Pandas を使用して DataFrame 内の重複行を検索する
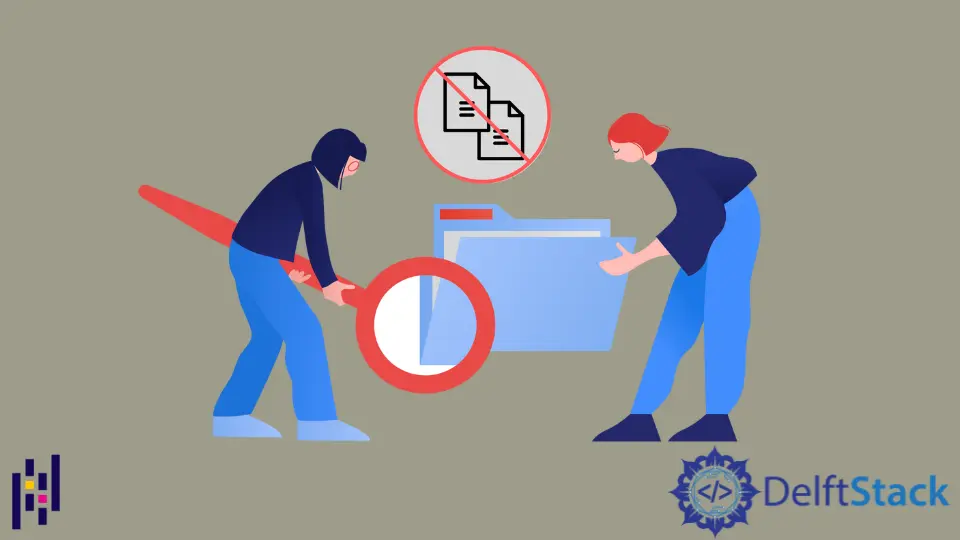
クリーニング手順の一環として、重複する値をデータ セットから特定する必要があります。 重複データは不要なストレージ スペースを消費し、少なくとも計算速度を低下させます。 ただし、最悪の場合、データの重複によって分析結果が歪められ、データ セットの整合性が損なわれる可能性があります。
Pandas と呼ばれるオープンソースの Python パッケージは、構造化データの処理と保存を強化します。 さらに、このフレームワークには、重複する行や列を見つけて削除するなど、データ クリーニング手順に対する組み込みの支援機能が用意されています。
この記事では、列のすべてまたはサブセットを使用して、Pandas データフレームで重複を見つける方法について説明します。 このために、Pandas の Dataframe.duplicated()
メソッドを使用します。
DataFrame.duplicated()
メソッドを使用して、DataFrame 内の重複行を検索する
Python の DataFrame
クラスの Pandas ライブラリには、次のようなすべての列またはそれらの列のサブセットに基づいて重複行を検出するメンバー メソッドが用意されています。
DataFrame.duplicated(subset=None, keep="first")
行が重複しているか一意であるかを示す一連のブール値を返します。
パラメーター:
subset
: これには、列または列ラベルのコレクションが必要です。 None がデフォルト値です。 列を渡した後は、重複のみが考慮されます。keep
: 重複値の処理を規制します。first
がデフォルトで、3つの異なる値しかありません。first
の場合、最初のアイテムは一意として扱われ、残りの値は重複として扱われます。latest
の場合、最後のアイテムは一意として扱われ、残りの値は重複として扱われます。False
の場合、すべての同一の値は重複と見なされます。- ブール系列によって示される重複行を返します。
重複行を含む DataFrame を作成する
リストのコレクションを含む基本的なデータフレームを作成し、列にName
、Age
、およびCity
という名前を付けましょう。
コード例:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
print(df)
出力:
Name Age City
0 Joe 28 Chicago
1 John 32 Austin
2 Melvin 25 Dallas
3 John 32 Austin
4 John 32 Austin
5 John 32 Houston
6 Melvin 40 Dehradun
7 Hazel 32 Austin
すべての列に基づいて重複行を選択
subset
パラメータなしで Dataframe.duplicate()
を呼び出して、すべての列に応じてすべての行の重複を見つけて選択します。 ただし、重複する行がある場合は、最初のインスタンスの位置に True
を持つブール系列のみが返されます (retain 引数のデフォルト値は first
)。
次に、このブール シリーズを DataFrame の []
演算子に渡して、重複する行を選択します。
コード例:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
duplicate = df[df.duplicated()]
print("Duplicate Rows :")
print(duplicate)
出力:
Duplicate Rows :
Name Age City
3 John 32 Austin
4 John 32 Austin
最終的なものを除くすべての重複を考慮したい場合は、引数として retain = "last"
を渡します。
コード例:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
duplicate = df[df.duplicated(keep="last")]
print("Duplicate Rows :")
print(duplicate)
出力:
Duplicate Rows :
Name Age City
1 John 32 Austin
3 John 32 Austin
次に、指定されたいくつかの列に応じて重複する行のみを選択する場合は、subset
の列名のリストをパラメーターとして指定します。
コード例:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
# on 'City' column
duplicate = df[df.duplicated("City")]
print("Duplicate Rows based on City:")
print(duplicate)
出力:
Duplicate Rows based on City:
Name Age City
3 John 32 Austin
4 John 32 Austin
7 Hazel 32 Austin
Name
や Age
など、複数の列名に基づいて重複する行を選択します。
コード例:
# Import pandas library
import pandas as pd
# List of Tuples
employees = [
("Joe", 28, "Chicago"),
("John", 32, "Austin"),
("Melvin", 25, "Dallas"),
("John", 32, "Austin"),
("John", 32, "Austin"),
("John", 32, "Houston"),
("Melvin", 40, "Dehradun"),
("Hazel", 32, "Austin"),
]
df = pd.DataFrame(employees, columns=["Name", "Age", "City"])
# list of the column names
duplicate = df[df.duplicated(["Name", "Age"])]
print("Duplicate the rows based on Name and Age:")
print(duplicate)
出力:
Duplicate Rows based on Name and Age:
Name Age City
3 John 32 Austin
4 John 32 Austin
5 John 32 Houston
まとめ
DataFrame で重複する行を見つけるには、Pandas で dataframe.duplicated()
メソッドを使用します。 行が重複しているか一意であるかを示す一連のブール値を返します。
この記事が、ここで説明したすべての例を確認することで、列のすべてまたはサブセットを使用してデータフレーム内の重複行を見つけるのに役立つことを願っています。 次に、上記の簡単な手順を使用して、Pandas を使用して重複を見つける方法をすばやく判断できます。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn