MySQL での完全結合
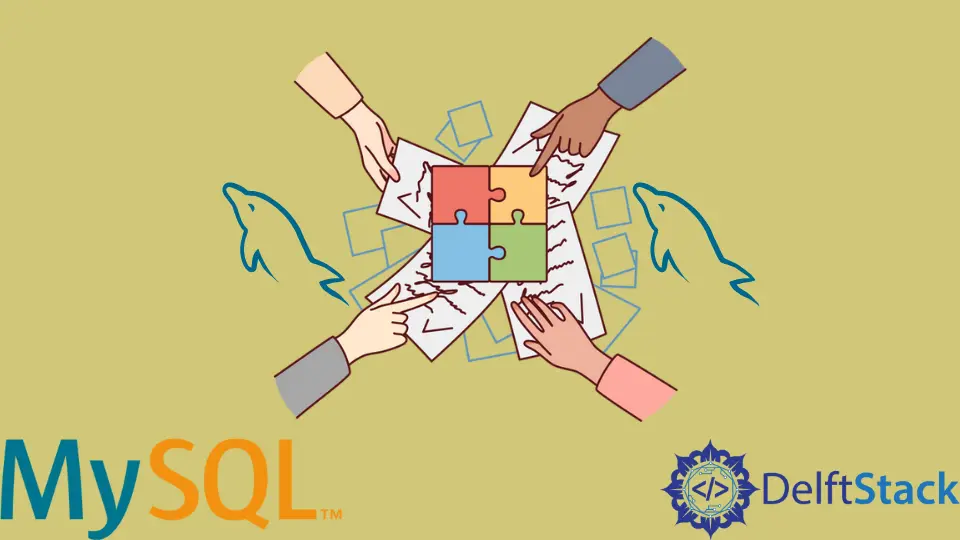
このチュートリアルは、MySQL で完全結合または完全外部結合を実行する方法を探ることを目的としています。
完全外部結合は、2つの別々のテーブルからのデータ全体をマージまたは結合するために使用されます。たとえば、student_id
と student_name
という名前の 2つのテーブルがあり、共通の列があるとします。
共通列の値を UNION
、LEFT JOIN
、および RIGHT JOIN
と照合することにより、これら 2つのテーブルを MySQL で完全にマージできます。このプロセスは一見複雑に見えますが、段階的に理解していきましょう。
始める前に、数行の student_details
テーブルを作成してダミーのデータセットを作成します。
-- create the table student_details
CREATE TABLE student_details(
stu_id int,
stu_firstName varchar(255) DEFAULT NULL,
stu_lastName varchar(255) DEFAULT NULL,
primary key(stu_id)
);
-- insert rows to the table student_details
INSERT INTO student_details(stu_id,stu_firstName,stu_lastName)
VALUES(1,"Preet","Sanghavi"),
(2,"Rich","John"),
(3,"Veron","Brow"),
(4,"Geo","Jos"),
(5,"Hash","Shah"),
(6,"Sachin","Parker"),
(7,"David","Miller");
データのエントリを表示するには、次のコードを使用します。
SELECT * FROM student_details;
出力:
stu_id stu_firstName stu_lastName
1 Preet Sanghavi
2 Rich John
3 Veron Brow
4 Geo Jos
5 Hash Shah
6 Sachin Parker
7 David Miller
stu_id
に対応する各学生のマークを含む student_marks
という名前の別のテーブルが必要です。次のクエリを使用して、このようなテーブルを作成できます。
-- create the table student_marks
CREATE TABLE student_marks(
stu_id int,
stu_marks int
);
-- insert rows to the table student_marks
INSERT INTO student_marks(stu_id,stu_marks)
VALUES(1,10),
(2,20),
(3,30),
(4,7),
(5,9),
(6,35),
(7,15);
次のクエリを使用して、このテーブルを視覚化できます。
SELECT * from student_marks;
出力:
stu_id stu_marks
1 10
2 20
3 30
4 7
5 9
6 35
7 15
両方のテーブルの共通列として stu_id
を使用して、student_details
テーブルと student_marks
テーブルで完全外部結合を作成することを目指しましょう。
MySQL の FULL JOIN
ステートメント
FULL OUTER JOIN
手法の基本的な構文は次のとおりです。
SELECT * FROM table_1
LEFT JOIN table_2 ON table_1.id = table_2.id
UNION
SELECT * FROM table_1
RIGHT JOIN table_2 ON table_1.id = table_2.id
上記のクエリに見られるように、左結合
と右結合
を使用して、共通の id
列に基づいて table_1
と table_2
という名前の 2つのテーブルを結合することを目指しています。
次のクエリを使用して、student_details
テーブルと student_marks
テーブルの問題を解決できます。
-- Full Join using UNION
SELECT * FROM student_details
LEFT JOIN student_marks ON student_details.stu_id = student_marks.stu_id
UNION
SELECT * FROM student_details
RIGHT JOIN student_marks ON student_details.stu_id = student_marks.stu_id
上記のコードに見られるように、stu_id
列に基づいて検討中の両方のテーブルをマージしています。上記のコードの出力は次のとおりです。
stu_id stu_firstName stu_lastName stu_id stu_marks
1 Preet Sanghavi 1 10
2 Rich John 2 20
3 Veron Brow 3 30
4 Geo Jos 4 7
5 Hash Shah 5 9
6 Sachin Parker 6 35
7 David Miller 7 15
出力ブロックに示されているように、完全外部結合後の stu_id
に基づいて、stu_marks
が各学生に正しく割り当てられます。
左結合
と右結合
を使用して結合する正確な列名を指定することにより、列 stu_id
の重複を回避できます。これにより、クエリの UNION
句が原因で、重複行のない外部結合が作成されます。したがって、2つの異なるテーブルでの左結合
と右結合
とともに UNION
ステートメントを使用すると、重複する行がなくても、MySQL で完全外部結合を効率的に作成できます。