完全な NumPy 配列を出力する
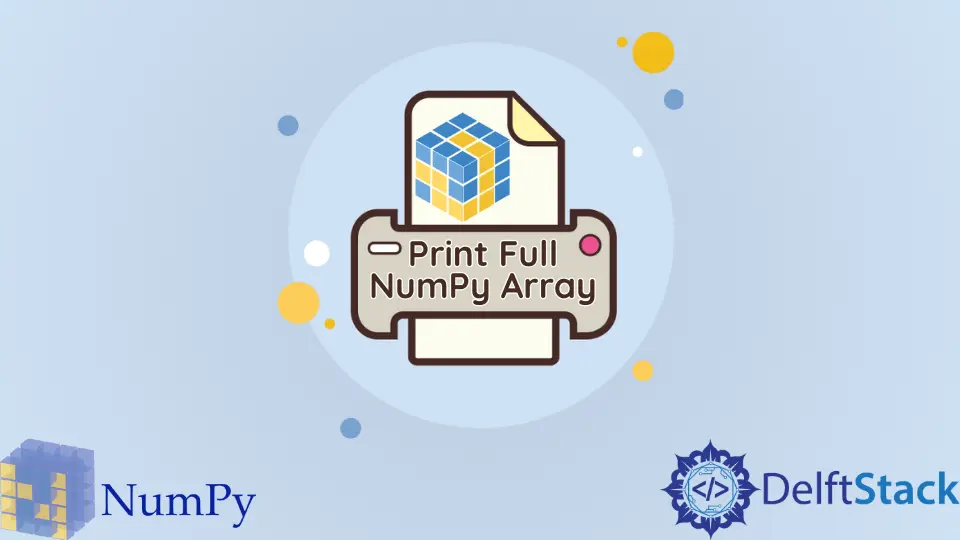
このチュートリアルでは、Python で完全な NumPy 配列を出力する方法を紹介します。
Python で numpy.set_printoptions()
関数を使用して完全な NumPy 配列を出力する
デフォルトでは、配列の長さが大きい場合、Python は配列が出力されるときに出力を切り捨てます。この現象は、以下のコード例で示されています。
import numpy as np
array = np.arange(10000)
print(array)
出力:
[ 0 1 2 ... 9997 9998 9999]
上記のコードでは、Python の np.arange()
関数を使用して、0 から 9999 までの数値を含む NumPy 配列 array
を最初に作成しました。次に、print()
関数を使用して配列の要素を出力しました。配列が大きすぎて完全に表示できないため、出力が切り捨てられます。
この問題は、numpy.set_printoptions()
関数を使用して解決できます。Python の出力配列に関連するさまざまなパラメーターを設定します。numpy.set_printoptions()
関数の threshold
パラメーターを sys.maxsize
に使用して、完全な NumPy 配列を出力できます。sys.maxsize
プロパティを使用するには、sys
ライブラリもインポートする必要があります。次のコード例は、Python で numpy.set_printoptions()
関数と sys.maxsize
プロパティを使用して完全な NumPy 配列を出力する方法を示しています。
import sys
import numpy as np
array = np.arange(10001)
np.set_printoptions(threshold=sys.maxsize)
print(array)
出力:
[ 0 1 2 3 4 5 6 7 8 9 10 11
12 13 14 15 16 17 18 19 20 21 22 23
24 25 26 27 28 29 30 31 32 33 34 35
36 37 38 39 40 41 42 43 44 45 46 47
48 49 50 51 52 53 54 55 56 57 58 59
60 61 62 63 64 65 66 67 68 69 70 71
72 73 74 75 76 77 78 79 80 81 82 83
84 85 86 87 88 89 90 91 92 93 94 95
96 97 98 99 100 101 102 103 104 105 106 107
108 109 110 111 112 113 114 115 116 117 118 119
...
9912 9913 9914 9915 9916 9917 9918 9919 9920 9921 9922 9923
9924 9925 9926 9927 9928 9929 9930 9931 9932 9933 9934 9935
9936 9937 9938 9939 9940 9941 9942 9943 9944 9945 9946 9947
9948 9949 9950 9951 9952 9953 9954 9955 9956 9957 9958 9959
9960 9961 9962 9963 9964 9965 9966 9967 9968 9969 9970 9971
9972 9973 9974 9975 9976 9977 9978 9979 9980 9981 9982 9983
9984 9985 9986 9987 9988 9989 9990 9991 9992 9993 9994 9995
9996 9997 9998 9999 10000]
上記のコードでは、最初に、numpy.arange()
関数を使用して 0 から 10000 までの要素を含む NumPy 配列 array
を作成しました。np.set_printoptions(threshold = sys.maxsize)
関数を使用して、配列の出力オプションを最大に設定します。次に、Python の単純な print()
関数を使用して配列全体を出力しました。
NumPy
ライブラリの使用のみを含む問題に対する別の解決策があります。numpy.set_printoptions()
関数内の threshold
を np.inf
と等しくなるように指定して、Python で完全な配列を出力できます。np.inf
プロパティは、配列全体が出力されるまで print()
が無限に実行されることを指定します。次のコード例を参照してください。
import numpy as np
array = np.arange(10001)
np.set_printoptions(threshold=np.inf)
print(array)
出力:
[ 0 1 2 3 4 5 6 7 8 9 10 11
12 13 14 15 16 17 18 19 20 21 22 23
24 25 26 27 28 29 30 31 32 33 34 35
36 37 38 39 40 41 42 43 44 45 46 47
48 49 50 51 52 53 54 55 56 57 58 59
60 61 62 63 64 65 66 67 68 69 70 71
72 73 74 75 76 77 78 79 80 81 82 83
84 85 86 87 88 89 90 91 92 93 94 95
96 97 98 99 100 101 102 103 104 105 106 107
108 109 110 111 112 113 114 115 116 117 118 119
...
9912 9913 9914 9915 9916 9917 9918 9919 9920 9921 9922 9923
9924 9925 9926 9927 9928 9929 9930 9931 9932 9933 9934 9935
9936 9937 9938 9939 9940 9941 9942 9943 9944 9945 9946 9947
9948 9949 9950 9951 9952 9953 9954 9955 9956 9957 9958 9959
9960 9961 9962 9963 9964 9965 9966 9967 9968 9969 9970 9971
9972 9973 9974 9975 9976 9977 9978 9979 9980 9981 9982 9983
9984 9985 9986 9987 9988 9989 9990 9991 9992 9993 9994 9995
9996 9997 9998 9999 10000]
np.set_printoptions()
関数を使用して、threshold
パラメーターを np.inf
に設定します。次に、Python の単純な print()
関数を使用して配列全体を出力しました。このアプローチは NumPy
ライブラリのみを必要とするため、以前の方法よりもこのアプローチが推奨されます。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn