Pandas DataFrame の行数を取得する方法
Asad Riaz
2023年1月30日
Pandas
Pandas DataFrame Row
-
DataFrame
の行数を取得するの.shape
メソッド -
Pandas で行数を取得する最速のメソッドとして
.len(DataFrame.index)
-
Pandas の条件を満たす行をカウントする
dataframe.apply()
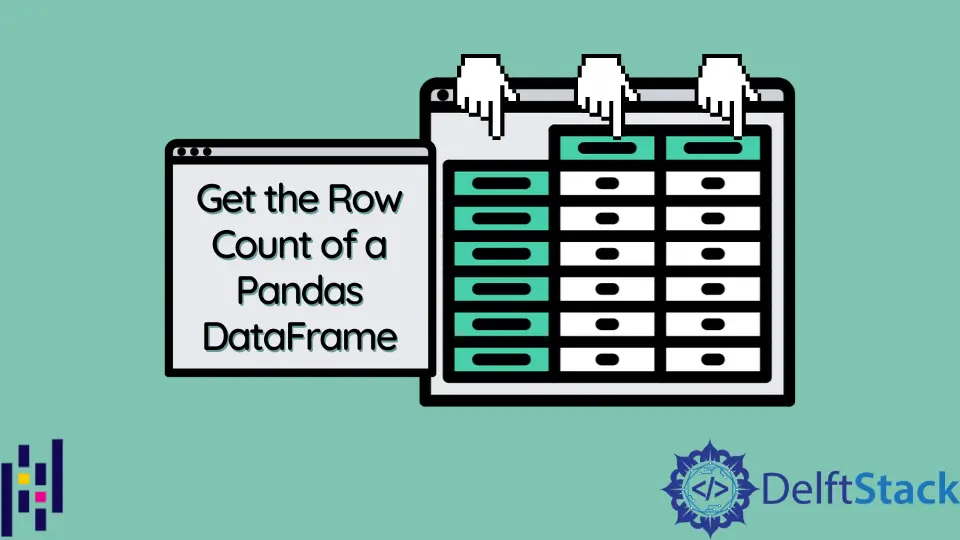
Pandas の行数を取得する方法を紹介します。shape
や len(DataFrame.index)
などのさまざまなメソッドを使用します。len(DataFrame.index)
が最速であることが判明した顕著なパフォーマンスの違いがあります。
また、dataframe.apply()
を使用して、条件を満たす行の要素数を取得する方法についても説明します。
DataFrame
の行数を取得するの .shape
メソッド
行数を計算するには、
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", df.shape[0])
出力:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
列数には、df.shape[1]
を使用できます。
Pandas で行数を取得する最速のメソッドとして .len(DataFrame.index)
DataFrame
の行数を計算するには、インデックス行の長さを取得します。
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.index))
出力:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
df.index
の代わりに df.axes[0]
を渡すこともできます:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
print(df)
print("Row count is:", len(df.axes[0]))
出力:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count is: 3
列数のカウントには、df.axes[1]
を使用できます。
Pandas の条件を満たす行をカウントする dataframe.apply()
dataframe.apply()
の返された結果の True
の数をカウントすることで、条件を満たす DataFrame
の行数を取得できます。
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(np.arange(15).reshape(3, 5))
counterFunc = df.apply(lambda x: True if x[1] > 3 else False, axis=1)
numOfRows = len(counterFunc[counterFunc == True].index)
print(df)
print("Row count > 3 in column[1]is:", numOfRows)
出力:
0 1 2 3 4
0 0 1 2 3 4
1 5 6 7 8 9
2 10 11 12 13 14
Row count > 3 in column[1]is: 2
column[1]
の値が 3 より大きい行の数を取得しています。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe