IndexError の解決: インデックスとして使用される配列は、整数(またはブール) 型でなければなりません
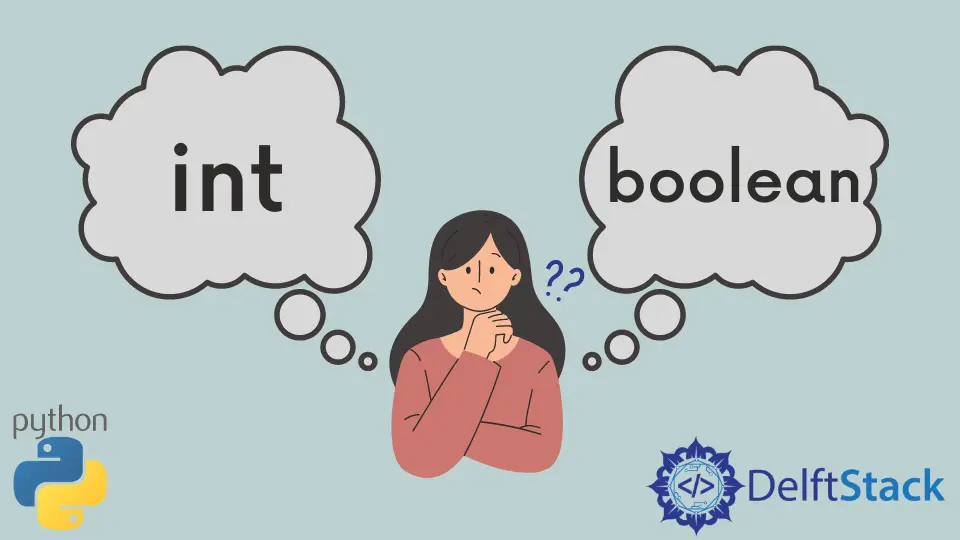
Python で Numpy 配列を操作する場合、インデックスまたはタイプの問題を扱うさまざまなエラー メッセージが表示されることがあります。 これらの多くのエラー タイプでは、IndexError: インデックスとして使用される配列は整数 (またはブール) 型でなければなりません
は注意が必要です。
IndexError
エラー メッセージに直面した場合、間違ったタイプを使用します。 この場合、Integer
または Boolean
を使用することになっていましたが、配列インデックスは別のデータ型 (string または float) を受け取ります。
この記事では、Numpy で数値を操作する際の IndexError: arrays used as indexes must be of integer (or boolean) type
エラー メッセージの対処方法について説明します。
astype()
を使用して Numpy で IndexError: array used as index must be of integer (or boolean) type
を解決する
Numpy は、Integer または Boolean の 2つのタイプでのみ機能します。 そのため、理解できない型があるとエラーになります。
このエラー メッセージをよりよく理解するために、エラー メッセージを再作成してみましょう。 エラー メッセージを再現するには、2つの Numpy 配列 index
と array
を生成し、index
から値を抽出し、抽出した値を使用して array
の値にアクセスする必要があります。
抽出された値については、最初の列の値を使用します。
import numpy as np
index = np.array([[0, 1, 2.1], [1, 2, 3.4]])
array = np.array([[1, 2, 3], [4, 5, 6]])
indices = index[:, 0]
print(array[indices])
出力:
Traceback (most recent call last):
File "temp.py", line 7, in <module>
print(array[indices])
IndexError: arrays used as indices must be of integer (or boolean) type
IndexError: arrays used as indicators must be of integer (or boolean) type
エラー メッセージから、問題が print(array[indices])
セクションに起因することがわかります。
構文的に正しいことがわかっているので、探している問題が array
バインディングに解析しているものに存在することがわかります。 これで、indices
バインディングにたどり着きます。
エラー メッセージからわかることによると、indices
バインディングの要素は integer
または Boolean
ではない可能性があります。 dtype
プロパティは、indices
内の要素のタイプをチェックするのに役立ちます。
print(indices.dtype)
出力:
float64
これで、私たちが直面している問題の原因が確認されました。 array
バインディングのインデックスに渡す値は、Boolean
ではなく float64
です。
これを解決するには、indices
の値を Integer
または Boolean
に変換する必要があります。 それらを Integer
に変換する方が理にかなっています。
それらを Boolean
に変換すると、別の機会に役立つかもしれません。
astype()
メソッド は、Numpy 配列の dtype
プロパティを変更するのに役立ちます。 indices
バインディングの dtype
を変更するには、以下を使用できます。
indices = index[:, 0].astype(int)
indices.dtype
式を使用して dtype
プロパティをチェックすると、以下が得られます。
int32
これで、コードは次のようになります。
import numpy as np
index = np.array([[0, 1, 2.1], [1, 2, 3.4]])
array = np.array([[1, 2, 3], [4, 5, 6]])
indices = index[:, 0].astype(int)
print(array[indices])
出力:
[[1 2 3]
[4 5 6]]
indices
の値を Boolean
に変換できました。 それを試してみましょう。
そのために、2つのブール値を持つ Numpy 配列があります。
indices = index[:, 0].astype(bool)
print(indices)
出力:
[False True]
indices
バインディングの値は [0. 1.]
であり、0
をブール値に変換すると False
になり、それ以外の数値は True
になります。 すべてを一緒に実行しましょう。
import numpy as np
index = np.array([[0, 1, 2.1], [1, 2, 3.4]])
array = np.array([[1, 3, 5], [7, 9, 11]])
indices = index[:, 0].astype(bool)
print(array[indices])
出力:
[[ 7 9 11]]
これは、True
値のみを処理するためです。
したがって、IndexError: arrays used as indexes must be of integer (or boolean) type
エラー メッセージが表示された場合は、どこかに間違った dtype
があることを知ってください。 コードをトレースし、必要な値を変換します。
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn関連記事 - Python Error
- AttributeError の解決: 'list' オブジェクト属性 'append' は読み取り専用です
- AttributeError の解決: Python で 'Nonetype' オブジェクトに属性 'Group' がありません
- AttributeError: 'generator' オブジェクトに Python の 'next' 属性がありません
- AttributeError: 'numpy.ndarray' オブジェクトに Python の 'Append' 属性がありません
- AttributeError: Int オブジェクトに属性がありません
- AttributeError: Python で 'Dict' オブジェクトに属性 'Append' がありません