Kotlin で例外を処理する
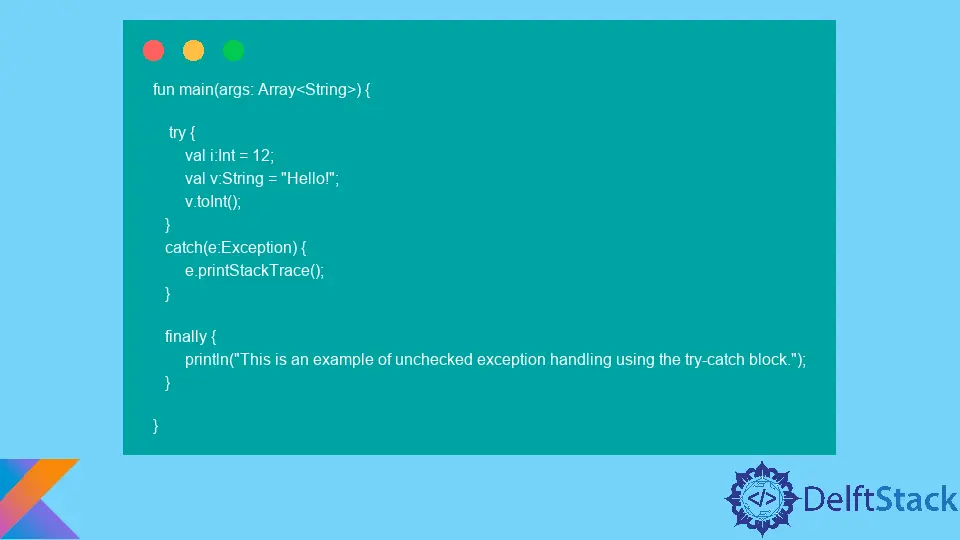
例外処理は、ほとんどのプログラミング言語の基本的な概念です。コードが問題なく実行されるように、例外を処理するのに役立ちます。
Kotlin でさえ、@Throws
アノテーションを使用した例外処理を許可しています。この記事では、@Throws
アノテーションを使用して Kotlin の例外を処理する方法について説明します。
しかし、その前に、Kotlin の例外の基本的な概念を見てみましょう。
コトリンの例外の概念
Kotlin の例外は Java の例外に似ています。それらはすべて、Kotlin の Throwable
クラスの子孫です。ただし、いくつかの違いもあります。
Java とは異なり、Kotlin にはチェックされた例外はありません。したがって、Kotlin にはチェックされていない例外または実行時の例外のみがあります。
また、Kotlin ではカスタム例外を作成できます。したがって、ランタイムエラーを防ぐために、独自の例外処理コードを作成できます。
Kotlin でチェックされていない例外を処理する
前述のように、チェックされていない例外は、実行時に発生する例外です。ArithmeticException
、NullPointerException
、NumberFormatException
などを含むすべての Java のチェックされていない例外は、Kotlin のチェックされていない例外の例です。
try-catch
ブロックと finally
キーワードを使用して、Kotlin でチェックされていない例外を処理できます。
これは、チェックされていない例外を示すためのサンプルコードです。
fun main(args: Array<String>) {
try {
val i:Int = 12;
val v:String = "Hello!";
v.toInt();
}
catch(e:Exception) {
e.printStackTrace();
}
finally {
println("This is an example of unchecked exception handling using the try-catch block.");
}
}
出力:
サンプルコードのデモを確認するには、ここをクリックをクリックしてください。
Kotlin でカスタム例外を作成する
throw
キーワードを使用して、Kotlin でカスタム例外を作成することもできます。整数変数を宣言するコードを作成しましょう。
次に、その数が 18 以上であるかどうかを確認します。もしそうなら、"You are eligible to vote."
というメッセージを表示し、そうでなければ、カスタムエラーメッセージを投げることになります。
fun main(args: Array<String>) {
val v:Int;
v = 16;
if(v >= 18)
{
println("Welcome!! You are eligible to vote.")
}
else
{
//throwing custom exception using the throw keyword
throw customExceptionExample("Sorry! You have to wait to cast a vote.")
}
}
//custom exception class
class customExceptionExample(message: String) : Exception(message)
出力:
サンプルコードのデモを確認するには、ここをクリックをクリックしてください。
Kotlin で@Throws
注釈を使用して例外を処理する
チェックされた例外はありませんが、Kotlin で処理できます。Kotlin @Throws
例外アノテーションを使用してこれを行うことができます。
@Throws
アノテーションは、Java の相互運用性に役立ちます。したがって、Java に変換する必要のあるチェック済みの例外コードがある場合は、JVM マシンに@Throws
アノテーションを使用できます。
これは、同じことを示すためのサンプルコードです。
import java.io.*
import kotlin.jvm.Throws
fun main(args: Array<String>) {
val va=0
var res=0
try {
res=va/0 // Since nothing is divisible by 0, it will throw an exception
} catch (excep: Exception) {
// While this is an Airthmetic exception,
// we will throw a NullPointerException using the function call
excep().throwJavaChecked()
}
}
class excep{
@Throws(NullPointerException::class)
fun throwJavaChecked() {
throw NullPointerException()
}
}
出力:
サンプルコードのデモを確認するには、ここをクリックをクリックしてください。
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn