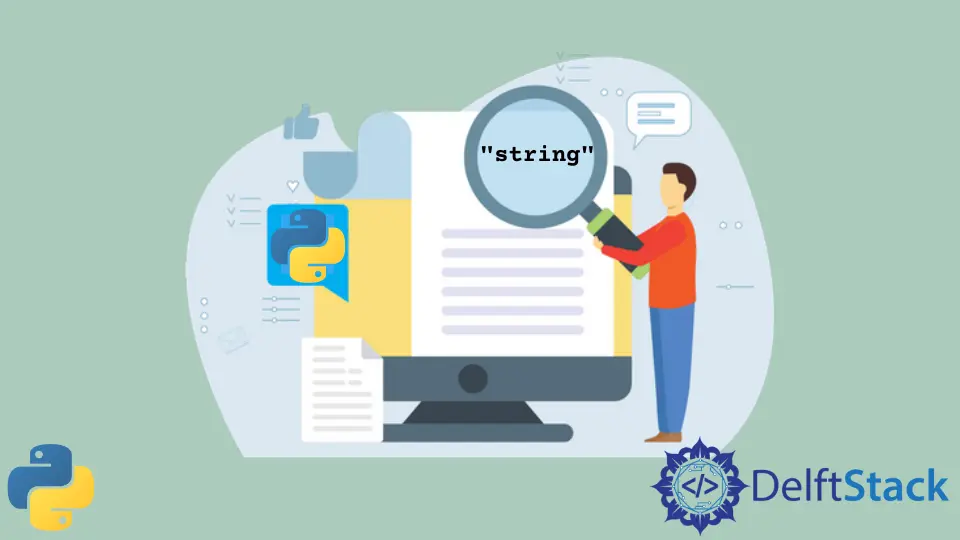
チュートリアルでは、Python でテキストファイル内の特定の文字列を検索する方法を説明しています。
ファイル readlines()
メソッドを使って Python でファイル内の文字列を探す
Pyton のファイル readlines()
メソッドは、ファイルの内容を改行してリストに分割したものを返します。for
ループを使ってリストを反復し、in
演算子を使って文字列が行内にあるかどうかを確認することができます。
行内に文字列が見つかった場合は True
を返してループを中断します。すべての行を繰り返しても文字列が見つからなかった場合は、最終的に False
を返します。
このアプローチの例を以下に示します。
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
def check_string():
with open("temp.txt") as temp_f:
datafile = temp_f.readlines()
for line in datafile:
if "blabla" in line:
return True # The string is found
return False # The string does not exist in the file
if check_string():
print("True")
else:
print("False")
出力:
True
ファイルの中の文字列を検索するためにファイル read()
メソッドを使用する
ファイル read()
メソッドはファイルの内容を文字列全体として返します。次に in
演算子を用いて、返された文字列の中にその文字列があるかどうかを調べることができます。
以下にコード例を示します。
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
if "blabla" in f.read():
print("True")
以下にコード例を示します。
True
Python でファイル内の文字列を検索するには find
メソッドを使用する
単純な find
メソッドを read()
メソッドと一緒に使うと、ファイル内の文字列を見つけることができます。find
メソッドには必要な文字列が渡されます。文字列が見つかった場合は 0
を、見つからなかった場合は -1
を返します。
以下にコード例を示します。
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
print(open("temp.txt", "r").read().find("blablAa"))
出力:
-1
Python でファイル内の文字列を検索するには mmap
モジュールを用いる
また、mmap
モジュールは、Python でファイル内の文字列を探すのにも利用でき、ファイルサイズが比較的大きい場合にはパフォーマンスを向上させることができます。mmap.mmap()
メソッドは Python 2 で文字列のようなオブジェクトを生成し、暗黙のファイルのみをチェックし、ファイル全体を読み込まないようにします。
Python 2 でのコード例を以下に示します。
# python 2
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find("blabla") != -1:
print("True")
出力:
True
しかし、Python 3 以上では mmap
は文字列状のオブジェクトのような振る舞いはせず、bytearray
オブジェクトを生成します。そのため、find
メソッドは文字列ではなくバイトを探します。
そのためのエクスマップコードを以下に示します。
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find(b"blabla") != -1:
print("True")
出力:
True
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn関連記事 - Python String
- Python で文字列からコンマを削除する
- Pythonic な方法で文字列が空かどうかを確認する方法
- Python で文字列を変数名に変換する
- Python 文字列の空白を削除する方法
- Python で文字列から数字を抽出する
- Python が文字列を日時 datetime に変換する方法