jQuery を使用して CSS プロパティを削除する
-
jQuery
.css()
API を使用して CSS プロパティを削除する -
jQuery
.prop()
API を使用して CSS プロパティを削除する -
jQuery
.removeAttr()
API を使用して CSS プロパティを削除する -
jQuery
.removeClass()
API を使用して CSS プロパティを削除する
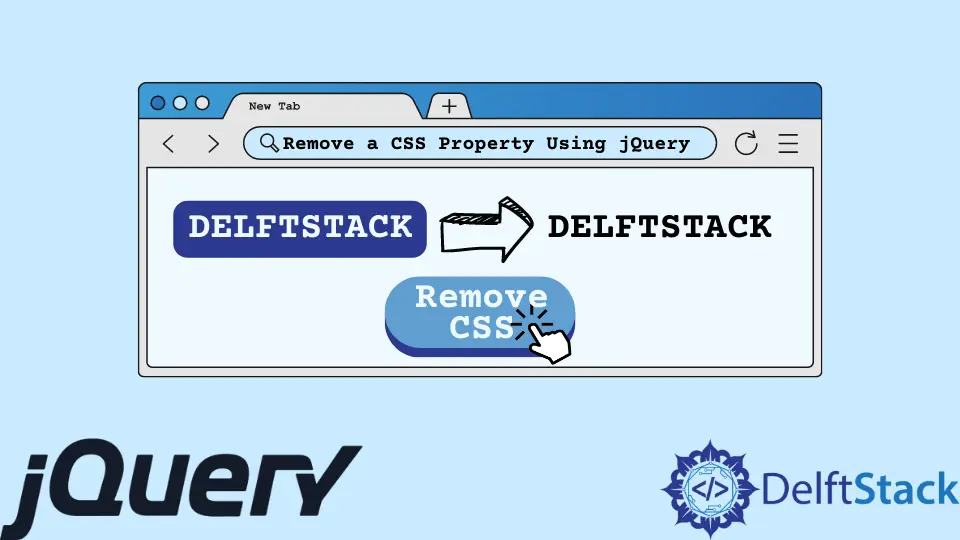
この記事では、jQuery を使用して CSS プロパティを削除する 4つの方法を説明します。 これらのメソッドは、.css()
、.removeAttr()
、.removeClass()
、および .prop()
の 4つの jQuery API を使用します。
jQuery .css()
API を使用して CSS プロパティを削除する
jQuery では、デフォルトで .css()
API を使用して要素に CSS プロパティとその値を設定します。 これから、jQuery はプロパティと値を HTML の style
属性を介してインライン スタイルとして適用します。
ただし、プロパティの値を空の文字列に設定することで、CSS プロパティを削除するためにも使用できます。 つまり、CSS が <style>
タグまたは外部 CSS スタイル シートからのものである場合、このメソッドは機能しません。
次のコードでは、jQuery .css()
API を使用して要素に CSS プロパティを適用しています。 その後、ボタンを押すことができます。 これにより、CSS プロパティを削除する関数が呼び出されます。
関数のコアは、CSS プロパティの値を空の文字列に設定することです。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'font-size': '2em',
'background-color': 'red'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").css({
'font-size': '',
'background-color': ''
});
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
出力:
jQuery .prop()
API を使用して CSS プロパティを削除する
jQuery .css()
API を介して CSS を適用した場合は、jQuery .prop()
API を使用して CSS プロパティを削除できます。 そのような要素には、.prop()
API を使用してアクセスできる style
属性があるためです。
以下では、.prop()
API を使用して CSS プロパティを削除するように前のコードを更新しました。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text 2.0</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'font-size': '3em',
'background-color': '#1560bd'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
let element_with_css_property = $("#test").prop('style');
element_with_css_property.removeProperty('font-size');
element_with_css_property.removeProperty('background-color');
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
出力:
jQuery .removeAttr()
API を使用して CSS プロパティを削除する
.removeAttr()
API は、要素から style
属性を削除することにより、CSS プロパティを削除します。 これは、CSS がインラインである必要があるか、jQuery .css()
API を使用して適用したことを意味します。
このボタンは、style
属性を削除することにより、すべての CSS プロパティを削除します。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<p id="test">Random Text 3.0</p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add two CSS properties
$("#test").css({
'padding': '2em',
'border': '5px solid #00a4ef',
'font-size': '3em'
});
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").removeAttr('style');
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
出力:
jQuery .removeClass()
API を使用して CSS プロパティを削除する
.removeClass()
API は、<style>
タグまたは外部スタイル シートを介して追加された CSS プロパティを削除します。 クラス名を渡すと、それが削除されます。 そうしないと、要素のすべてのクラス名が削除されます。
次の例は、動作中の .removeClass()
を示しています。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-CSS</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
.colorful_big_text {
color: #1560bd;
font-size: 3em;
}
</style>
</head>
<body>
<main>
<p id="test"><i>Random Text 4.0</i></p>
<button id="remove_css">Remove CSS</button>
</main>
<script>
$(document).ready(function(){
// Add a CSS class that contains CSS properties
$("#test").addClass('colorful_big_text');
/*
* Remove the CSS properties.
*/
$("#remove_css").click(function() {
$("#test").removeClass();
// Remove the click event.
$("#remove_css").off('click');
});
});
</script>
</body>
</html>
出力:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn