PowerShell で CmdletBinding 属性を使用する方法
-
PowerShell の
CmdletBinding
属性 -
Verbose
パラメーターと共にCmdletBinding
属性を使用する -
$PSCmdlet
オブジェクトとSupportsShouldProcess
と共にCmdletBinding
属性を使用する -
Parameter
属性を使って関数パラメーターを制御するためにCmdletBinding
属性を使用する
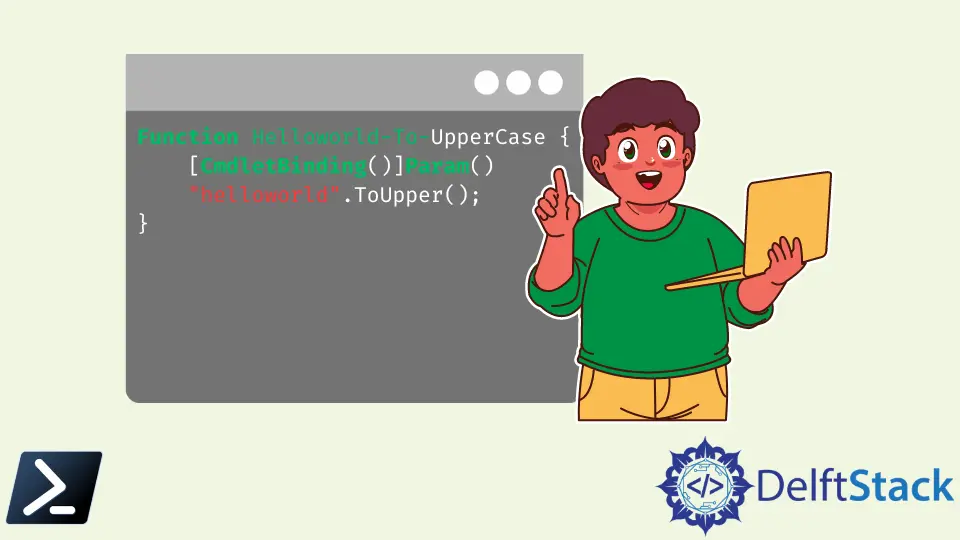
cmdlet
は、PowerShell 環境内で単一の機能を実行する軽量スクリプトです。cmdlet
は、任意の .Net
言語で記述することができます。
通常、cmdlet
はコマンドを実行するために動詞-名詞のペアで表現されます。このコマンドは、エンドユーザーによって特定のサービスを実行するように基礎となるオペレーティングシステムへの命令です。
PowerShell 環境には、New-Item
、Move-Item
、Set-Location
、Get-Location
など、200 を超える基本的な cmdlet
が含まれています。cmdlet
は、単純な PowerShell 関数では利用できない共通の機能セットを共有しています。
-WhatIf
、ErrorAction
、Verbose
などの共通パラメーターをサポート- 確認を求めるプロンプト
- 必須パラメーターのサポート
PowerShell の CmdletBinding
属性
単純な PowerShell 関数は、上記の基本的な cmdlet
機能を継承することによって、高度な関数として記述することができます。CmdletBinding
属性を使用すると、これらの基本的な cmdlet
機能にアクセスできます。
以下は、すべての可能な引数を含む CmdletBinding
属性の構文を示しています。
{
[CmdletBinding(ConfirmImpact = <String>,
DefaultParameterSetName = <String>,
HelpURI = <URI>,
SupportsPaging = <Boolean>,
SupportsShouldProcess = <Boolean>,
PositionalBinding = <Boolean>)]
Param ($myparam)
Begin {}
Process {}
End {}
}
簡単な PowerShell 関数 Helloworld-To-UpperCase
があるとしましょう。
Function Helloworld-To-UpperCase {
"helloworld".ToUpper();
}
この関数にはパラメーターが付いていません。したがって、これは単純な PowerShell 関数と呼ばれます。
しかし、CmdletBinding
属性を使用して、この関数を高度なものに変換し、基本的な cmdlet
機能とパラメーターにアクセスできるようにできます。次のように示されます。
Function Helloworld-To-UpperCase {
[CmdletBinding()]Param()
"helloworld".ToUpper();
}
上記の関数を -verbose
パラメーターで呼び出すと、すべての Write-Verbose
文字列が PowerShell ウィンドウに印刷されます。
HelloWorld-To-UpperCase -Verbose
出力:
$PSCmdlet
オブジェクトと SupportsShouldProcess
と共に CmdletBinding
属性を使用する
CmdletBinding
属性を使用しているため、高度な関数は $PSCmdlet
オブジェクトに手間なくアクセスできます。このオブジェクトには、ShouldContinue
、ShouldProcess
、ToString
、WriteDebug
などのいくつかのメソッドが含まれています。
ShouldContinue
メソッドと共に CmdletBinding
属性を使用する
このメソッドは、ユーザーが確認リクエストを処理できるようにします。その間、SupportsShouldProcess
引数を $True
に設定することが必須です。
ShouldContinue
メソッドにはいくつかのオーバーロードされたメソッドがあり、私たちは二つのパラメーターを持つものを使用します。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]Param()
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
"helloworld".ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
-Confirm
パラメーターで関数を呼び出すと、次のように確認ボックスが表示されます。
HelloWorld-To-UpperCase -Confirm
出力:
ユーザーが Yes
をクリックすると、if
ブロック内のメソッドが実行され、helloworld
文字列が大文字で印刷されるはずです。
そうでない場合は、helloworld kept in lowercase
メッセージが表示されます。
Parameter
属性を使って関数パラメーターを制御するために CmdletBinding
属性を使用する
私たちの高度な関数が文字列として 1つのパラメーターを取るようにしましょう。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]
Param([string]$word)
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
私たちは Helloworld-To-UpperCase
関数を、$word
という名前の文字列型の 1つのパラメーターを取るように変更しました。この関数が呼び出されるとき、文字列を引数として指定する必要があります。
提供されたテキストは大文字に変換されます。ユーザーがテキスト引数を提供しなかった場合、関数は空の出力を与えます。
私たちは $word
パラメーターを必須にして位置を 0
に指定することによって、これを制御できます。
Function Helloworld-To-UpperCase {
[CmdletBinding(SupportsShouldProcess = $True)]
Param(
[Parameter(
Mandatory = $True, Position = 0
) ]
[string]$word
)
#Verbose
Write-Verbose "This is the common parameter usage -Version within our Helloworld-To-UpperCase function"
#If/Else block for request processing
if ($PSCmdlet.ShouldContinue("Are you sure on making the helloworld all caps?", "Making uppercase with ToUpper")) {
$word.ToUpper();
}
Else {
"helloworld kept in lowercase."
}
}
私たちは $word
パラメーターの動作を制御するためにいくつかのフラグを追加しました。必須であるため、関数が実行されるときに文字列の値を指定する必要があります。
HelloWorld-To-UpperCase -Confirm "stringtouppercase"
PowerShell は、テキスト引数を提供しないと何度も要求します。
あなたは、以下の Parameter
属性の構文に示されているように、関数内のパラメーターを制御するためにいくつかのフラグを使用できます。
Param
(
[Parameter(
Mandatory = <Boolean>,
Position = <Integer>,
ParameterSetName = <String>,
ValueFromPipeline = <Boolean>,
ValueFromPipelineByPropertyName = <Boolean>,
ValueFromRemainingArguments = <Boolean>,
HelpMessage = <String>,
)]
[string[]]
$Parameter1
)
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.