Linux で PHP を使用してユーザー クォータを返す
-
crontab -e
を使用して Linux OS の PHP でユーザー クォータを返す -
repquota -a
コマンドを使用して Linux OS の PHP でユーザー クォータを返す -
du -bs
コマンドを使用して Linux OS の PHP でユーザー クォータを返す - MySQL クォータを使用して Linux OS の PHP でユーザー クォータを返す
-
imap_get_quota
関数を使用して Linux OS の PHP でユーザー クォータを返す
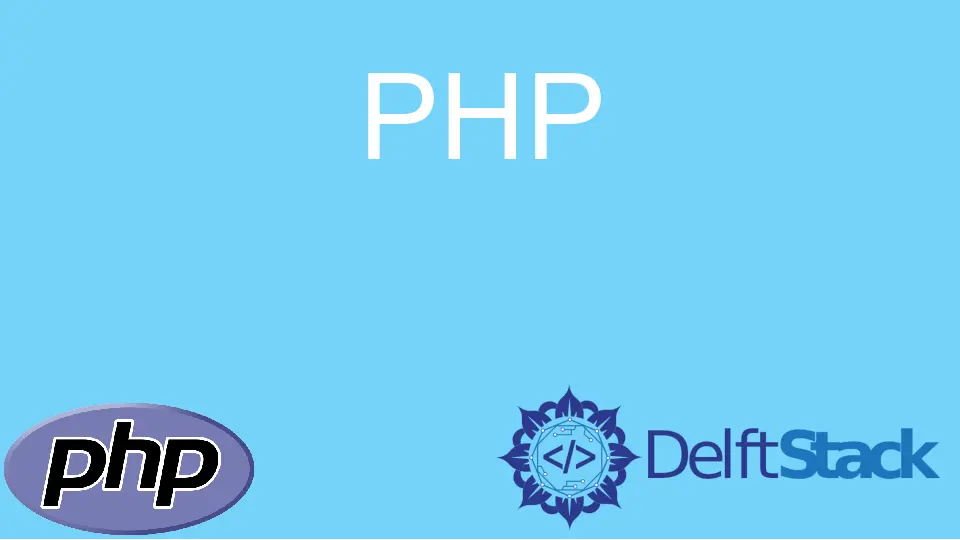
ユーザー クォータは、Linux OS で特定のユーザーのディスク使用量と制限を表示するための用語です。 このチュートリアルでは、Linux オペレーティング システムで PHP を使用してユーザーにクォータを取得または返すさまざまな方法を学習します。
デフォルトでは、サーバーはすべてのファイルシステムの他のクォータレポートが/etc/mtab
にリストされているため、ユーザークォータのみを出力することを許可されています。サーバーマシンでrpc.rquotad
を呼び出して、ファイルシステムの情報を取得できます。 NFS マウントされています。
クォータがゼロ以外のステータスで終了する場合、1つまたは複数のファイルシステムをオーバークォータする方法を学ぶことが不可欠です。ほとんどの場合、デフォルトのファイルシステムは /etc/mtab
および quota.user
または quota.group
クォータにあります。 ファイルシステムのルートにあるファイル。
クォータはユーザーを意図しない乱用から保護し、データの流出を最小限に抑え、ユーザー リソースを過剰な使用から保護できるため、ユーザーによる管理、更新、または表示のためにクォータを返すことが重要であり、ディスク容量または ユーザーが使用またはアクセスできるファイルの数。
クォータは、特定のユーザーが使用するディスク容量とファイル数を制限または追跡するために不可欠であり、特定のボリュームまたはqtree
に適用されます。
PHP でユーザー クォータを返す前に、要件として SELinux
を無効にする必要があるシナリオである可能性があり、[root@managed2 ~]# setenforce 0
のような方法でこれを行うことができます。
crontab -e
を使用して Linux OS の PHP でユーザー クォータを返す
考慮すべき重要なことは、ユーザー (PHP コードを制御するユーザー) は、外部コマンドを実行して Linux OS でユーザー クォータを抽出できないということです。
アクセスを取得したり、システムによるユーザー クォータを取得するための制限を解除したりするために、ユーザーはサーバーのセットアップに従って PHP の構成を変更できます。 共有ホスティングであり、PHP 呼び出しを介してプロバイダーと通信してアクセスを取得する必要があります。
外部の cron ジョブで .txt
ファイルにクォータを収集することで、PHP の制限を簡単に回避できますが、これは、cron ジョブを設定する権限を持っているか、サーバーへの適切なアクセス権を持つユーザーに対してのみ機能します。
PHP で crontab -e
コマンドを使用して、Web サーバーでルート アクセス権と読み取り可能なディレクトリを取得し、ユーザー _username
のユーザー クォータを Web サーバー フォルダーにある quota.txt
テキスト ファイルに保存できます。 時間。
読み取り可能な形式が G
ではないユーザー ケースでこのアプローチを採用するには、-s
を削除してバイトを解析し、PHP で人間が読み取れる形式に変換します。
ユーザーは、cronjob
が毎時であることを理解する必要があります。また、ユーザー クォータにアクセスするたびに、ユーザー クォータ情報のリアルタイム統計が含まれず、矛盾が含まれる可能性があることを理解する必要があります。 ただし、cron
の頻度を増やして Web サーバーのクォータ ファイルを頻繁に更新することで回避できます。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<?php
// use `echo "The time is " . date("h:i:sa");` to implement cronjob in your PHP project
/* Get User Quota Information
*
* add `0 * * * * quota -u -s someusername | grep /dev > /path/to/webserver/quota.txt`
* to save quota setting
*
* prepare user account and array the disk space information by ascertaining the available space on its quota
* and the space used and the space free to use by a specific user
*
* @param string $_user The system user name
*
* @return array
*/
function get_QuotaInfo_User($_user) {
$user_quota = exec("quota -s -u ".$_user); // query server
$user_quota = preg_replace("/[\s-]+/", ' ', $user_quota); // clear spaces
$arr = explode(' ', $user_quota);
$_free_space = str_replace('M', '', $arr[3]) - str_replace('M', '', $arr[2]);
return array(
"total" => $arr[3],
"used" => $arr[2],
"free" => $_free_space.'M'
);
}
/*
* you can write this in your php project to return or show the file containing the user quota
$user_quota_file_txt = file_get_contents("quota.txt");
$user_quota_array = explode(" ",$user_quota_file_txt);
function user_quota_exe($str){
return intval(substr_replace($str, "", -1));
}
echo "total quota: ". $user_quota_array[4]."<br>";
echo "used: ". $user_quota_array[3]."<br>";
$free =(user_quota_exe($user_quota_array[4]))-user_quota_exe($user_quota_array[3]);
echo "free: " . $free . "G";
*/
?>
</body>
</html>
出力:
* display text from the `quota.txt` file
repquota -a
コマンドを使用して Linux OS の PHP でユーザー クォータを返す
これは、ストレージ使用量に関するユーザー クォータ レポートを返す Linux コマンドであり、これを PHP で採用できます。 ただし、クォータを編集するための主要なコマンドはedquota
であり、/etc/fstab
などの毎日の重要なクォータ ファイルを編集できます。
コマンド yum install quota
を発行して quota
ツールをインストールし、必要な依存関係を受け入れて、インストールを完了させます。 その後、quotacheck -cu /home
を使用してデータベース ファイルを作成し、このコマンドを再度実行して、-c
を -av
に置き換えます (新しいコマンドは quotacheck -avu
のようになります)。 ローカルにマウントされ、クォータが有効になっているすべてのパーティションをチェックすると、-v
は詳細な出力を使用します。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<?php
function execCommand($command, $log) {
if (substr(php_uname(), 0, 7) == "Windows")
{
//windows
// write alternative command for Windows
}
else
{
//linux
shell_exec( $command . "repquota -a" );
}
// or
/*
shell_exec( $command . "repquota -a" ); // just use this line in your code
*/
return false;
}
?>
</body>
</html>
出力:
* it will run the `repquota -a` command in PHP code and show the user quota
MySQL および PHP ホスティングを共有する Linux サーバーへの非特権アクセス権を持つユーザーは、sudo -u
コマンドを実行してユーザー クォータを取得できます。 sudoers
ファイルでこの権限を付与し、権限のないユーザーとしてこのコマンドを実行できます。
# repquota -a
および # repquota /home
と同様に、構成されたすべてのクォータと特定のパーティションのクォータをそれぞれ表示します。 さらに、# quota -u user
や # quota -g group
などの Linux コマンドを使用して、特定のユーザーまたはユーザー グループに適用されるクォータを表示できます。
du -bs
コマンドを使用して Linux OS の PHP でユーザー クォータを返す
PHP はクォータを出力できないため、Linux コマンドを活用する簡単な方法が必要です。explode
を使用することは、文字列を文字列で分割して、PHP で文字列を文字列の配列に変換する完璧な例です。 ただし、crontable を使用してクォータを /temp
に出力し、$var = exec("cat /tmp/quotas | grep domz | tail -n 1 | awk '{print $4}'") のような方法で取得できます。 ;
PHPで。
さらに、MySQL を使用してユーザー クォータを返すには、別のアプローチが必要です。 各データベースのサイズをチェックし、指定されたサイズ制限を超えるデータベースの INSERT
および CREATE
権限を取り消すことによって機能します。
クォータはユーザーベースではなくデータベースであるため、グローバル権限を持つユーザーには機能しませんが、ほとんどの環境では、MySQL を使用して PHP で変更できる "db" テーブル
で権限が与えられます。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<?php
$conn_server = $db_host;
$conn_user = $db_user;
$user_passwrd = $db_pass;
$conn_database = "root_cwp";
// Create a connection
$conn = new mysqli($conn_server, $conn_user, $user_passwrd, $conn_database);
class user_quota_spaceused
{
private $conn;
public function __construct($conn)
{
$this->conn = $conn;
}
public function calculate()
{
$quota['home'] = $this->user_homeQuota();
$quota['mysql'] = $this->user_MySQL_Quota();
return $quota;
}
public function user_homeQuota()
{
$user_quota = shell_exec("du -bs /home/*");
$userQuota_all = explode("\n", $user_quota);
$quota = [];
foreach ($userQuota_all as $user_homeQuota_info) {
if (!empty($user_homeQuota_info)) {
$user_quotaInfo = explode('/', trim($user_homeQuota_info), 2);
$conn_user = trim(str_replace('home/', '', $user_quotaInfo[1]));
$user_quota = trim($user_quotaInfo[0]);
$quota[$conn_user] = (int) $user_quota;
}
}
return $quota;
}
public function user_MySQL_Quota()
{
$com_getallQuota = shell_exec("du -bs /var/lib/mysql/*");
$userQuota_rows = explode("\n", $com_getallQuota);
$quota = [];
foreach ($userQuota_rows as $infoQuota_row) {
if (!empty($infoQuota_row)) {
$quotaInfo_user = explode('/', trim($infoQuota_row), 2);
$userQuota_file = trim($quotaInfo_user[0]);
$database_name = trim(str_replace('var/lib/mysql/', '', $quotaInfo_user[1]));
$explodedDatabase_name = explode('_', trim($database_name), 2);
$conn_quotaUser = $explodedDatabase_name[0];
if (isset($explodedDatabase_name[1])) {
$conn_user_database = $explodedDatabase_name[1];
};
if (isset($quota[$conn_quotaUser])) {
if (isset($conn_user_database)) {
$quota[$conn_quotaUser]['db'][$conn_user_database] = (int) $userQuota_file;
}
$quota[$conn_quotaUser]['db_quota'] += (int) $userQuota_file;
$quota[$conn_quotaUser]['db_count']++;
}
else
{
if (isset($conn_user_database)) {
$quota[$conn_quotaUser]['db'][$conn_user_database] = (int) $userQuota_file;
}
$quota[$conn_quotaUser]['db_quota'] = (int) $userQuota_file;
$quota[$conn_quotaUser]['db_count'] = 1;
}
unset($conn_user_database);
}
}
return $quota;
}
}
$cwpUsersQuota = new user_quota_spaceused($conn);
$quota = $cwpUsersQuota->calculate();
?>
</body>
</html>
出力:
* gets user home and MySQL quota info and shows the user quota info on a webpage table
MySQL クォータを使用して Linux OS の PHP でユーザー クォータを返す
この C++ プログラムは、無料の再配布可能なソフトウェアを使用して、特定のユーザーの MySQL クォータ スクリプトを取得するプロセスを説明しています。詳細については、GNU 一般ライセンスを参照してください。 CREATE TABLE
と Db
および Limit
変数を使用して、MySQL クォータ スクリプト用の Quota
テーブルを作成します。
フィールド Db
にはサイズを制限するデータベースの情報が格納され、フィールド Limit
はユーザーごとのサイズ制限 (バイト単位) です。 さらに、exceeded
(Exceeded
ENUM (Y
,N
) DEFAULT N
NOT NULL, PRIMARY KEY (Db
), UNIQUE (Db
)) は内部でのみ使用され、初期化する必要があります。 N
で。
#!/user/bin/am -q
<?PHP
/*
* Settings
*/
$host_mysql = 'localhost';
// do not change the `$nysql_user` because root access is required
$user_mysql = 'root';
$pass_mysql = '';
// to check not just the Db but the Db with the user quota table
$database_mysql = 'quotadb';
$table_mysql = 'quota';
/*
* it is restricted to change anything below without a proper understanding
*/
$conn_debug = 0;
// connection to MySQL server
if (!mysql_connect($host_mysql, $user_mysql, $pass_mysql))
{
echo "Connection to MySQL-server failed!";
exit;
}
// database selection process
if (!mysql_select_db($database_mysql))
{
echo "Selection of database $database_mysql failed!";
exit;
}
// to check the quota in each entry of the quota table from the selected database
$query_mysql = "SELECT * FROM $table_mysql;";
$result = mysql_query($query_mysql);
while ($db_row = mysql_fetch_array($result))
{
$database_quota = $db_row['db'];
$limit_userquota = $db_row['limit'];
$exceeded_quota = ($db_row['exceeded']=='Y') ? 1 : 0;
if ($conn_debug){
echo "Checking quota for '$database_quota'...
";
}
$show_mysqlQuery = "SHOW TABLE STATUS FROM $database_quota;";
$query_result = mysql_query($show_mysqlQuery);
if ($conn_debug){
echo "SQL-query is `$show_mysqlQuery`
";
}
$size_userQuota = 0;
while ($query_row = mysql_fetch_array($query_result))
{
if ($conn_debug)
{
echo "Result of query:
"; var_dump($query_row);
}
$size_userQuota += $query_row['Data_length'] + $query_row['Index_length'];
}
if ($conn_debug){
echo "Size is $size_userQuota bytes, limit is $limit_userquota bytes
";
}
if ($conn_debug && $exceeded_quota){
echo "Quota is marked as exceeded.
";
}
if ($conn_debug && !$exceeded_quota){
echo "Quota is not marked as exceeded.
";
}
if (($size_userQuota > $limit_userquota) && !$exceeded_quota)
{
if ($conn_debug){
echo "Locking database...
";
}
// save the information in the quota table
$user_infoQuery = "UPDATE $table_mysql SET exceeded='Y' WHERE db='$database_quota';";
mysql_query($user_infoQuery);
if ($conn_debug)
{
echo "Querying: $user_infoQuery
";
}
// dismissing the CREATE and INSERT privileges for the database
mysql_select_db('mysql');
$user_infoQuery = "UPDATE db SET Insert_priv='N', Create_priv='N' WHERE Db='$database_quota';";
mysql_query($user_infoQuery);
if ($conn_debug)
{
echo "Querying: $user_infoQuery
";
}
mysql_select_db($database_mysql);
}
if (($size_userQuota <= $limit_userquota) && $exceeded_quota)
{
if ($conn_debug){
echo "Unlocking database...
";
}
// to save info in the user quota table
$user_infoQuery = "UPDATE $table_mysql SET exceeded='N' WHERE db='$database_quota';";
mysql_query($user_infoQuery);
if ($conn_debug){
echo "Querying: $user_infoQuery
";
}
// granting the CREATE and INSERT privileges for the database
mysql_select_db('mysql');
$user_infoQuery = "UPDATE db SET Insert_priv='Y', Create_priv='Y' WHERE Db='$database_quota';";
mysql_query($user_infoQuery);
if ($conn_debug){
echo "Querying: $user_infoQuery
";
}
mysql_select_db($database_mysql);
}
}
?>
出力:
* Select the MySQL user quota from the MySQL database
imap_get_quota
関数を使用して Linux OS の PHP でユーザー クォータを返す
これは、管理者ユーザーがメールボックスごとのユーザー クォータ設定またはクォータ使用統計を取得するのに役立ちます。 imap_get_quota
関数は管理ユーザー用であり、このグループの非管理ユーザー バージョンは imap_get_quotaroot()
であり、同様の目的で使用できます。
この関数を利用して、IMAP
または Connection
インスタンスの $imap
と、メールボックスの user.name
の形式の名前として $quota_root
を含めます。 最終的な関数は imap_get_quota(IMAP\Connection $imap, string $quota_root): array|false
のようになり、指定されたユーザーのストレージ制限と使用量を含む整数配列を返します。
この関数からの戻り値は、特定のメールボックスまたはユーザーに許可されている合計容量 (limit
で表される) と、ユーザーの現在の容量または容量使用量 (usage
で表される) を表します。 ユーザーまたはメールボックスの情報が正しくない場合、またはユーザー クォータから容量使用情報を取得できなかった場合は、false
を返し、エラーを表示します。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<?php
$userQuota_checkMail = imap_open("{imap.example.org}", "admin_mailaddress", "your_password", OP_HALFOPEN)
or die("Caution! can't connect to the server: " . imap_last_error());
$accessuserQuota_checkValue = imap_get_quota($userQuota_checkMail, "user.hassankazmi");
if (is_array($accessuserQuota_checkValue))
{
echo "User's usage level: " . $accessuserQuota_checkValue['usage'];
echo "User's storage limit: " . $accessuserQuota_checkValue['limit'];
}
imap_close($userQuota_checkMail);
?>
// use the following PHP example for `imp_get_quota` 4.3 or greater
/*
$userQuota_checkMail = imap_open("{imap.example.org}", "admin_mailadmin", "your_password", OP_HALFOPEN)
or die("Caution! can't connect to the server: " . imap_last_error());
$accessuserQuota_checkValue = imap_get_quota($userQuota_checkMail, "user.hassankazmi");
if (is_array($accessuserQuota_checkValue))
{
$user_storageQuota_info = $accessuserQuota_checkValue['STORAGE'];
echo "User's usage level: " . $user_storageQuota_info['usage'];
echo "User's storage limit: " . $user_storageQuota_info['limit'];
$user_storageQuota_message = $accessuserQuota_checkValue['MESSAGE'];
echo "User's MESSAGE usage level: " . $user_storageQuota_message['usage'];
echo "User's MESSAGE limit: " . $user_storageQuota_message['limit'];
/* ... */
}
imap_close($userQuota_checkMail);
*/
</body>
</html>
出力:
* user quota info from the mailbox
User's usage level: 32.66 GB
User's storage limit: 60 GB
下位互換性のために、PHP の元のユーザー クォータ アクセスまたはリターン メソッドを使用できます。 PHP 4.3 以降、imap_get_quota
関数は c-client2000
以降のライブラリのユーザーのみが利用できます。 これは、ユーザー管理者がユーザーのために imap
を開くときにのみ役立つ機能です。 そうしないと、機能しません。
スクリプトの終了後、imap
関数の使用中に Notice: Unknown
エラー (Quota root does not exist (errflg=2)
または同様のエラー) が発生することに注意することが重要です。 imap
ストリームを閉じる前に imap_error()
関数を呼び出して、エラー スタックをクリアし、エラー通知の取得を停止します。
さらに、この機能が動作するには、IMAP サーバーにgetquota
機能が必要です。これは、telnet <メール サーバー> <ポート>
を使用して直接ログインすることで確認できます。 たとえば、telnet mail.myserver.com 143
を使用すると、0 CAPABILITY
のようなものが表示されます。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub