Pandas の列のデータ型を変更する方法
Asad Riaz
2023年1月30日
-
Pandas で列を数値に変換する
to_numeric
メソッド -
1つの型を他のデータ型に変換する
astype()
メソッド -
列のデータ型をより具体的な型に変換する
infer_objects()
メソッド
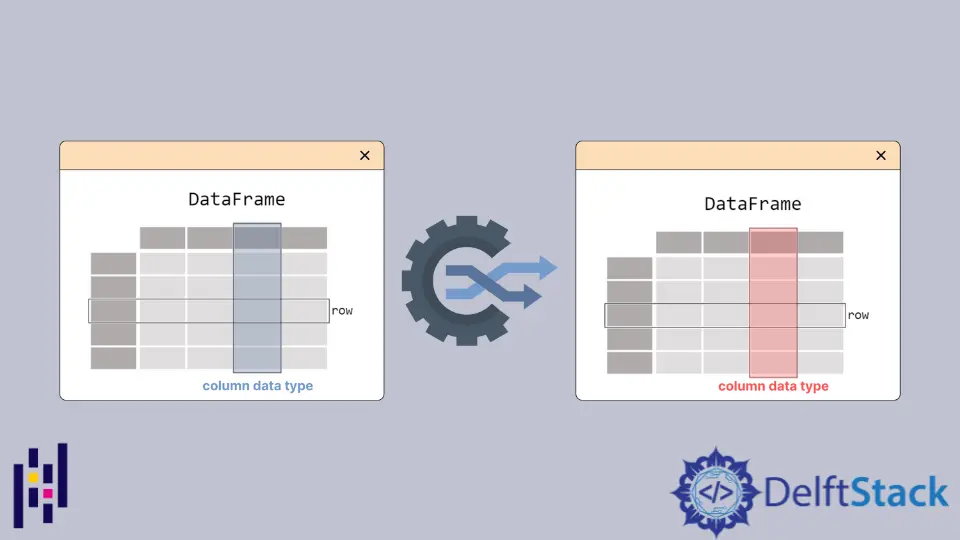
Pandas の DataFrame
の列のデータ型を変更するメソッドと、to_numaric
、as_type
、infer_objects
などのオプションを紹介します。また、to_numaric
で downcasting
オプションを使用する方法についても説明します。
Pandas で列を数値に変換する to_numeric
メソッド
to_numeric()
は、DataFrame
の 1つ以上の列を数値に変換する最良の方法です。また、非数値オブジェクト(文字列など)を必要に応じて整数または浮動小数点数に変更しようとします。to_numeric()
入力は、Series
または dataFrame
の列にすることができます。一部の値を数値型に変換できない場合、to_numeric()
を使用すると、数値以外の値を強制的に NaN
にすることができます。
コード例:
# python 3.x
import pandas as pd
s = pd.Series(["12", "12", "4.7", "asad", "3.0"])
print(s)
print("------------------------------")
print(pd.to_numeric(s, errors="coerce"))
出力:
0 12
1 12
2 4.7
3 asad
4 3.0
dtype: object0 12.0
1 12.0
2 4.7
3 NaN
4 3.0
dtype: float64
to_numeric()
はデフォルトで int64
または float64
dtype のいずれかを提供します。オプションを使用して、integer
、signed
、unsigned
、または float
のいずれかにキャストできます。
# python 3.x
import pandas as pd
s = pd.Series([-3, 1, -5])
print(s)
print(pd.to_numeric(s, downcast="integer"))
出力:
0 -3
1 1
2 -5
dtype: int64
0 -3
1 1
2 -5
dtype: int8
1つの型を他のデータ型に変換する astype()
メソッド
astype()
メソッドを使用すると、変換する dtype を明示的に指定できます。astype()
メソッド内でパラメーターを渡すことで、あるデータ型から別のデータ型に変換できます。
次のコードについて考えます。
# python 3.x
import pandas as pd
c = [["x", "1.23", "14.2"], ["y", "20", "0.11"], ["z", "3", "10"]]
df = pd.DataFrame(c, columns=["first", "second", "third"])
print(df)
df[["second", "third"]] = df[["second", "third"]].astype(float)
print("Converting..................")
print("............................")
print(df)
出力:
first second third
0 x 1.23 14.2
1 y 20 0.11
2 z 3 10
Converting..................
............................
first second third
0 x 1.23 14.20
1 y 20.00 0.11
2 z 3.00 10.00
列のデータ型をより具体的な型に変換する infer_objects()
メソッド
dataFrame
の列をより特定のデータ型に変換するための Pandas のバージョン 0.21.0 から導入された infer_objects()
メソッド(ソフト変換)。
次のコードを検討してください。
# python 3.x
import pandas as pd
df = pd.DataFrame({"a": [3, 12, 5], "b": [3.0, 2.6, 1.1]}, dtype="object")
print(df.dtypes)
df = df.infer_objects()
print("Infering..................")
print("............................")
print(df.dtypes)
出力:
a object
b object
dtype: object
Infering..................
............................
a int64
b float64
dtype: object