Bash での while ループの使い方法
-
構文:
while
ループインバッシュ -
例:Bash の
while
ループ -
例:Bash での無限ループ
while
の実行 -
例:
break
ステートメントを使った Bash のwhile
ループ -
例:
continue
文を使った Bash のwhile
ループ
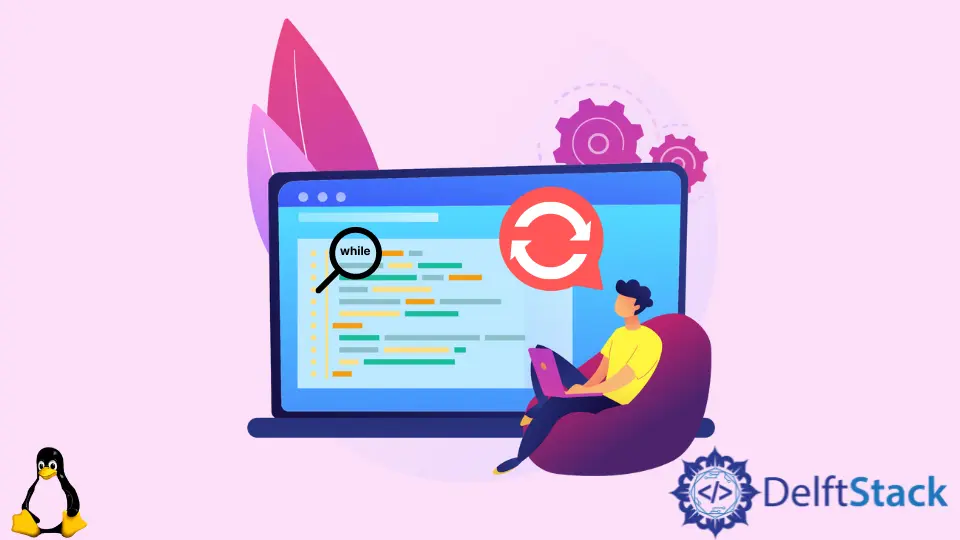
while
ループは、ほとんどすべてのプログラミング言語で最も広く使われているループ構造の一つです。ループを何回実行する必要があるかわからない場合に使われます。while
ループに条件を指定すると、その条件が偽になるまでループ内の文が実行されます。
構文: while
ループインバッシュ
while [condition]
do
command-1
command-2
...
...
command-n
done
ここで、condition
はループ内のコマンドを実行する前に毎回チェックしなければならない条件です。condition
が真であれば、ループ内のコマンドを実行します。condition
が偽ならばループを抜ける。command-1
から command-n
までの文は、condition
が偽になるまでループ内で実行される文です。
例:Bash の while
ループ
#!/bin/bash
num=5
while [ $num -ge 0 ]
do
echo $num
((num--))
done
出力:
5
4
3
2
1
0
ここでは、最初は num
は 5 に設定されています。ターミナルに num
を表示し、num
の値が 0 以上である限りループで num
を 1 減らし続きます。
例:Bash での無限ループ while
の実行
#!/bin/bash
while true
do
echo "This is an infinite while loop. Press CTRL + C to exit out of the loop."
sleep 0.5
done
出力:
This is an infinite while loop. Press CTRL + C to exit out of the loop.
This is an infinite while loop. Press CTRL + C to exit out of the loop.
This is an infinite while loop. Press CTRL + C to exit out of the loop.
^C
これは無限の while
ループで、This is an infinite while loop. Press <kbd>Ctrl</kbd>+<kbd>C</kbd> to exit out of the loop.
を 0.5 秒ごとに出力します。ループを終了するには、CTRL+C を押します。
例:break
ステートメントを使った Bash の while
ループ
#!/bin/bash
num=5
while [ $num -ge 0 ]
do
echo $num
((num--))
if [[ "$num" == '3' ]]; then
echo "Exit out of loop due to break"
break
fi
done
出力:
5
4
Exit out of loop due to break
上記のプログラムでは num
は 5 で初期化されており、num
が 0 以上であればループが実行されるが、num
が 3 になると break
文があるので、num
の値が 3 になった時点でループを抜ける。
例:continue
文を使った Bash の while
ループ
#!/bin/bash
num=6
while [ $num -ge 1 ]
do
((num--))
if [[ "$num" == '3' ]]; then
echo "Ignore a step due to continue"
continue
fi
echo $num
done
出力:
5
4
Ignore a step due to continue
2
1
0
上のプログラムでは num
は 6 で初期化されているが、ループでは num
を 1 減らしてから最新の値を出力します。ループは num
の値が 1 以上である限り実行されます。num
が 3 になると、num
が 3 のときに continue
文があるので、スクリプトは num
の値を表示しません。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn