Java による MongoDB ドキュメントの一括更新
- 前提条件
- Java を使用した MongoDB のドキュメントの一括更新
-
updateMany()
を使用して、既存のドキュメントに新しいフィールドを追加して一括更新を実行する -
updateMany()
を使用して、フィルターに一致する複数のドキュメントの既存のフィールドの一括更新を実行する -
updateMany()
を使用して、フィルターに一致する埋め込みドキュメントの一括更新を実行する -
bulkWrite()
API を使用して、フィルタークエリに一致する既存のドキュメントを更新する
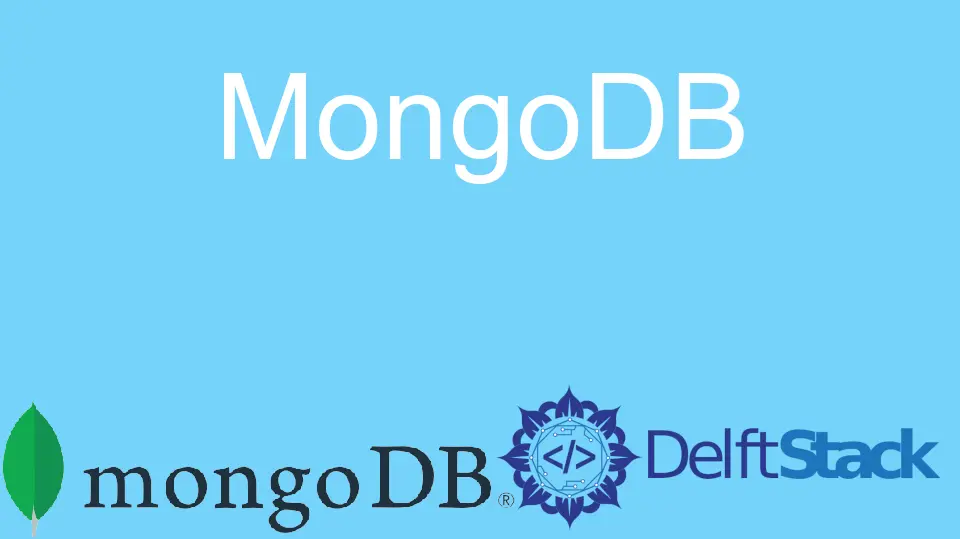
このチュートリアルは、Java ドライバーを使用して MongoDB でドキュメントの一括更新を実行する方法について説明しています。また、新しいフィールドを追加したり、既存のフィールドまたは配列の値を変更したり、ドキュメントを削除したりして更新する方法についても学習します。
前提条件
このチュートリアルでは、従う必要のある次のツールを使用しています。
- Java(Java 18.0.1.1 を使用しています)
- MongoDB サーバー(MongoDB 5.0.8 を使用しています)
- コードエディタまたは任意の JavaIDE(Apache NetBeans IDE 13 を使用しています)
- Maven または Gradle を使用した MongoJava ドライバーの依存関係。Maven で使用しています。
Java を使用した MongoDB のドキュメントの一括更新
一括更新は、データベース内の複数のドキュメントを更新する必要がある場合に役立ちます。さて、問題は、ドキュメントをどのように更新したいかということです。
新しいフィールドを追加したり、既存のフィールドまたは配列の値を更新したり、ドキュメントを削除したりして更新しますか?これらすべてのシナリオを学習するために、コレクションを作成して 2つのドキュメントを挿入しましょう。
コレクションの作成:
db.createCollection('supervisor');
最初のドキュメントを挿入:
db.supervisor.insertOne(
{
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact" : {
"email":"mehvishofficial@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123456789"
},
{
"type" : "personal",
"number" : "124578369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 1,3,4,5,6,7 ] },
{ "_id" : 2, "itemperday" : [ 2,3,4,5,2,7 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,4,0,1 ] }
]
}
);
2 番目のドキュメントを挿入:
db.supervisor.insertOne(
{
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact" : {
"email":"tahirraza@gmail.com",
"phone" : [
{
"type" : "official",
"number" : "123478789"
},
{
"type" : "personal",
"number" : "122378369"
},
]
},
"entries" : [
{ "_id" : 1, "itemsperday" : [ 4,5,6,7,4,6 ] },
{ "_id" : 2, "itemperday" : [ 2,3,2,7,5,2 ] },
{ "_id" : 3, "itemperday" : [ 5,0,0,1,0,0 ] }
]
}
);
コレクションにデータを入力したら、db.supervisor.find().pretty();
を使用できます。挿入されたドキュメントを確認します。簡単な解決策から始めて、少しトリッキーですが興味深い解決策に移ります。
updateMany()
を使用して、既存のドキュメントに新しいフィールドを追加して一括更新を実行する
サンプルコード:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.BasicDBObject;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_1
public class Example_1 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update documents by adding a new field and its value
collection.updateMany(
new BasicDBObject(), new BasicDBObject("$set", new BasicDBObject("status", "Good")));
// print a new line
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_1
出力:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "Female",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "Male",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
出力をフォーマットしました(上記)が、IDE では 1 行に 1つのドキュメントが表示されます。db.supervisor.find().pretty();
を使用することもできますそこに更新されたドキュメントを表示するには、mongo シェルで。
このサンプルコードでは、filter
クエリと update
ステートメントを使用して一致するドキュメントを更新する mongo コレクションオブジェクトの updateMany()
メソッドを使用します。ここでは、ドキュメントをフィルタリングするのではなく、既存のすべてのドキュメントに status
という名前の新しいフィールドを追加しています(上記の出力を参照)。
updateMany()
を使用して、フィルターに一致する複数のドキュメントの既存のフィールドの一括更新を実行する
サンプルコード:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import static com.mongodb.client.model.Filters.eq;
import static com.mongodb.client.model.Updates.combine;
import static com.mongodb.client.model.Updates.set;
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import org.bson.Document;
// Example_2
public class Example_2 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// update the filtered documents by updating the existing field
collection.updateMany(eq("gender", "Female"), combine(set("gender", "F")));
collection.updateMany(eq("gender", "Male"), combine(set("gender", "M")));
System.out.println();
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_2
出力:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "official", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "official", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
このコード例は前のコード例と似ていますが、フィルターに一致するすべてのドキュメントの特定のフィールドの値を更新しています。
updateMany()
を使用して、gender
が Female
に等しく、gender
が Male
に等しいすべてのドキュメントの一括更新を実行しています。 "gender": "Female"
と"gender": "Male"
をそれぞれ"gender":"F"
と"gender":"M"
に変更します。
これまで、updateMany()
メソッドを使用して新しいフィールドを追加したり、最初のレベルで既存のフィールドの値を更新したりする方法を見てきました。次に、updateMany()
を使用して、フィルタークエリを満たす埋め込みドキュメントを一括更新する方法を学習します。
updateMany()
を使用して、フィルターに一致する埋め込みドキュメントの一括更新を実行する
サンプルコード:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.UpdateOptions;
import org.bson.Document;
import org.bson.conversions.Bson;
// Example_3
public class Example_3 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// filter
Bson where = new Document().append("contact.phone.type", "official");
// update
Bson update = new Document().append("contact.phone.$.type", "assistant");
// set
Bson set = new Document().append("$set", update);
// update collection
collection.updateMany(where, set, new UpdateOptions());
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
// print error if unable to execute a command
catch (MongoException error) {
System.err.println("An error occurred while running a command: " + error);
} // end catch block
} // end main method
} // end Example_3
出力:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{ "type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Good"
}
{
"_id": {"$oid": "62a8670192fd89ad9c4932ee"},
"firstname": "Tahir",
"lastname": "Raza",
"gender": "M",
"contact": {
"email": "tahirraza@gmail.com",
"phone": [
{"type": "assistant", "number": "123478789"},
{"type": "personal", "number": "122378369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [4.0, 5.0, 6.0, 7.0, 4.0, 6.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 2.0, 7.0, 5.0, 2.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 1.0, 0.0, 0.0]}
],
"status": "Good"
}
この Java プログラムでは、updateMany()
メソッドを使用して、フィルタークエリに一致する埋め込みドキュメントの一括更新を実行します。
ここでは、contact.phone.type
の値を official
から assistant
に更新します。ドキュメントの配列にアクセスするには、位置演算子($
)を使用して、フィルタークエリを満たす最初の配列値を更新します。
位置演算子を使用しているときに、更新する配列要素を指定することもできます。更新する最初、すべて、または特定の配列要素を指定できます。
位置演算子を使用して配列要素を指定するには、BSON
オブジェクトをナビゲートするためのプロパティアクセス構文であるドット表記
を使用します。次の方法で位置演算子を使用して、最初、すべて、または指定された配列要素を更新します。
-
位置演算子(
$
)を使用して、フィルタークエリを満たす配列の最初の要素を更新します。一方、位置演算子を使用するには、配列フィールドがフィルタークエリの一部である必要があります。 -
すべての位置演算子(
$[]
)を使用して、配列のすべての項目(要素)を更新します。 -
フィルター処理された位置演算子(
$[<identifier>]
)は、フィルタークエリに一致する配列の要素を更新するために使用されます。ここでは、更新操作に配列フィルターを含めて、更新する必要のある配列要素を指定する必要があります。<identifier>
は、配列フィルターに付ける名前であることを忘れないでください。この値は小文字で始まり、英数字のみを保持する必要があります。
bulkWrite()
API を使用して、フィルタークエリに一致する既存のドキュメントを更新する
サンプルコード:
// write your package where you want to save your source file
package com.voidtesting.javamongobulkupdateexample;
// import necessary libraries
import com.mongodb.MongoException;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.DeleteOneModel;
import com.mongodb.client.model.UpdateManyModel;
import com.mongodb.client.model.WriteModel;
import java.util.ArrayList;
import java.util.List;
import org.bson.Document;
// Example_4
public class Example_4 {
// main method
public static void main(String[] args) {
// Replace the connection string with your own connection string
var uri = "mongodb://localhost:27017";
// try block
try (MongoClient mongoClient = MongoClients.create(uri)) {
// print message if connection is successfully established
System.out.println("Connected successfully to server.");
// get the specified database from the MongoDB server
MongoDatabase database = mongoClient.getDatabase("bulkUpdate");
// get the specified collection from the selected database
MongoCollection<Document> collection = database.getCollection("supervisor");
// create object
List<WriteModel<Document>> writes = new ArrayList<>();
// delete the document matching the filter
writes.add(new DeleteOneModel<>(new Document("firstname", "Tahir")));
// update document matching the filter
writes.add(new UpdateManyModel<>(new Document("status", "Good"), // filter
new Document("$set", new Document("status", "Excellent")) // update
));
// bulk write
collection.bulkWrite(writes);
// iterate over all documents of the selected collection
// to print in Java IDE
collection.find().forEach(doc -> {
System.out.println(doc.toBsonDocument());
System.out.println();
}); // end forEach
} // end try block
catch (MongoException me) {
System.err.println("An error occurred while running a command: " + me);
} // end catch block
} // end main method
} // end Example_4
出力:
{
"_id": {"$oid": "62a866e592fd89ad9c4932ed"},
"firstname": "Mehvish",
"lastname": "Ashiq",
"gender": "F",
"contact": {
"email": "mehvishofficial@gmail.com",
"phone": [
{"type": "assistant", "number": "123456789"},
{"type": "personal", "number": "124578369"}
]
},
"entries": [
{"_id": 1.0, "itemsperday": [1.0, 3.0, 4.0, 5.0, 6.0, 7.0]},
{"_id": 2.0, "itemperday": [2.0, 3.0, 4.0, 5.0, 2.0, 7.0]},
{"_id": 3.0, "itemperday": [5.0, 0.0, 0.0, 4.0, 0.0, 1.0]}
],
"status": "Excellent"
}
この例では、bulkWrite()
API を使って複数の操作(削除と更新)を一度に行なっています。DeleteOneModel()
は、フィルターに一致するドキュメントを削除するために使用されますが、UpdateManyModel()
を使用して、フィルターに一致するすべてのドキュメントの既存のフィールドの値を更新します。