PHP での DatePicker の操作
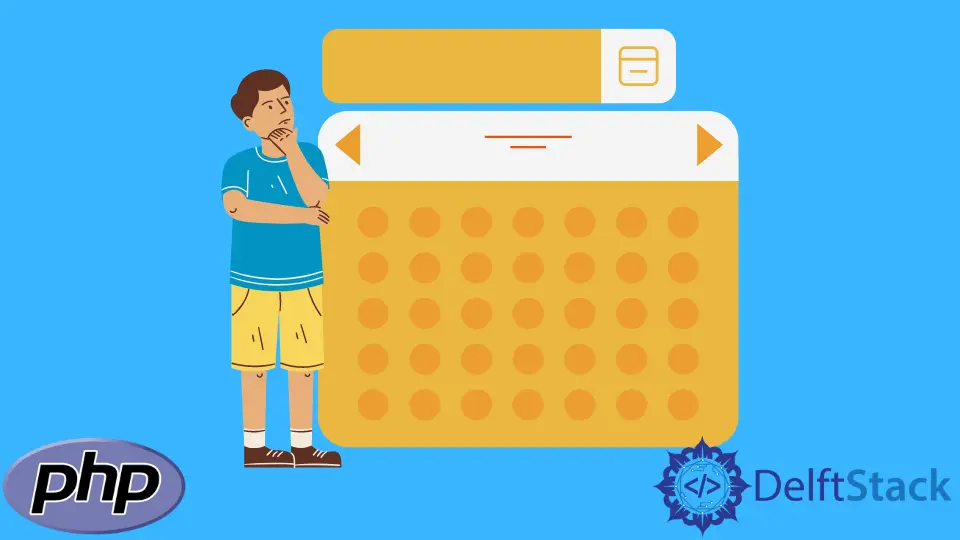
サイト内のフォームに入力する場合、DatePicker を使用して日付を選択する必要がある場合があります。たとえば、生年月日やコース修了日などです。
これを行うには、HTML と JavaScript を使用できます。ただし、日付を使用するには、ユーザーがサーバー側で選択した日付を比較する必要があります。
クライアント側で PHP を使用することはできませんが、サーバー側で DatePicker 値を比較することはできます。この記事では、2つの関数を使用して、クライアント側の DatePicker から取得した値と DatePickers の PHP ライブラリを比較します。
PHP 内で strtotime()
および DateTime()
を使用して日付を作成する
JS DatePicker
を使用すると、ユーザーが選択した日付の値が文字列に格納される可能性が高くなります。strtotime()
関数を使用して、適切に使用できるようにします。
この例では、ユーザーの時刻をベースラインの日付と比較して、ユーザーが 18 歳を超えているかどうかを知ることができます。
コード:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
function above_18($arg) {
$baseline_date = strtotime("2004-03-26");
$users_date = strtotime($arg);
if ($user_date < $baseline_date) {
return "You are below the age of 18.";
} else {
return "You are above the age of 18. Continue with your registration process";
}
}
// Obtain the date of birth of user
// and place as the argument for the above_18() function
$notification = above_18("2007-10-29");
print_r($notification);
?>
</body>
</html>
出力:
You are below the age of 18.
次に、DateTime()
メソッドを使用して同じ結果を達成しましょう。ここで、DatePicker
の値はサーバー側の値とは異なります。
コード:
<html>
<head>
<title>PHP Test</title>
</head>
<body>
<?php
function above_18($arg) {
$baseline_date = new DateTime("2004-03-26");
$users_date = new DateTime($arg);
if ($user_date < $baseline_date) {
return "You are below the age of 18.";
} else {
return "You are above the age of 18. Continue with your registration process";
}
}
// Obtain the date of birth of user
// and place as the argument for the above_18() function
$notification = above_18("07-10-29");
print_r($notification);
?>
</body>
</html>
出力:
You are below the age of 18.
Essential JS for PHP を使って DatePicker
機能を利用する
JS DatePicker
値を比較するだけでなく、Essential JS for PHPというサードパーティライブラリを使用して、PHP DatePicker
を作成できます。
次の例では、EJ
名前空間から PHP ラッパークラスを呼び出して PHP DatePicker
コントロールを作成し、minDate
メソッドと maxDate
メソッドを使用して最小日付と最大日付を設定します。
コード:
<?php
$date = new EJ\DatePicker("datePicker");
echo $date->value(new DateTime())->
minDate(new DateTime("11/1/2016"))->
maxDate(new DateTime("11/24/2016"))->
render();
?>
出力:
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn