PHP でグラフを描く
- 環境を設定する
-
PHP で
pChart
を使用して棒グラフをプロットする -
PHP で
pChart
を使用してスプラインチャートをプロットする - MySQL データベースから PHP でヒストグラムをプロットする
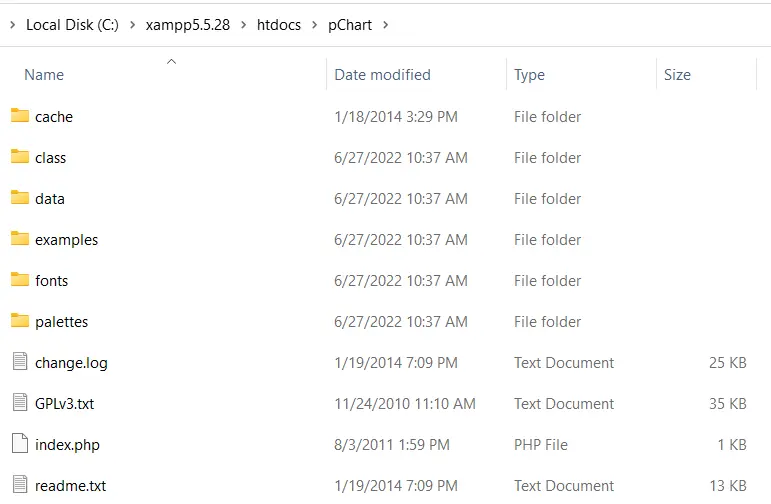
この記事では、PHP で pChart
を使用してグラフを作成する方法について説明します。1つ目は棒グラフ、2つ目はスプライングラフ、最後は MySQL のヒストグラムです。
環境を設定する
pChart
を使用する前に最初に必要なのは、PHP5 のインストールです。PHP5 は、SourceForge から XAMPP5.5.28 の一部として入手できます。
XAMPP 5.5.28 をお持ちの場合は、公式 Web サイトから pChart
をダウンロードしてください。その後、pChart
を XAMPP5.5.28 の htdocs
フォルダーに抽出します。
pChart
フォルダを開くと、その構造は次の画像のようになります。
ノート:
class
フォルダには、使用するクラス定義が含まれています。fonts
フォルダには、チャートで使用できるフォントファイルが含まれています。
pChart
の設定が完了したら、プロットを開始できます。
PHP で pChart
を使用して棒グラフをプロットする
pChart
を使用して棒グラフをプロットする PHP コードには、class
フォルダーの 3つのファイルが含まれている必要があります。これらのファイルは次のとおりです。
pData.class.php
pImage.class.php
pDraw.class.php
これらのファイルのうち、pData.class.php
を使用すると、グラフで使用するデータを読み込むことができます。グラフを描画するには、pDraw.class.php
が必要です。
次に、pImage.class.php
を使用すると、Web ブラウザでグラフをレンダリングできます。PHP required_once()
を使用してこれらのファイルを含める必要があります。
相対パスを使用してそれらを含めるか、PCHART_PATH
定数を定義できます。次に、set_include_path()
を使用して、pChart
クラスに短いディレクトリ名を使用できます。
そうは言っても、次の手順を使用して、pChart
で棒グラフを作成できます。
-
PCHART_PATH
定数を定義します。 -
pChart
クラスの短いディレクトリ名には、set_include_path()
を使用します。 -
required_once()
を使用してpChart
クラスを含めます。 -
新しい
pData
オブジェクトを作成します。 -
データを作成するか、インポートします。
-
addPoints
メソッドを使用して、pData
オブジェクトにデータを追加します。 -
pImage
のオブジェクトを使用してチャートの画像を作成します。 -
チャートのフォントを設定します。
-
pData
のsetGraphArea
メソッドを使用してグラフ領域を設定します。 -
pData
のdrawScale
およびdrawBarChart
メソッドを使用して、スケールと棒グラフを描画します。 -
ヘッダー情報を送信して、画像を送信していることをブラウザに通知します。
-
pData
のRender
メソッドを使用して画像をレンダリングします。null
をRender
メソッドに渡すようにしてください。
以下は、これらのステップの実装です。以下は、Firefox101.0 の出力イメージです。
<?php
// The definition of the PCHART_PATH assumes
// you have pChart one directory above your
// current working folder.
define("PCHART_PATH", "../pChart");
set_include_path(get_include_path() . PATH_SEPARATOR . PCHART_PATH);
// Since we have defined the path, and used
// the get_include_path() function, we can
// reference the class folder without writing
// its full path.
require_once "class/pDraw.class.php";
require_once "class/pImage.class.php";
require_once "class/pData.class.php";
// Create the pChart Object
$pchart_data = new pData();
// Some sample data that we'll use to plot
// the bar chart.
$sample_data_set = [5, 4, 3, 2, 1, 9, 10, 12];
$pchart_data->addPoints($sample_data_set);
// Create the pChart Image. The first two argument
// to the pImage object are the width and height
// of the rendered chart.
$pchart_image = new pImage(500, 300, $pchart_data);
// Set the font.
$pchart_image->setFontProperties(
["FontName" => PCHART_PATH . "/fonts/Forgotte.ttf",
"FontSize" => 16]
);
// Define the graph area. The first two arguments
// are the x-coordinates. While the last two are
// the y-coordinates.
$pchart_image->setGraphArea(35, 25, 475, 275);
$pchart_image->drawScale();
$pchart_image->drawBarChart();
// Render the chart as a PNG image
header("Content-Type: image/png");
$pchart_image->Render(null);
?>
出力:
PHP で pChart
を使用してスプラインチャートをプロットする
スプライングラフのプロットは、drawSplineChart
メソッドを使用してスプライングラフを描画することを除いて、棒グラフの場合と同じプロセスに従います。また、チャートを画像として送信しないように選択することもできます。
代わりに、pData
の Stroke
メソッドを選択して、Web ブラウザでグラフをレンダリングできます。
次のコードは、pChart
を使用してスプラインチャートを描画します。さらに、fonts
ディレクトリの MankSans.ttf
フォントを使用しています。
<?php
// The definition of the PCHART_PATH assumes
// you have pChart one directory above your
// current working folder.
define("PCHART_PATH", "../pChart");
set_include_path(get_include_path() . PATH_SEPARATOR . PCHART_PATH);
// Since we have defined the path, and used
// the get_include_path() function, we can
// reference the class folder without writing
// its full path.
require_once "class/pDraw.class.php";
require_once "class/pImage.class.php";
require_once "class/pData.class.php";
// Create the pChart Object
$pchart_data = new pData();
// Some sample data that we'll use to plot
// the spline chart.
$pchart_data->addPoints([4,2,1,4]);
// Create the pChart Image. The first two argument
// to the pImage object are the width and height
// of the rendered chart.
$pchart_image = new pImage(700, 220, $pchart_data);
// Set the font.
$pchart_image->setFontProperties(
["FontName" => PCHART_PATH . "/fonts/MankSans.ttf",
"FontSize"=> 18]
);
// Define the graph area. The first two arguments
// are the x-coordinates. While the last two are
// the y-coordinates.
$pchart_image->setGraphArea(60, 40, 670, 190);
$pchart_image->drawScale();
$pchart_image->drawSplineChart();
// Draw the chart as a stroke.
$pchart_image->Stroke();
?>
出力:
MySQL データベースから PHP でヒストグラムをプロットする
ヒストグラムのプロットは、棒グラフやスプラインチャートと同様の手順に従います。ただし、指摘する価値のあるいくつかの違いがあります。
まず、ヒストグラムのデータは MySQL から取得されます。これは、いくつかのサンプルデータを含むデータベースが必要であることを意味します。
次に、表の列名をヒストグラムの軸として使用します。このために、setAbscissa
、setSeriesOnAxis
、setAxisName
などのいくつかの pData
メソッドを使用します。
次に、weather_measurements
というデータベースを作成し、次を使用してテーブルを作成します。
CREATE TABLE measures (
timestamp INT NOT NULL DEFAULT '0',
temperature INT NOT NULL,
humidity INT NOT NULL
)
以下を使用して、サンプルデータをメジャー
テーブルに挿入します。
INSERT INTO measures (timestamp, temperature, humidity) VALUES (UNIX_TIMESTAMP(), 20, 50);
INSERT INTO measures (timestamp, temperature, humidity) VALUES (UNIX_TIMESTAMP(), 18, 44);
INSERT INTO measures (timestamp, temperature, humidity) VALUES (UNIX_TIMESTAMP(), 19, 70);
サンプルデータがデータベースにあることを確認してから、以下を使用してヒストグラムを作成します。
<?php
// The definition of the PCHART_PATH assumes
// you have pChart one directory above your
// current working folder.
define("PCHART_PATH", "../pChart");
set_include_path(get_include_path() . PATH_SEPARATOR . PCHART_PATH);
// Since we have defined the path, and used
// the get_include_path() function, we can
// reference the class folder without writing
// its full path.
require_once "class/pDraw.class.php";
require_once "class/pImage.class.php";
require_once "class/pData.class.php";
// Create the pChart Object
$pchart_data = new pData();
// Connect to MySQL
$connect_to_mysql = new mysqli("localhost", "root", "", "weather_measurements");
// query the database and get the result
$query_the_table = "SELECT * FROM measures";
$mysql_result = mysqli_query($connect_to_mysql, $query_the_table);
// Declare the variables for the database
// records as empty strings. Later, we'll
// turn them into arrays for better performance
$timestamp = ""; $temperature = ""; $humidity = "";
while($row = mysqli_fetch_array($mysql_result, MYSQLI_ASSOC)) {
$timestamp[] = $row["timestamp"];
$temperature[] = $row["temperature"];
$humidity[] = $row["humidity"];
}
$pchart_data->addPoints($timestamp,"Timestamp");
$pchart_data->addPoints($temperature,"Temperature");
$pchart_data->addPoints($humidity,"Humidity");
// Put the table column on the appropriate axis
$pchart_data->setAbscissa("Timestamp");
$pchart_data->setSerieOnAxis("Humidity", 1);
$pchart_data->setXAxisName("Time");
$pchart_data->setXAxisDisplay(AXIS_FORMAT_TIME,"H:i");
// Dedicate the first and second axis to
// Temperature and Humidity.
$pchart_data->setAxisName(0, "Temperature");
$pchart_data->setAxisUnit(0, "°C");
$pchart_data->setAxisName(1, "Humidity");
$pchart_data->setAxisUnit(0, "%");
// Create the pChart Image. The first two argument
// to the pImage object are the width and height
// of the rendered chart.
$pchart_image = new pImage(500, 300, $pchart_data);
// Set the font.
$pchart_image->setFontProperties(
["FontName" => PCHART_PATH . "/fonts/verdana.ttf",
"FontSize"=> 11]
);
// Set the graph area.
$pchart_image->setGraphArea(55,25, 475,275);
$pchart_image->drawScale();
$pchart_image->drawBarChart();
// Draw the chart as a stroke.
$pchart_image->Stroke();
?>
出力(あなたの時間は異なります):
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn