Python 辞書を値でソートする方法
胡金庫
2023年1月30日
- Python の値による辞書のソート-ソートされた値のみを取得
-
operator.itemgetter
を使用して Python 辞書をソートする -
Python の辞書をソートするために、
sorted
のキーでlambda
関数を使用する -
Python の辞書ソートで辞書互換の結果を得るための
OrderedDict
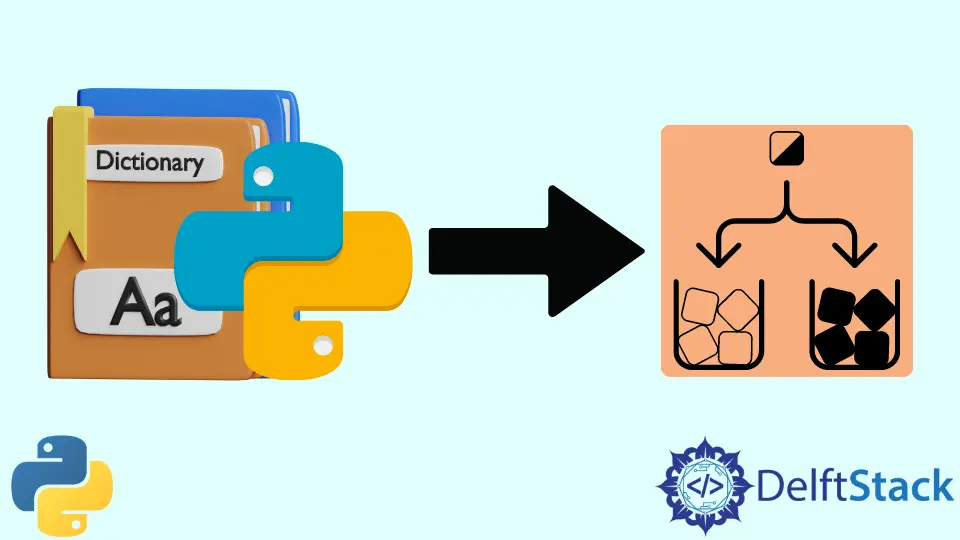
Python 辞書は順序のないデータ型であるため、キーまたは値で Python 辞書を並べ替えることはできません。しかし、リストのような他のデータ型でソートされた Python 辞書の表現を取得できます。
以下のような辞書があるとします。
exampleDict = {"first": 3, "second": 4, "third": 2, "fourth": 1}
Python の値による辞書のソート-ソートされた値のみを取得
sortedDict = sorted(exampleDict.values())
# Out: [1, 2, 3, 4]
operator.itemgetter
を使用して Python 辞書をソートする
import operator
sortedDict = sorted(exampleDict.items(), key=operator.itemgetter(1))
# Out: [('fourth', 1), ('third', 2), ('first', 3), ('second', 4)]
exampleDict.items
は、辞書要素のキーと値のペアを返します。key=operator.itemgetter(1)
は、比較キーが辞書の値であることを指定し、一方、operator.itemgetter(0)
は辞書キーの比較キーを持ちます。
Python の辞書をソートするために、sorted
のキーで lambda
関数を使用する
operator.itemgetter
の代わりに lambda
関数で比較キーを取得することもできます
sortedDict = sorted(exampleDict.items(), key=lambda x: x[1])
# Out: [('fourth', 1), ('third', 2), ('first', 3), ('second', 4)]
exampleDict.items()
は辞書のキーと値のペアのリストを返し、その要素のデータ型はタプルです。x
はこのタプルの要素です。x[0]
はキー、x[1]
は値です。key=lambda x:x[1]
は、比較キーが辞書要素の値であることを示します。
値を降順に並べ替える必要がある場合、オプションのパラメーターreverse
を True
に設定できます。
sortedDict = sorted(exampleDict.items(), key=lambda x: x[1], reverse=True)
# Out: [('second', 4), ('first', 3), ('third', 2), ('fourth', 1)]
Python の辞書ソートで辞書互換の結果を得るための OrderedDict
上記のサンプルコードは、辞書型ではなくリストとして結果を返します。結果を辞書互換型として保持したい場合は、Python 2.7 から導入された OrderedDict
が正しい選択です。
from collections import OrderedDict
sortedDict = OrderedDict(sorted(exampleDict.items(), key=lambda x: x[1]))
# Out: OrderedDict([('fourth', 1), ('third', 2), ('first', 3), ('second', 4)])
OrderedDict
は Python の dict
サブクラスであり、通常のメソッドをサポートし、キーが最初に挿入される順序も記憶しています。
著者: 胡金庫