Java の静的インターフェース
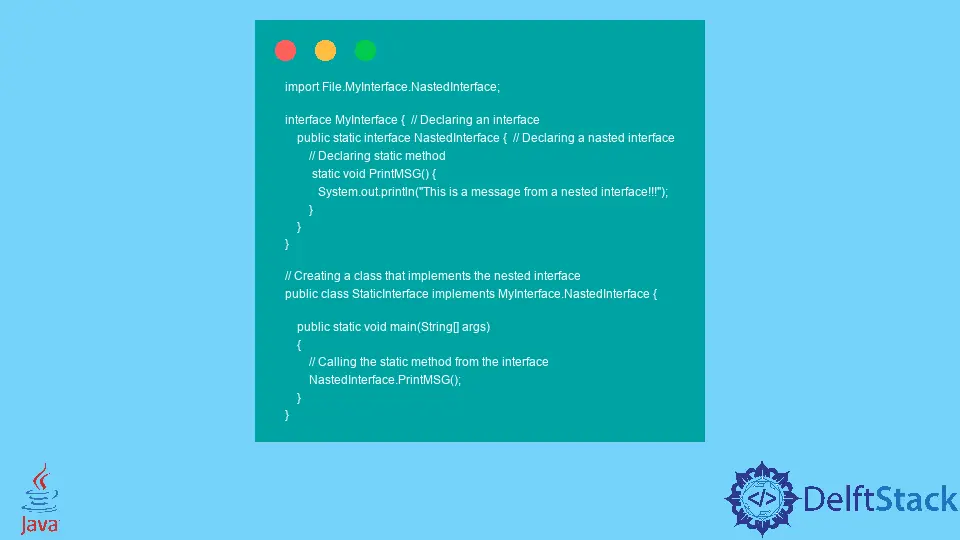
interface
は Java などのオブジェクト指向プログラミング言語で最も一般的に使用される要素であり、キーワード static
は要素を固定するために使用されます。
この記事では、静的インターフェイスを宣言する方法を示します。 また、トピックをより簡単にするために、必要な例と説明を使用してトピックについて説明します。
interface
がネストされているか、別のインターフェイスの子である場合、interface
を static に宣言できることに注意してください。
Java のネストされたインターフェイスで static
を使用する
以下の例では、ネストされた interface
で static
を使用する方法を示しています。 ただし、ネストされた interface
を宣言するときにキーワード static
を使用することはオプションです。これは、キーワード static
を使用しない場合と同じ結果を示すためです。
この例のコードは次のようになります。
import File.MyInterface.NastedInterface;
interface MyInterface { // Declaring an interface
public static interface NastedInterface { // Declaring a nasted interface
// Declaring static method
static void PrintMSG() {
System.out.println("This is a message from a nested interface!!!");
}
}
}
// Creating a class that implements the nested interface
public class StaticInterface implements MyInterface.NastedInterface {
public static void main(String[] args) {
// Calling the static method from the interface
NastedInterface.PrintMSG();
}
}
各行の目的はコメントとして残されています。 ネストされた interface
のキーワード static
はオプションであることに注意してください。
上記の例を実行すると、コンソールに以下の出力が表示されます。
This is a message from a nested interface!!!
Java の interface
内で static
メソッドを使用する
インターフェイス内で各要素 static
を宣言することにより、静的インターフェイスを作成することもできます。 メソッドを static として宣言するときは、interface
内でメソッドを定義する必要があります。
以下の例を見てみましょう。
// Declaring an interface
interface MyInterface {
// A static method
static void PrintMSG() {
System.out.println("This is a message from the interface!!!"); // Method is defined
}
// An abstract method
void OverrideMethod(String str);
}
// Implementation Class
public class StaticInterface implements MyInterface {
public static void main(String[] args) {
StaticInterface DemoInterface = new StaticInterface(); // Creating an interface object
// An static method from the interface is called
MyInterface.PrintMSG();
// An abstract method from interface is called
DemoInterface.OverrideMethod("This is a message from class!!!");
}
// Overriding the abstract method
@Override
public void OverrideMethod(String str) {
System.out.println(str); // Defining the abstract method
}
}
上記の例では、interface
内で static
を使用する方法を示しました。
各行の目的はコメントとして残されています。 上記の例の interface
で、PrintMSG
という静的メソッドと OverrideMethod
という抽象メソッドの 2つのメソッドを宣言しました。
メソッド PrintMSG
はインターフェイス内の静的メソッドであるため、インターフェイスで定義されていることがわかります。
上記の例を実行すると、コンソールに以下の出力が表示されます。
This is a message from the interface!!!
This is a message from class!!!
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn