Java の JavaTuples
Rupam Yadav
2023年10月12日
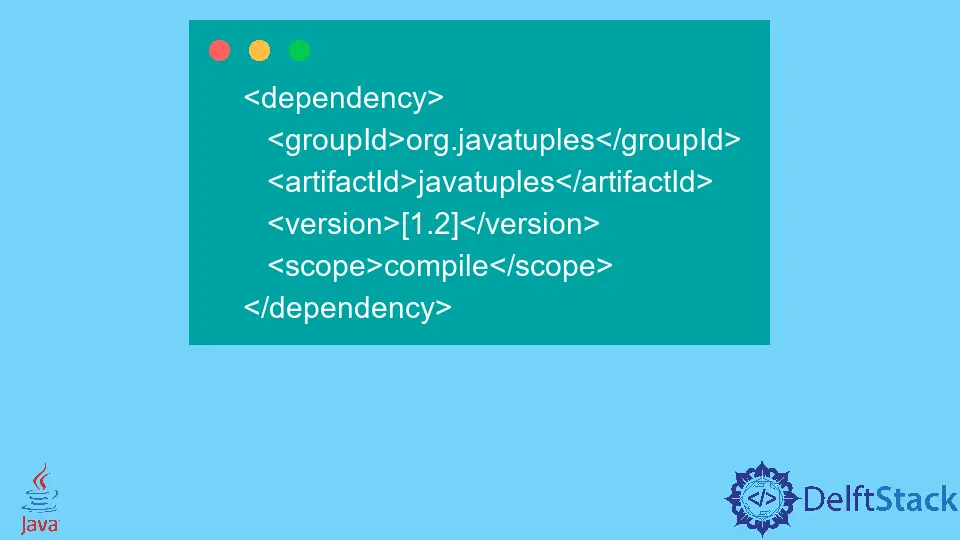
タプルは、オブジェクト間の関係を必要とせずにオブジェクトを格納できるが、相互の動機を持つデータ構造です。Java はタプルデータ構造をサポートしていませんが、JavaTuples
ライブラリを使用できます。
JavaTuples
の機能
- タプルはタイプセーフです
- それらは不変です
- タプル値を反復処理できます
- それらはシリアル化可能です
Comparable<Tuple>
を実装しますequals()
、hashCode()
、toString()
などのメソッドを実装します
JavaTuples
のタプルクラス
タプルクラスでは最大 10 個の要素を使用できます。以下の表に記載されている JavaTuples
の要素の数ごとに特別なサブクラスがあります。
要素数(タプルサイズ) | クラス名 | 例 |
---|---|---|
一 | Unit |
Unit<1> |
二 | Pair |
Pair<1,2> |
三 | Triplet |
Triplet<1,2,3> |
四 | Quartet |
Quartet<1,2,3,4> |
五 | Quintet |
Quintet<1,2,3,4,5> |
六 | Sextet |
Sextet<1,2,3,4,5,6> |
七 | Septet |
Septet<1,2,3,4,5,6,7> |
八 | Octet |
Octet<1,2,3,4,5,6,7,8> |
九 | Ennead |
Ennead<1,2,3,4,5,6,7,8,9> |
十 | Decade |
Decade<1,2,3,4,5,6,7,8,9,10> |
JavaTuples
のメソッド
Tuple
クラスは、操作を実行するためのさまざまなメソッドを提供します。
方法 | 説明 |
---|---|
equals() |
これは、Object クラスの equals メソッドのデフォルトの実装をオーバーライドするために使用されます |
hashCode() |
各オブジェクトのハッシュコードを返します。 |
toString() |
文字列表現を返します |
getSize() |
タプルのサイズを返します |
getValue() |
タプル内の指定された位置の値を返します |
indexOf() |
指定された文字列内の要求されたサブ文字列のインデックスを返します。 |
contains() |
タプルに要素が存在することを確認してください |
containsAll() |
リストまたは配列のすべての要素がタプルに存在するかどうかを確認します |
iterator() |
イテレータを返します |
lastIndexOf() |
指定された文字列内の要求された部分文字列の最後のインデックスを返します。 |
toArray() |
タプルからアレイへ |
toList() |
リストするタプル |
Java でタプルを実装する
JavaTuples
ライブラリを使用するために、次の Maven ベースの依存関係をインポートします。
<dependency>
<groupId>org.javatuples</groupId>
<artifactId>javatuples</artifactId>
<version>[1.2]</version>
<scope>compile</scope>
</dependency>
この例では、上記の表にさまざまなタプルクラスを実装し、それらを出力して値を取得します。String
、Integer
、Boolean
などの異なるデータ型を用意します。
また、クラス DummyClass
を作成し、エラーがスローされた場合はそのオブジェクトをタプルに渡します。
import org.javatuples.*;
public class JavaTuplesExample {
public static void main(String[] args) {
Unit<String> unitTuple = new Unit<>("First Tuple Element");
Pair<String, Integer> pairTuple = new Pair<>("First Tuple Element", 2);
Triplet<String, Integer, Boolean> tripletTuple = new Triplet<>("First Tuple Element", 2, true);
Quartet<String, Integer, Boolean, String> quartetTuple =
new Quartet<>("First Tuple Element", 2, true, "Fourth Tuple Element");
Quintet<String, Integer, Boolean, String, Integer> quintetTuple =
new Quintet<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5);
Sextet<String, Integer, Boolean, String, Integer, DummyClass> sextetTuple =
new Sextet<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5, new DummyClass());
Septet<String, Integer, Boolean, String, Integer, DummyClass, Integer> septetTuple =
new Septet<>(
"First Tuple Element", 2, true, "Fourth Tuple Element", 5, new DummyClass(), 7);
Octet<String, Integer, Boolean, String, Integer, DummyClass, Integer, String> octetTuple =
new Octet<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5, new DummyClass(), 7,
"Eight Tuple Element");
Ennead<String, Integer, Boolean, String, Integer, DummyClass, Integer, String, Integer>
enneadTuple = new Ennead<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5,
new DummyClass(), 7, "Eight Tuple Element", 9);
Decade<String, Integer, Boolean, String, Integer, DummyClass, Integer, String, Integer, String>
decadeTuple = new Decade<>("First Tuple Element", 2, true, "Fourth Tuple Element", 5,
new DummyClass(), 7, "Eight Tuple Element", 9, "Tenth Tuple Element");
System.out.println(unitTuple);
System.out.println(pairTuple);
System.out.println(tripletTuple);
System.out.println(quartetTuple);
System.out.println(quintetTuple);
System.out.println(sextetTuple);
System.out.println(septetTuple);
System.out.println(octetTuple);
System.out.println(enneadTuple);
System.out.println(decadeTuple);
}
}
class DummyClass {}
出力:
[First Tuple Element]
[First Tuple Element, 2]
[First Tuple Element, 2, true]
[First Tuple Element, 2, true, Fourth Tuple Element]
[First Tuple Element, 2, true, Fourth Tuple Element, 5]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@254989ff]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@5d099f62, 7]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@37f8bb67, 7, Eight Tuple Element]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@49c2faae, 7, Eight Tuple Element, 9]
[First Tuple Element, 2, true, Fourth Tuple Element, 5, DummyClass@20ad9418, 7, Eight Tuple Element, 9, Tenth Tuple Element]
著者: Rupam Yadav
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn