jQuery のドロップダウンから選択したオプションを取得
-
.val()
メソッドを使用して、ドロップダウンから選択したオプションを取得する -
.find()
メソッドを使用して、ドロップダウンから選択したオプションを取得する -
.filter()
メソッドを使用して、ドロップダウンから選択したオプションを取得する -
:selected
セレクターjQuery 拡張機能を使用して、ドロップダウンから選択されたオプションを取得する -
.text()
メソッドを使用して、選択したオプションテキストを取得する
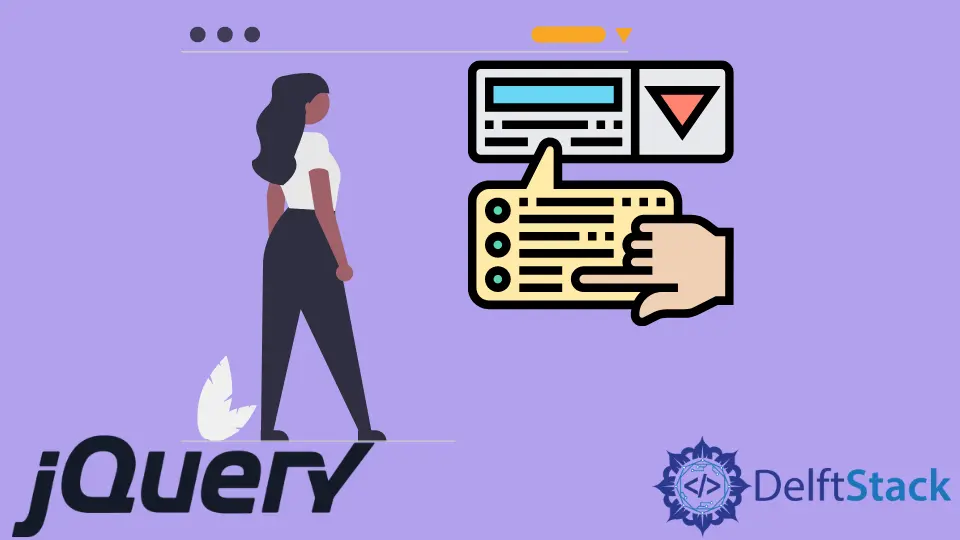
このチュートリアルでは、jQuery を使用してドロップダウンから選択したオプションを取得する方法に取り組みます。 .val()
、.find()
、および .filter()
メソッドを使用します。
また、.text()
を使用して選択したオプションテキストを取得する方法についても説明します。
.val()
メソッドを使用して、ドロップダウンから選択したオプションを取得する
.val()
メソッドを使用して、選択した要素のコレクションの最初の要素の値を取得します。ドロップダウン選択要素に適用すると特殊なケースがあり、この状況では選択されたオプションの値を直接取得します。
jQuery の .val()
メソッドのこの特殊な形式を使用して、選択したオプションを取得します。
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is :
${$("#first-level").val()} <br>`);
});
#first-level
ID 要素は、単一選択の選択ボックスです。コールバックが発生すると、イベントハンドラーをアタッチして、"change"
イベントをリッスンします。
コールバックは、ID が p-first
の <p>
要素に対して .html()
メソッドを実行することにより、新しく選択されたオプションを表示します。
キー実行行は次のコードです。
$("#first-level").val()
.val()
メソッドは、ID first-level
の選択ボックスで実行され、選択されたオプションの値を直接取得します。 .val()
メソッドのもう 1つの特殊なケースでは、複数選択選択ボックスの値による jQuery 選択オプションにその使用を拡張できます。
複数選択の選択ボックスで .val()
メソッドを実行すると、選択したすべてのオプションの値を含む配列が返されます。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
ID multiple
の複数選択選択ボックスの val()
メソッドは、選択されたオプションの値の配列を返します。次に、.map()
メソッドをチェーンしてそれらを反復処理し、ページに表示するテキストとして値を含む <span>
要素の配列を返します。
.val()
メソッド jQuery を使用して、グループ化された選択ボックスから選択されたオプションを取得することもできます。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
ID grouped
のグループ化された選択ボックスには、<optgroup>
タグでオプションが異なるグループに分けられています。 .val()
メソッドを使用すると、選択したオプションを簡単に取得できます。
完全なコード:
//gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
// this directly gets the selected option with the jQuery val() method
//first-level select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").val().map(function(item){
return (`<span> ${item} </span>`)
})}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").val()}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
出力:
jQuery .val()メソッドの詳細についてはこちらをご覧ください
.find()
メソッドを使用して、ドロップダウンから選択したオプションを取得する
要素のコレクションを、.find()
メソッドで選択されたものだけに絞り込むことができます。次に、.val()
メソッドを一般的な形式で適用して、選択したオプション jQuery の値を取得します。
.find()
メソッドは、渡したセレクター引数に一致する、実行する要素のすべての子孫を返します。そのようです:
$("ul .vegetables").find("li .green")
.find()
は、.vegetables
クラス ul
要素の子孫である緑色の li
要素のみを返します。これを使用して、単一選択のドロップダウンから選択したオプションを取得できます。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
:selected
セレクターを使用して、first-level
select
ボックスの option
子孫要素のみを最初に検索します。次に、.val()
メソッドを一般的な形式でチェーンして値を取得します。
この方法を複数選択の選択ボックスに拡張することもできます。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
まず、$("multiple").find(:selected)
コードスニペットを使用して、選択した option
要素のコレクションを見つけます。次に、.map()
メソッドをチェーンしてそれらを繰り返します。
コールバックは、<span>
要素の配列内のコードの $(this).val()
チャンクを使用して、選択されたオプションの値を収集します。最後に、get()
メソッドと join()
メソッドをチェーンして、これらの値を適切なテキストで表示します。
グループ化された選択ボックスにも同じ方法を使用できます。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
完全なコード:
// we first select the particular option using the find method and then find its val indirectly here
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple").find(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped").find(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
出力:
.find()
メソッドの詳細については、こちらをご覧ください
.filter()
メソッドを使用して、ドロップダウンから選択したオプションを取得する
.filter()
メソッドを使用して、選択したオプションのみを収集できます。次に、.val()
メソッドを通常の形式で実行して、選択したオプション jQuery の値を取得できます。
.filter()
メソッドは、子孫を選択せず、呼び出されたコレクションから一致する要素のみを選択するという点で、.find()
メソッドとは異なります。次のスニペットのように、これを使用して jQuery でオプションを値で選択できます。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
$("#first-level option")
セレクターを使用して、first-level
select要素から
option` 要素を明示的に収集します。
.filter()
は、.find()
メソッドのような子孫要素を自動的に取得しないことに注意してください。手動で行う必要があります。
次に、.filter(":selected")
メソッドをチェーンして選択した option
要素を取得し、.val()
メソッドを jQuery getselected オプションにチェーンします。複数選択の選択ボックスについても同じことができます。
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
コードは上記の .find()
ソリューションと似ていますが、.filter()
メソッドをチェーンする前に、 $("#multiple option")
チャンクで option
要素を手動で選択する必要がある点が異なります。同様に、このソリューションをグループ化されたオプションと選択ドロップダウンに使用できます。
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
完全なコード:
// we can get the selected option with indirect use of val() method and the filter() method
// first-select element
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level option").filter(":selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple option").filter(":selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped option").filter(":selected").val()
}`);
})
//reset and restart again button
$("button").on("click",function(){
location.reload();
})
出力:
:selected
セレクターjQuery 拡張機能を使用して、ドロップダウンから選択されたオプションを取得する
.find()
または .filter()
メソッドを使用する代わりに、jQuery :selected
拡張子を使用して、選択したオプションのみを直接選択することもできます。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level :selected").val()} <br>`);
});
//multiple select element
$("#multiple").on("change",function(){
$("#p-multiple").html(`The selected items in the multiple-choice box are :
${$("#multiple :selected").map(function(){
return (`<span> ${$(this).val()} </span>`)
}).get().join()
}`);
});
//grouped select element
$("#grouped").on("change",function(){
$("#p-grouped").html(`The selected items in the grouped box are: ${$("#grouped :selected").val()}`);
})
コードは上記の他のメソッドと同じですが、他のメソッドをチェーンする前に $("first-level :selected")
セレクター式を使用する点が異なります。
出力:
:selected
疑似セレクターは jQuery 拡張機能であり、CSS の一部ではないため、最初に .find()
または .filter()
を使用することをお勧めします。したがって、純粋な CSS セレクターのようにネイティブ DOM メソッドのパフォーマンスが向上するというメリットはありません。
:checked
セレクターは、'select'
要素に適用されると、選択されたオプションを取得します。これは純粋な CSS セレクターであるため、:selected
疑似セレクターよりもパフォーマンスが優れています。
.text()
メソッドを使用して、選択したオプションテキストを取得する
value
の代わりに option
要素内のテキストを検索することをお勧めします。jQuery の .text()
メソッドを使用して、選択したオプションテキストを取得できます。
$("#first-level").on("change",function(){
$("#p-first").html(`<br> The selected item in the first single-choice box is : ${$("#first-level").find(":selected").text()} <br>`);
});
コードの残りの部分は上記の他のメソッドと同じですが、選択したオプションテキストを取得するために .val()
メソッドの代わりに .text()
メソッドを最後にチェーンする点が異なります。
出力: