JavaScript NodeList
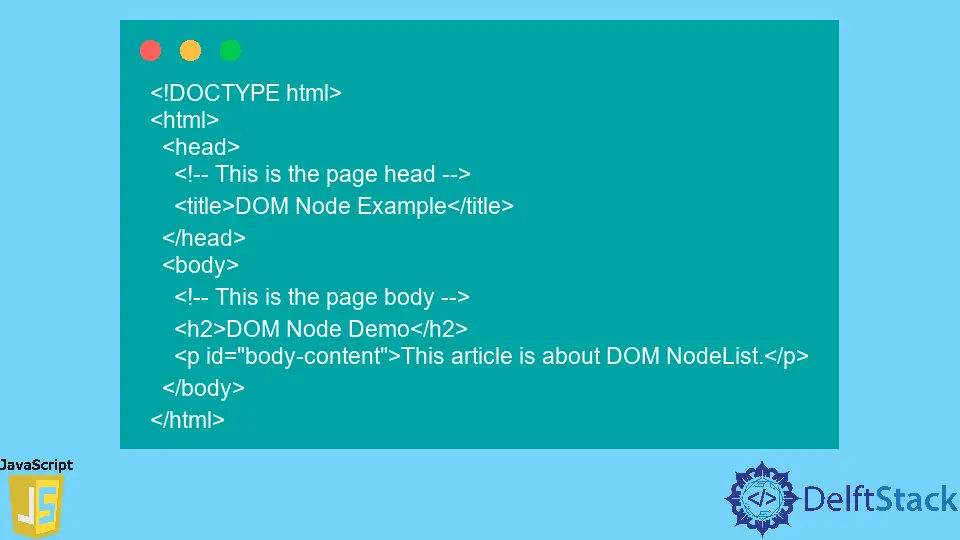
HTML DOM は、HTML ドキュメントのインターフェイスである Document Object Model の略です。 すべての Web ページまたはドキュメントを、ノードのコレクションで構成されるツリー構造として扱います。
簡単に言えば、ノードは Web ドキュメントのオブジェクトです。 これらのノードは、階層的に DOM ドキュメントに配置されます。
さらに、親ノードと子ノードがある場合もあります。
HTML DOM ノードの紹介
次のような HTML ページがあるとします。
<!DOCTYPE html>
<html>
<head>
<!-- This is the page head -->
<title>DOM Node Example</title>
</head>
<body>
<!-- This is the page body -->
<h2>DOM Node Demo</h2>
<p id="body-content">This article is about DOM NodeList.</p>
</body>
</html>
上記の HTML ページは、ブラウザによってツリー構造として解釈されます。 したがって、次のようになります。
|-doctype: html
|-HTML
-|HEAD
-|TITLE
-|text: DOM Node Example
-|BODY
-|comment: This is the page body
-|H2
-|text: DOM Node Demo
-|P id="body-content"
-|text: This article is about DOM NodeList.
各 HTML タグは DOM ノードですが、ノードは HTML タグに限定されません。 HTML テキストやコメントも DOM ノードと見なされます。
したがって、HTML ノードと要素は 2つの異なるものであり、HTML 要素は DOM ノードのサブタイプです。
JavaScript NodeList
オブジェクト
名前が示すように、NodeList
オブジェクトは DOM ノードのコレクションです。 HTMLCollection
オブジェクトに非常に似ていますが、わずかな違いがあります。 HTMLCollection
オブジェクトのようなものは名前と ID でアクセスできますが、NodeList
オブジェクトはインデックスベースのアクセスしかサポートしていません。
さらに、HTMLCollection
には Node.ELEMENT_NODE
タイプのノードのみが含まれます。 NodeList
オブジェクトは、属性ノード、テキスト ノード、要素ノードを含むコメント ノードなど、さまざまなタイプのノードを保持できます。
NodeList
オブジェクトには、ライブと静的の 2 種類があります。これについては、次のセクションで説明します。
静的 vs ライブ NodeList
オブジェクト
document
オブジェクトの childNodes
プロパティは、ライブ NodeList
として呼び出した NodeList
オブジェクトを返します。 このオブジェクトは DOM の変更によって自動的に影響を受けるためです。
以下に示すように、ブラウザ コンソールを使用して、JavaScript を使用して上記の HTML ドキュメントにアクセスしてみましょう。
const bodyContent = document.getElementById('body-content');
var nodeListForBody = bodyContent.childNodes;
console.log(nodeListForBody);
出力:
予想どおり、NodeList
オブジェクトは childNodes
プロパティによって返されました。 nodeListForBody
オブジェクトの長さを確認してみましょう。
console.log(nodeListForBody.length);
出力:
次のように、paragraph 要素に新しい子要素を追加しましょう。
bodyContent.appendChild(document.createElement('div'));
次に、以下に示すように、NodeList
nodeListForBody
の長さを確認します。
console.log(nodeListForBody.length);
出力:
DOM の変更により nodeListForBody
の長さが変更されたため、これはライブの NodeList
オブジェクトです。
ライブの NodeList
オブジェクトとは対照的に、静的な NodeList
は DOM の変更の影響を受けません。 通常、document.querySelectorAll()
メソッドは静的な NodeList
オブジェクトを返します。
NodeList
オブジェクトの操作
NodeList
は配列ではありません。 NodeList
をそのインデックスでループすることができます。これは JavaScript 配列に似ていますが、pop()
、push()
、 join()
などの配列操作はサポートしていません。
上記の NodeList
: nodeListForBody
の最初のノードにインデックス 0 でアクセスしてみましょう。
console.log(nodeListForBody[0]);
出力:
NodeList
は、Array.from()
メソッド を使用して、典型的な JavaScript 配列に簡単に変換できます。
const nodeListAsArray = Array.from(nodeListForBody)
console.log(nodeListAsArray);
出力:
予想通り、上記の NodeList
nodeListForBody
は配列 nodeListAsArray
に変換されました。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.