Windows PowerShell でコマンドライン引数を取得する方法
- PowerShell スクリプトでのパラメータの定義
- PowerShell スクリプトでの名前付きパラメータ
- PowerShell スクリプトでのパラメータへのデフォルト値の割り当て
- PowerShell スクリプトでのスイッチパラメータ
- PowerShell スクリプトでの必須パラメータ
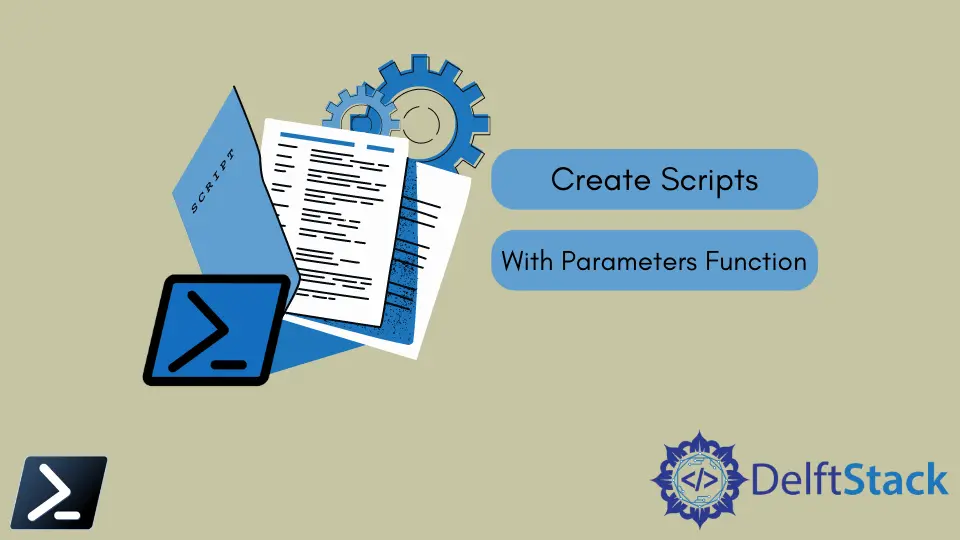
私たちは、param()
と呼ばれる Windows PowerShell パラメータ関数を使用して引数を処理します。Windows PowerShell パラメータ関数は、任意のスクリプトの基本的なコンポーネントです。パラメータは、開発者がスクリプトユーザーに実行時に入力を提供させる方法です。
この記事では、パラメータ関数を使用してスクリプトを作成し、それを使用する方法、およびパラメータを構築するためのいくつかのベストプラクティスについて学びます。
PowerShell スクリプトでのパラメータの定義
管理者は、パラメータ関数 param()
を使用してスクリプトや関数のためのパラメータを作成できます。関数には、変数によって定義された 1つ以上のパラメータが含まれています。
Hello_World.ps1
:
param(
$message
)
ただし、パラメータが必要なタイプの入力のみを受け付けることを保証するために、ベストプラクティスでは、パラメータブロック [Parameter()]
を使用してパラメータにデータ型を割り当て、変数の前に角括弧 []
でデータ型を囲むことを指示します。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message
)
上記の例 Hello_World.ps1
では、変数 message
は、与えられた値が String
というデータ型を持っている場合にのみ、渡された値を受け入れます。
PowerShell スクリプトでの名前付きパラメータ
スクリプトでパラメータ関数を使用する方法の 1つは、パラメータ名を使用することです。この方法は名前付きパラメータと呼ばれます。名前付きパラメータを介してスクリプトまたは関数を呼び出す場合、変数名をパラメータのフルネームとして使用します。
この例では、Hello_World.ps1
を作成し、パラメータ関数内に変数を定義しました。パラメータ関数内に 1つ以上の変数を置くことができることを覚えておいてください。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion
)
Write-Output $message
Write-Output "I am $emotion"
その後、.ps1
ファイルを実行するときに引数として名前付きパラメータを使用できます。
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy'
出力:
Hello World!
I am happy
PowerShell スクリプトでのパラメータへのデフォルト値の割り当て
スクリプト内でパラメータに値を割り当てることで、前もってパラメータに値を割り当てることができます。そして、実行ラインから値を渡さずにスクリプトを実行すると、スクリプト内で定義された変数のデフォルト値が取得されます。
param(
[Parameter()]
[String]$message = "Hello World",
[String]$emotion = "happy"
)
Write-Output $message
Write-Output "I am $emotion"
.\Hello_World.ps1
出力:
Hello World!
I am happy
PowerShell スクリプトでのスイッチパラメータ
使用できる別のパラメータは、[switch]
データ型によって定義されたスイッチパラメータです。スイッチパラメータは、true
または false
を示すためにバイナリまたはブール値に使用されます。
Hello_World.ps1
:
param(
[Parameter()]
[String]$message,
[String]$emotion,
[Switch]$display
)
if ($display) {
Write-Output $message
Write-Output "I am $emotion"
}
else {
Write-Output "User denied confirmation."
}
以下の構文を使用して、スクリプトパラメータでスクリプトを呼び出すことができます。
.\Hello_World.ps1 -message 'Hello World!' -emotion 'happy' -display:$false
出力:
User denied confirmation.
PowerShell スクリプトでの必須パラメータ
スクリプトが実行されるときに使用しなければならない 1つ以上のパラメータがあることは一般的です。パラメータブロック [Parameter()]
内に Mandatory
属性を追加することで、パラメータを必須として設定できます。
Hello_World.ps1:
param(
[Parameter(Mandatory)]
[String]$message,
[String]$emotion,
[Switch]$display
)
if ($display) {
Write-Output $message
Write-Output "I am $emotion"
}
else {
Write-Output "User denied confirmation."
}
Hello_World.ps1
を実行すると、Windows PowerShell はスクリプトの実行を許可せず、値を求めるメッセージを表示します。
.\Hello_World.ps1
出力:
cmdlet hello_world.ps1 at command pipeline position 1
Supply values for the following parameters:
message:
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn