Matplotlib で軸の制限を設定する方法
Suraj Joshi
2023年1月30日
-
Matplotlib で軸の範囲を設定するための
xlim()
およびylim()
-
軸の範囲を設定するための
set_xlim()
およびset_ylim()
メソッド -
Matplotlib で軸の範囲を設定するための
axis()
メソッド
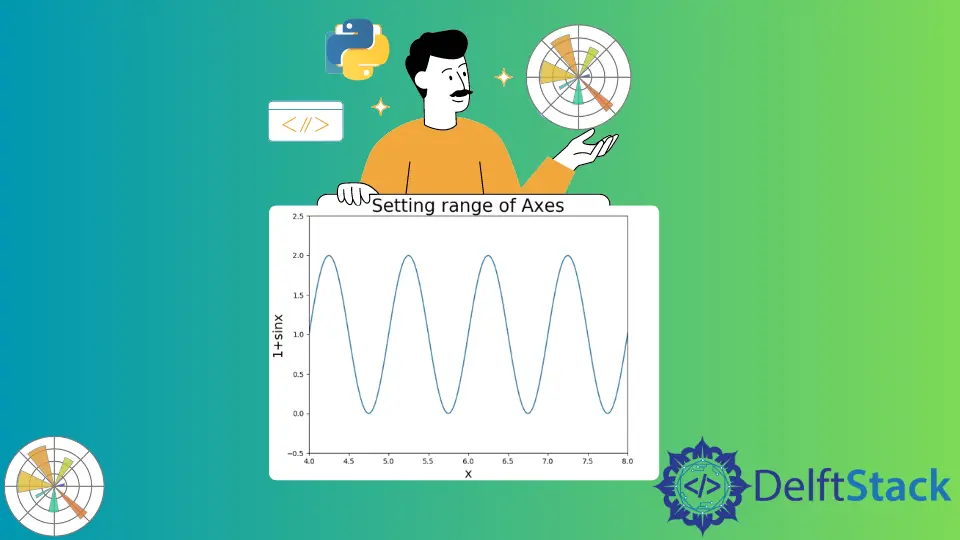
X 軸のみの制限を設定するには、xlim()
メソッドと set_xlim()
メソッドを使用できます。同様に、Y 軸の範囲を設定するために、ylim()
および set_ylim()
メソッドを使用できます。両方の軸の範囲を制御できる axis()
メソッドを使用することもできます。
Matplotlib で軸の範囲を設定するための xlim()
および ylim()
matplotlib.pyplot.xlim()
および matplotlib.pyplot.ylim()
を使用して、X 軸と Y 軸の制限をそれぞれ設定または取得できます。これらのメソッドで引数を渡すと、それぞれの軸に制限が設定され、引数を渡さないと、それぞれの軸の範囲が取得されます。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 500)
y = np.sin(2 * np.pi * x) + 1
fig = plt.figure(figsize=(8, 6))
plt.plot(x, y)
plt.title("Setting range of Axes", fontsize=25)
plt.xlabel("x", fontsize=18)
plt.ylabel("1+sinx", fontsize=18)
plt.xlim(4, 8)
plt.ylim(-0.5, 2.5)
plt.show()
出力:
これにより、X 軸の範囲が 4
から 8
に設定され、Y 軸の範囲が -0.5
から 2.5
に設定されます。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(1, 10, 500)
y = np.sin(2 * np.pi * x) + 1
fig = plt.figure(figsize=(8, 6))
plt.plot(x, y)
plt.title("Plot without limiting axes", fontsize=25)
plt.xlabel("x", fontsize=18)
plt.ylabel("1+sinx", fontsize=18)
plt.show()
出力:
出力の図に見られるように、xlim()
関数と ylim()
関数を使用しなかった場合、X 軸が 0 から 10 の範囲である全範囲の軸でプロットが得られます。Y 軸の範囲は 0
から 2
です。
軸の範囲を設定するための set_xlim()
および set_ylim()
メソッド
matplotlib.axes.Axes.set_xlim
および matplotlib.axes.Axes.set_ylim
は、結果のプロットに表示される数値の範囲の制限を設定するためにも使用されます。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 500)
y = np.sin(2 * np.pi * x) + 1
fig = plt.figure(figsize=(8, 6))
axes = plt.axes()
axes.set_xlim([4, 8])
axes.set_ylim([-0.5, 2.5])
plt.plot(x, y)
plt.title("Setting range of Axes", fontsize=25)
plt.xlabel("x", fontsize=18)
plt.ylabel("1+sinx", fontsize=18)
plt.show()
出力:
Matplotlib で軸の範囲を設定するための axis()
メソッド
matplotlib.pyplot.axis()
を使用して軸の範囲を設定することもできます。構文は次のとおりです。
plt.axis([xmin, xmax, ymin, ymax])
この方法により、X 軸と Y 軸を制御するための個別の機能が不要になります。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 50)
y = np.sin(2 * np.pi * x) + 1
fig = plt.figure(figsize=(8, 6))
plt.axis([4, 9, -0.5, 2.5])
plt.plot(x, y)
plt.title("Setting range of Axes", fontsize=25)
plt.xlabel("x", fontsize=18)
plt.ylabel("1+sinx", fontsize=18)
plt.show()
出力:
著者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn