jQuery: 要素の背景色を変更する
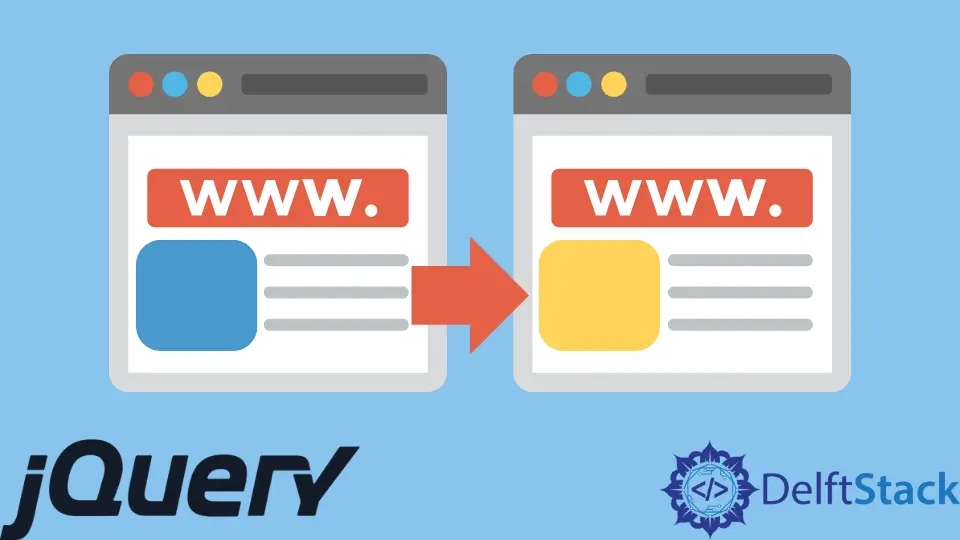
この記事では、jQuery を使用してマウスオーバー時に要素の背景色を変更する方法について説明します。 queue()
と hide()
という 2つの jQuery API を使用してこれを実現します。
jQuery queue()
API を使用して背景色を変更する
この最初の例は、アニメーションの前後で背景色を変更します。 ここでは、css()
API だけでは意図したとおりに機能しないため、queue()
API が必要になります。
これは、アタッチされた要素に即座に影響を与えるためです。 queue()
API を使用すると、css()
API の結果の後にエフェクトを起動する関数を渡すことができます。
これは、css()
の初期色がアニメーションの開始時に影響を与えることを意味します。 その後、queue()
API を使用して、初期色を別の色に変更できます。
以下は、プロセスの疑似コードです。
- ボタンの上にマウスを置くと、
css()
API の初期色が有効になります。 - 要素がフェードアウトします。
- 要素が再び表示されます。
- 最後に、その色が
queue()
API で定義された別の色に変わります。
次のコードは、疑似コードの実装です。 次に示すのは、Web ブラウザーでの結果です。
その結果、2 回目にマウスをボタンの上に置いたときにアニメーションが発生しないことがわかります。 これについては、次の例で説明します。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-change-background-color-with-queue-API</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
main { border: 3px solid #1a1a1a; padding: 1.2em; display: grid; }
button { align-self: center; padding: 1.2em; cursor: pointer; }
p { font-size: 5em; }
</style>
</head>
<body>
<main>
<p id="random_big_text">A big random text.</p>
<button>Move your mouse here.</button>
</main>
<script>
$(document).ready(function() {
$("button").mouseover(function() {
let random_big_text = $("#random_big_text");
random_big_text.css("background-color", "#ffff00");
random_big_text.hide(1500).show(1500);
random_big_text.queue(function() {
random_big_text.css("background-color", "#ff0000");
});
});
});
</script>
</body>
</html>
出力:
hide()
API を使用した jQuery の背景色の変更
jQuery hide()
API を使用して背景色を変更する方法は、queue()
API を使用した最初の例に基づいています。 結果は同じですが、2つの違いがあります。 API チェーンを使用しており、その効果は毎回機能します。
その結果、各アニメーションの最後にあるボタンにマウスを合わせると、プロセスが最初からやり直されます。 次のコードは、その方法を示しています。 以下は、Web ブラウザーでの結果です。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-change-background-color-with-hide-API</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
main { border: 3px solid #1a1a1a; padding: 1.2em; display: grid; }
button { align-self: center; padding: 1.2em; cursor: pointer; }
p { font-size: 5em; }
</style>
</head>
<body>
<main>
<p id="random_big_text">A big random text.</p>
<button>Move your mouse here.</button>
</main>
<script>
$(function(){
$("button").mouseover(function(){
let random_big_text = $("#random_big_text");
random_big_text.stop()
.css("background-color","#ffff00")
.hide(1500, function() {
random_big_text.css("background-color","#ff0000")
.show(1500);
});
});
});
</script>
</body>
</html>
出力:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn