PHP で HTML を解析する
-
PHP で
DomDocument()
を使用して HTML を解析する -
PHP で
simplehtmldom
を使用して HTML を解析する -
PHP で
DiDOM
を使用して HTML を解析する
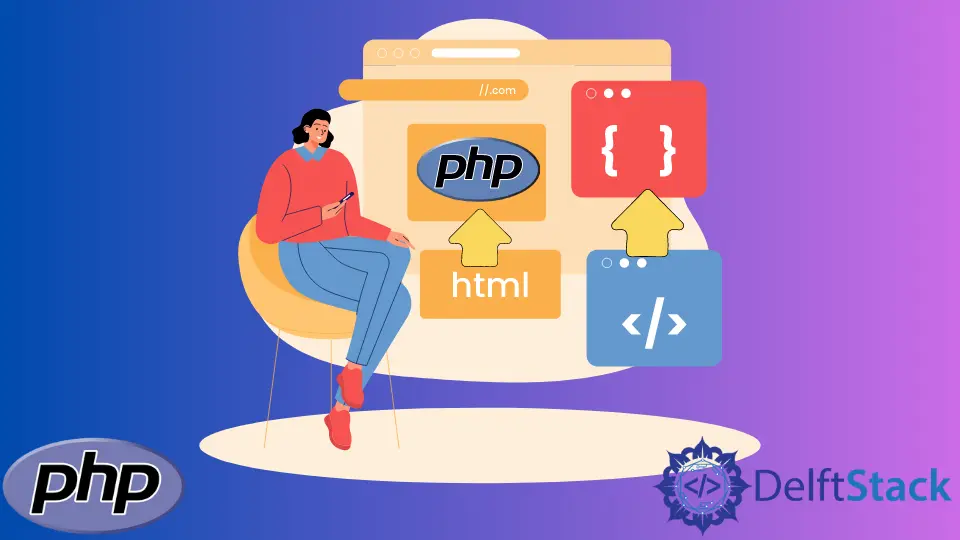
HTML を解析すると、コンテンツまたはマークアップを文字列に変換できるため、ダイナミック HTML ファイルの分析や作成が容易になります。より詳細には、生の HTML コードを取得して読み取り、段落から見出しまで DOM ツリーオブジェクト構造を生成し、重要な情報や必要な情報を抽出できるようにします。
組み込みライブラリを使用して HTML ファイルを解析し、場合によってはサードパーティのライブラリを使用して、PHP での Web スクレイピングやコンテンツ分析を行います。メソッドに応じて、目標は HTML ドキュメントの本文を文字列に変換して各 HTML タグを抽出することです。
この記事では、組み込みクラス DomDocument()
と、2つのサードパーティライブラリ simplehtmldom
と DiDOM
について説明します。
PHP で DomDocument()
を使用して HTML を解析する
ローカル HTML ファイルであろうとオンライン Web ページであろうと、DOMDocument()
クラスと DOMXpath()
クラスは、HTML ファイルを解析し、その要素を文字列またはこの例の場合は配列として格納するのに役立ちます。
関数を使用してこの HTML ファイルを解析し、見出し、小見出し、および段落を返しましょう。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h2 class="main">Welcome to the Abode of PHP</h2>
<p class="special">
PHP has been the saving grace of the internet from its inception, it
runs over 70% of website on the internet
</p>
<h3>Understanding PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Using PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Install PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h3>Configure PHP</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
<h2 class="main">Welcome to the Abode of JS</h2>
<p class="special">
PHP has been the saving grace of the internet from its inception, it
runs over 70% of website on the internet
</p>
<h3>Understanding JS</h3>
<p>
Lorem ipsum dolor, sit amet consectetur adipisicing elit. Eum minus
eos cupiditate earum et optio culpa, eligendi facilis laborum
dolore.
</p>
</body>
</html>
PHP コード:
<?php
$html = 'index.html';
function getRootElement($element, $html)
{
$dom = new DomDocument();
$html = file_get_contents($html);
$dom->loadHTML($html);
$dom->preserveWhiteSpace = false;
$content = $dom->getElementsByTagName($element);
foreach ($content as $each) {
echo $each->nodeValue;
echo "\n";
}
}
echo "The H2 contents are:\n";
getRootElement("h2", $html);
echo "\n";
echo "The H3 contents are:\n";
getRootElement("h3", $html);
echo "\n";
echo "The Paragraph contents include\n";
getRootElement("p", $html);
echo "\n";
コードスニペットの出力は次のとおりです。
The H2 contents are:
Welcome to the Abode of PHP
Welcome to the Abode of JS
The H3 contents are:
Understanding PHP
Using PHP
Install PHP
Configure PHP
Understanding JS
The Paragraph contents include
PHP has been the saving grace of the internet from its inception, it
runs over 70% of the website on the internet
...
PHP で simplehtmldom
を使用して HTML を解析する
CSS スタイルセレクターなどの追加機能については、Simple HTML DOM Parserと呼ばれるサードパーティのライブラリを使用できます。これは、シンプルで高速な PHP パーサーです。ダウンロードして、単一の PHP ファイルを含めるか要求することができます。
このプロセスを使用すると、必要なすべての要素を簡単に解析できます。前のセクションと同じコードスニペットを使用して、str_get_html()
と呼ばれる関数を使用して HTML を解析します。この関数は、HTML を処理し、find()
メソッドを使用して特定の HTML 要素またはタグを検索します。
特別な class
を持つ要素を見つけるには、各 find
要素に適用する class
セレクターが必要です。また、実際のテキストを見つけるには、要素で innertext
セレクターを使用する必要があります。これを配列に格納します。
前のセクションと同じ HTML ファイルを使用して、simplehtmldom
を使用してファイルを解析してみましょう。
<?php
require_once('simple_html_dom.php');
function getByClass($element, $class)
{
$content= [];
$html = 'index.html';
$html_string = file_get_contents($html);
$html = str_get_html($html_string);
foreach ($html->find($element) as $element) {
if ($element->class === $class) {
array_push($heading, $element->innertext);
}
}
print_r($content);
}
getByClass("h2", "main");
getByClass("p", "special");
コードスニペットの出力は次のとおりです。
Array
(
[0] => Welcome to the Abode of PHP
[1] => Welcome to the Abode of JS
)
Array
(
[0] => PHP has been the saving grace of the internet from its inception, it runs over 70% of the website on the internet
[1] => PHP has been the saving grace of the internet from its inception, it runs over 70% of the website on the internet
)
PHP で DiDOM
を使用して HTML を解析する
このサードパーティの PHP ライブラリでは、Composer と呼ばれる PHP 依存関係マネージャーを使用する必要があります。これにより、すべての PHP ライブラリと依存関係を管理できます。DiDOM
ライブラリは GitHub から利用でき、他のライブラリよりも高速でメモリ管理が可能です。
お持ちでない場合は、こちらの作曲家をインストールできます。ただし、次のコマンドは、DiDOM
ライブラリがあればプロジェクトに追加します。
composer require imangazaliev/didom
その後、以下のコードを使用できます。このコードは、find()
メソッドを使用した simplehtmldom
と同様の構造になっています。HTML 要素のコンテキストをコードで使用できる文字列に変換する text()
があります。
has()
関数を使用すると、HTML 文字列内に要素またはクラスがあるかどうかを確認し、ブール値を返します。
<?php
use DiDom\Document;
require_once('vendor/autoload.php');
$html = 'index.html';
$document = new Document('index.html', true);
echo "H3 Element\n";
if ($document->has('h3')) {
$elements = $document->find('h3');
foreach ($elements as $element) {
echo $element->text();
echo "\n";
}
}
echo "\nElement with the Class 'main'\n";
if ($document->has('.main')) {
$elements = $document->find('.main');
foreach ($elements as $element) {
echo $element->text();
echo "\n";
}
}
コードスニペットの出力は次のとおりです。
H3 Element
Understanding PHP
Using PHP
Install PHP
Configure PHP
Understanding JS
Element with the Class 'main'
Welcome to the Abode of PHP
Welcome to the Abode of JS
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn