Python で文字列から句読点を取り除く方法
Hassan Saeed
2023年1月30日
Python
Python String
-
Python で文字列から句読点を取り除く
string
クラスのメソッドを使用する -
Python で文字列から句読点を取り除くには
regex
を使用する -
Python で文字列から句読点を取り除くには
string.punctuation
を使用する -
Python で文字列から句読点を取り除くには
replace()
を使用する
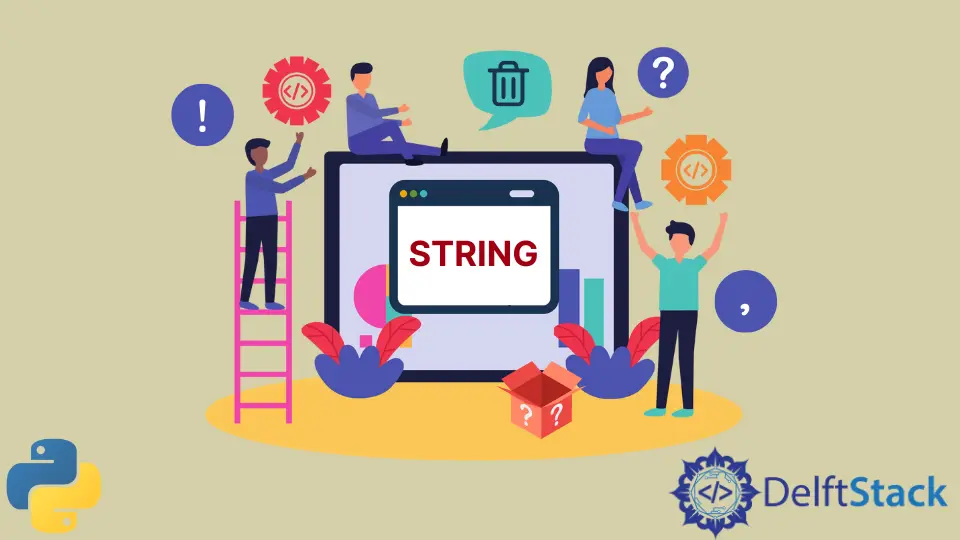
このチュートリアルでは、Python で文字列から句読点を取り除く方法について説明します。これは、NLP のためにテキストデータを前処理したり、クリーニングしたりする際に特に有用なステップです。
Python で文字列から句読点を取り除く string
クラスのメソッドを使用する
Python で文字列から句読点を取り除くには、string
クラスで提供されている組み込み関数を使用することができます。以下の例はこれを示しています。
s = "string. With. Punctuations!?"
out = s.translate(str.maketrans("", "", string.punctuation))
print(out)
出力:
'string With Punctuations'
上記のメソッドは、与えられた入力文字列からすべての句読点を削除します。
Python で文字列から句読点を取り除くには regex
を使用する
Python では、文字列から句読点を取り除くために regex
を使用することもできます。以下の例はこれを示しています。
import re
s = "string. With. Punctuation?"
out = re.sub(r"[^\w\s]", "", s)
print(out)
出力:
'string With Punctuations'
Python で文字列から句読点を取り除くには string.punctuation
を使用する
これは最初に説明した方法と似ています。string.punctuation
は英語で句読点とみなされるすべての文字を含む。このリストを使って、文字列からすべての句読点を除外することができます。以下の例はこれを示しています。
s = "string. With. Punctuation?"
out = "".join([i for i in s if i not in string.punctuation])
print(out)
出力:
'string With Punctuations'
Python で文字列から句読点を取り除くには replace()
を使用する
Python では、文字列から句読点を取り除くために replace()
を使用することもできます。ここでも string.punctuation
を使ってリストを定義し、すべての句読点を空の文字列に置き換えて句読点を削除します。以下の例はこれを示しています。
s = "string. With. Punctuation?"
punct = string.punctuation
for c in punct:
s = s.replace(c, "")
print(s)
出力:
'string With Punctuations'
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe