JavaScript で入力値を取得する
Kirill Ibrahim
2023年10月12日
JavaScript
JavaScript Input
-
JavaScript で
document.getElementById(id_name)
を使用して入力値を取得する -
JavaScript で
document.getElementsByClassName('class_name')
を使って入力値を取得する -
JavaScript で
document.querySelector('selector')
を使用して入力値を取得する
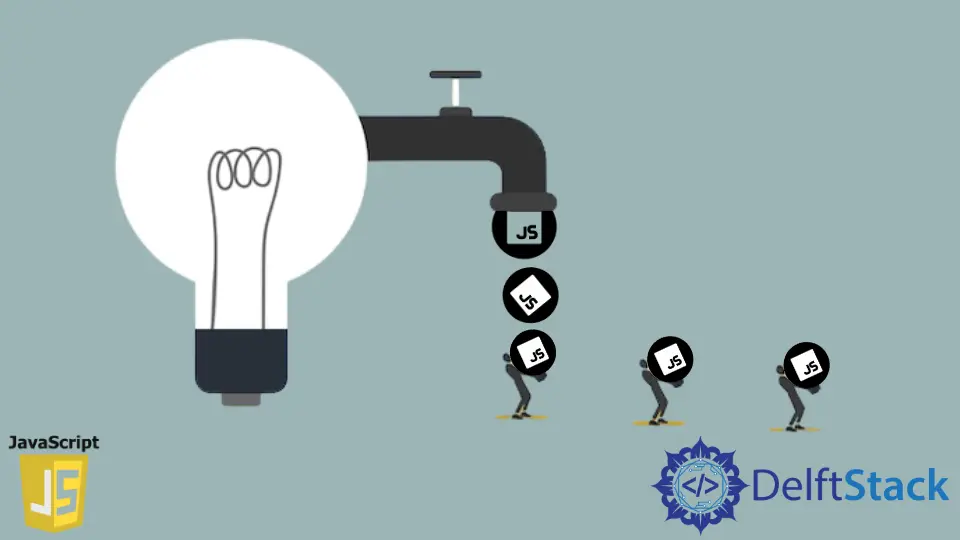
JavaScript では、DOM input 要素を選択して value
プロパティを使用することで、フォーム要素の中にラップさせずに入力の値を取得することができます。
JavaScript には、DOM 入力要素を選択するためのさまざまな方法があります。以下のすべてのメソッドには、マシンで実行できるコード例があります。
JavaScript で document.getElementById(id_name)
を使用して入力値を取得する
Dom 入力要素に id
プロパティを与え、document.getElementById(id_name)
を用いて DOM の入力要素を選択しますが、単に value
プロパティを用いることもできます。
例:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<label for="domTextElement">Name: </label>
<input type="text" id="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementById("domTextElement").value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
JavaScript で document.getElementsByClassName('class_name')
を使って入力値を取得する
DOM 入力要素に class プロパティを与え、document.getElementsByClassName('class_name')
を使用して DOM 入力要素を選択しますが、同じクラス名の異なる DOM 入力要素がある場合は DOM 入力の配列を返しますので、どれを選択するかはインデックス番号を指定して指定する必要があります: document.getElementsByClassName('class_name')[index_number].value
.
例:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" class="domTextElement" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue = document.getElementsByClassName("domTextElement")[1].value;
document.getElementById("valueInput").innerHTML = inputValue;
}
</script>
</body>
</html>
JavaScript で document.querySelector('selector')
を使用して入力値を取得する
document.querySelector('selector')
は CSS
セレクタを使用します。つまり、DOM 要素の id、class、タグ名、name プロパティで要素を選択できるということです。
例:
document.querySelector('class_name')
document.querySelector('id_name')
document.querySelector('input') // tag name
document.querySelector('[name="domTextElement"]') // name property
例:
<!DOCTYPE html>
<html>
<body>
<h4>
Change the text of the text field
,and then click the button.
</h4>
<input type="text" id="domTextElement1" >
<label for="domTextElement">Name: </label>
<input type="text" class="domTextElement2" >
<button type="button" onclick="getValueInput()">
click me!!
</button>
<p id="valueInput"></p>
<script>
const getValueInput = () =>{
let inputValue1 = document.querySelector("#domTextElement1").value;
let inputValue2 = document.querySelector(".domTextElement2").value;
document.querySelector("#valueInput").innerHTML = `First input value: ${inputValue1} Second Input Value: ${inputValue2}`;
}
</script>
</body>
</html>
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe