Python で CSV ファイルに新しい行を追加する
Najwa Riyaz
2023年1月30日
Python
Python CSV
-
writer.writerow()
を使用して、Python の CSV ファイルにリスト内のデータを追加する -
DictWriter.writerow()
を使用して、辞書のデータを Python の CSV ファイルに追加する
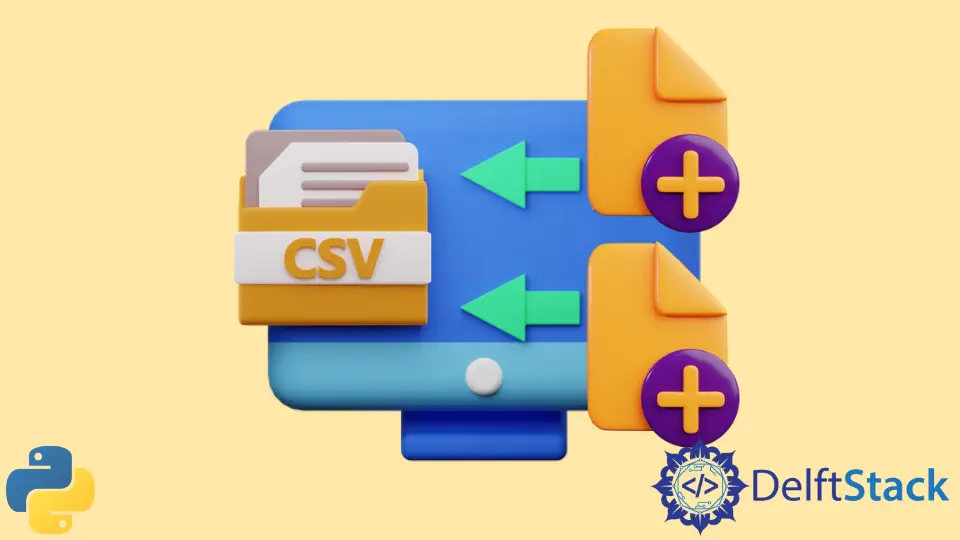
Python で CSV ファイルに新しい行を追加する場合は、次のいずれかの方法を使用できます。
- 目的の行のデータをリストに割り当てます。次に、
writer.writerow()
を使用して、このリストのデータを CSV ファイルに追加します。 - 目的の行のデータを辞書に割り当てます。次に、
DictWriter.writerow()
を使用して、この辞書のデータを CSV ファイルに追加します。
writer.writerow()
を使用して、Python の CSV ファイルにリスト内のデータを追加する
この場合、古い CSV ファイルに新しい行を追加する前に、行の値をリストに割り当てる必要があります。
例えば、
list = ["4", "Alex Smith", "Science"]
次に、このデータをリストの引数として CSV writer()
オブジェクトの writerow()
関数に渡します。
例えば、
csvwriter_object.writerow(list)
前提条件:
-
CSV
writer
クラスはCSV
モジュールからインポートする必要があります。from csv import writer
-
コードを実行する前に、CSV ファイルを手動で閉じる必要があります。
例-writer.writerow()
を使用してリスト内のデータを CSV ファイルに追加する
これは、リストに存在するデータを CSV ファイルに追加する方法を示すコードの例です-
# Pre-requisite - Import the writer class from the csv module
from csv import writer
# The data assigned to the list
list_data = ["03", "Smith", "Science"]
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the writer() function
writer_object = writer(f_object)
# Result - a writer object
# Pass the data in the list as an argument into the writerow() function
writer_object.writerow(list_data)
# Close the file object
f_object.close()
コードを実行する前に仮定します。古い CSV ファイルには以下の内容が含まれています。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
コードが実行されると、CSV ファイルが変更されます。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
DictWriter.writerow()
を使用して、辞書のデータを Python の CSV ファイルに追加する
この場合、新しい行を古い CSV ファイルに追加する前に、行の値をディクショナリに割り当てます。
例えば、
dict = {"ID": 5, "NAME": "William", "SUBJECT": "Python"}
次に、このデータをディクショナリからの引数としてディクショナリ DictWriter()
オブジェクトの writerow()
関数に渡します。
例えば、
dictwriter_object.writerow(dict)
前提条件:
-
DictWriter
クラスはCSV
モジュールからインポートする必要があります。from csv import DictWriter
-
コードを実行する前に、CSV ファイルを手動で閉じる必要があります。
例-DictWriter.writerow()
を使用して辞書のデータを CSV ファイルに追加する
これは、辞書にあるデータを CSV ファイルに追加する方法を示すコードの例です。
# Pre-requisite - Import the DictWriter class from csv module
from csv import DictWriter
# The list of column names as mentioned in the CSV file
headersCSV = ["ID", "NAME", "SUBJECT"]
# The data assigned to the dictionary
dict = {"ID": "04", "NAME": "John", "SUBJECT": "Mathematics"}
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the Dictwriter() function
# Result - a DictWriter object
dictwriter_object = DictWriter(f_object, fieldnames=headersCSV)
# Pass the data in the dictionary as an argument into the writerow() function
dictwriter_object.writerow(dict)
# Close the file object
f_object.close()
コードを実行する前に、古い CSV ファイルに以下のコンテンツが含まれているとします。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
コードが実行されると、CSV ファイルが変更されます。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
04,John,Mathematics
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe