Pandas の日時列から月と年を別々に抽出する方法
-
pandas.Series.dt.year()
およびpandas.Series.month()
と年を抽出するdt.month()
メソッド -
年と月を抽出する
strftime()
メソッド -
年と月を抽出するための
pandas.DatetimeIndex.month
とpandas.DatetimeIndex.year
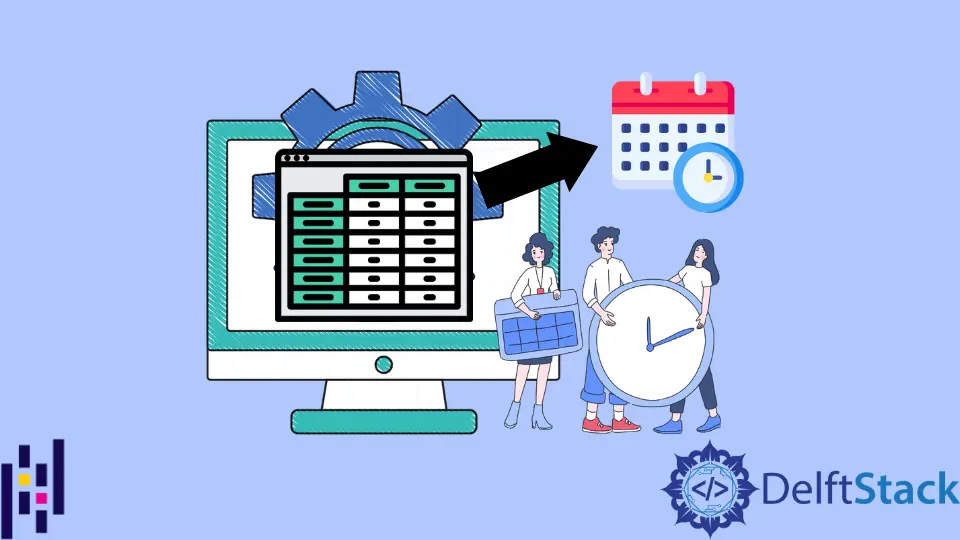
pandas.Series.dt.year()
メソッドと pandas.Series.dt.month()
メソッドをそれぞれ使用して、Datetime
列から年と月を抽出できます。データが Datetime
型でない場合は、最初に Datetime
に変換する必要があります。pandas.DatetimeIndex.year
と strftime()
メソッドとともに、pandas.DatetimeIndex.month
を使用して年と月を抽出することもできます。
pandas.Series.dt.year()
および pandas.Series.month()
と年を抽出する dt.month()
メソッド
Datetime
タイプに適用された pandas.Series.dt.year()
メソッドと pandas.Series.dt.month()
メソッドは、シリーズオブジェクトの Datetime
エントリの年と月の NumPy
配列をそれぞれ返します。
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["Year"] = df["Joined date"].dt.year
df["Month"] = df["Joined date"].dt.month
print(df)
出力:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
ただし、列が Datetime
型でない場合は、最初に to_datetime()
メソッドを使用して列を Datetime
型に変換する必要があります。
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["11/20/2019", "01/02/2020", "02/05/2020", "03/10/2020", "04/16/2020"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["Joined date"] = pd.to_datetime(df["Joined date"])
df["Year"] = df["Joined date"].dt.year
df["Month"] = df["Joined date"].dt.month
print(df)
出力:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
年と月を抽出する strftime()
メソッド
strftime()
メソッドは、Datetime を入力として受け取り、出力で指定された特定の形式を表す文字列を返します。年と月を抽出するためのフォーマットコードとして、%Y
と%m
を使用します。
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["year"] = df["Joined date"].dt.strftime("%Y")
df["month"] = df["Joined date"].dt.strftime("%m")
print(df)
出力:
Joined date year month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 01
Zeppy 2020-02-05 2020 02
Alina 2020-03-10 2020 03
Jerry 2020-04-16 2020 04
年と月を抽出するための pandas.DatetimeIndex.month
と pandas.DatetimeIndex.year
Datetime
列から月と年を抽出するもう 1つの簡単な方法は、pandas.DatetimeIndex
クラスのオブジェクトの年と月の属性の値を取得することです。
import pandas as pd
import numpy as np
import datetime
list_of_dates = ["2019-11-20", "2020-01-02", "2020-02-05", "2020-03-10", "2020-04-16"]
employees = ["Hisila", "Shristi", "Zeppy", "Alina", "Jerry"]
df = pd.DataFrame({"Joined date": pd.to_datetime(list_of_dates)}, index=employees)
df["year"] = pd.DatetimeIndex(df["Joined date"]).year
df["month"] = pd.DatetimeIndex(df["Joined date"]).month
print(df)
出力:
Joined date Year Month
Hisila 2019-11-20 2019 11
Shristi 2020-01-02 2020 1
Zeppy 2020-02-05 2020 2
Alina 2020-03-10 2020 3
Jerry 2020-04-16 2020 4
pandas.DatetimeIndex
クラスは、datetime64 データの不変の ndarray です。year
、month
、day
などの属性があります。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn