Python のループ内の辞書にキーと値のペアを追加する
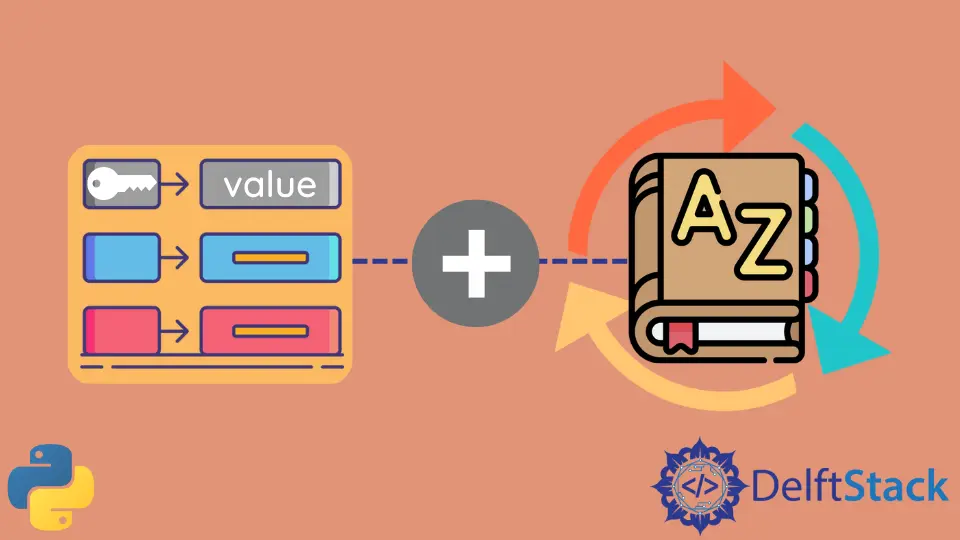
辞書は、Python でキーと値のペアの形式でデータを格納するための驚くべき効率的なデータ構造です。
データ構造であるため、辞書は Python に固有であるだけでなく、C++、Java、JavaScript などの他のプログラミング言語でも使用できます。map や JSON(JavaScript Object Notation)などの別の名前で参照されます。物体。
ディクショナリにはキーがあり、キーはハッシュ可能で不変の任意の値またはオブジェクトにすることができます。これらの 2つの要件の背後にある理由は、オブジェクトのハッシュ表現が、オブジェクト内に格納されている値に依存するためです。
値を時間の経過とともに操作できる場合、オブジェクトには一意の固定ハッシュ表現がありません。辞書内の値は何でもかまいません。整数値、浮動小数点値、倍精度値、文字列値、クラスオブジェクト、リスト、バイナリツリー、リンクリスト、関数、さらには辞書にすることができます。
時間計算量に関して言えば、辞書は、要素の追加、削除、およびアクセスに、平均して一定の時間 O(1)
を要します。
この記事では、ループ内の辞書にキーと値のペアを追加する方法について説明します。
ループ内の辞書にキーと値のペアを追加する
ループ内の辞書にキーと値のペアを追加するために、辞書のキーと値を格納する 2つのリストを作成できます。次に、ith
キーが ith
値を意味すると仮定すると、2つのリストを一緒に繰り返し処理し、ディクショナリ内のそれぞれのキーに値を追加できます。
いくつかの Python コードを使用してこれを理解しましょう。次のコードを参照してください。
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
for key, value in zip(keys, values):
dictionary[key] = value
print(dictionary)
出力:
{'Integer': 1, 'String': 'Hello', 'Float': 2.567, 'List of Strings': ['Computer', 'Science'], 'List of Float Numbers': [1.235, 5.253, 77.425], 'List of Integers': [11, 22, 33, 44, 55], 'Dictionary': {'a': 500, 'b': 1000, 'c': 1500}, 'Class Object': <__main__.Point object at 0x7f2c74906d90>, 'Function': <function add at 0x7f2c748a3d30>, 'List of Class Objects': [<__main__.Point object at 0x7f2c749608b0>, <__main__.Point object at 0x7f2c748a50a0>, <__main__.Point object at 0x7f2c748a5430>, <__main__.Point object at 0x7f2c748a53d0>]}
上記のソリューションの時間計算量は O(n)
であり、上記のソリューションの空間計算量も O(n)
です。ここで、n
はキー
および値
リストのサイズです。さらに、上記のコードは、説明したすべてのタイプの値をディクショナリ内に格納できることを示しています。
辞書を繰り返して各キーと値のペアを出力するか、各キーと値のペアを辞書に追加しながら出力することで、出力を美しくすることができます。json
パッケージや外部のオープンソースパッケージなどのビルド済みの Python パッケージを使用して、JSON 出力を試したり、色分けを追加したり、インデントを追加したりすることもできます。
このユースケースでは、辞書を出力するためのスタブ関数を作成します。次のコードを参照してください。
def print_dictionary(dictionary):
for key, value in dictionary.items():
print(f"{key}: {value}")
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
for key, value in zip(keys, values):
dictionary[key] = value
print_dictionary(dictionary)
出力:
Integer: 1
String: Hello
Float: 2.567
List of Strings: ['Computer', 'Science']
List of Float Numbers: [1.235, 5.253, 77.425]
List of Integers: [11, 22, 33, 44, 55]
Dictionary: {'a': 500, 'b': 1000, 'c': 1500}
Class Object: <__main__.Point object at 0x7f7d94160d90>
Function: <function add at 0x7f7d940fddc0>
List of Class Objects: [<__main__.Point object at 0x7f7d941ba8b0>, <__main__.Point object at 0x7f7d940ff130>, <__main__.Point object at 0x7f7d940ff310>, <__main__.Point object at 0x7f7d940ff3d0>]
上記のソリューションの時間と空間の複雑さは、前のソリューションの O(n)
と同じです。
上記の 2つのコードスニペットは、for
ループを使用しています。while
ループを使用して同じタスクを実行できます。
次のコードスニペットは、while
ループを使用してディクショナリに値を追加する方法を示しています。
def print_dictionary(dictionary):
for key, value in dictionary.items():
print(f"{key}: {value}")
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def add(*args):
s = 0
for x in args:
s += x
return s
dictionary = {}
keys = [
"Integer",
"String",
"Float",
"List of Strings",
"List of Float Numbers",
"List of Integers",
"Dictionary",
"Class Object",
"Function",
"List of Class Objects",
]
values = [
1,
"Hello",
2.567,
["Computer", "Science"],
[1.235, 5.253, 77.425],
[11, 22, 33, 44, 55],
{"a": 500, "b": 1000, "c": 1500},
Point(1, 6),
add,
[Point(0, 0), Point(0, 7.5), Point(7.5, 7.5), Point(7.5, 0)],
]
n = min(len(keys), len(values))
i = 0
while i != n:
dictionary[keys[i]] = values[i]
i += 1
print_dictionary(dictionary)
出力:
Integer: 1
String: Hello
Float: 2.567
List of Strings: ['Computer', 'Science']
List of Float Numbers: [1.235, 5.253, 77.425]
List of Integers: [11, 22, 33, 44, 55]
Dictionary: {'a': 500, 'b': 1000, 'c': 1500}
Class Object: <__main__.Point object at 0x7fdbe16c0d90>
Function: <function add at 0x7fdbe165ddc0>
List of Class Objects: [<__main__.Point object at 0x7fdbe171a8b0>, <__main__.Point object at 0x7fdbe165f130>, <__main__.Point object at 0x7fdbe165f310>, <__main__.Point object at 0x7fdbe165f3d0>]
上記のソリューションの時間と空間の複雑さは、前のソリューションの O(n)
と同じです。